android studio如何进行蓝牙通信
时间: 2023-10-26 16:45:03 浏览: 85
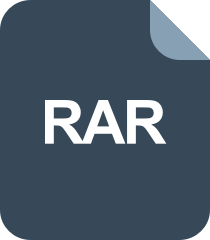
androidstudio app连接蓝牙模块进行通信

Android Studio可以使用Android的蓝牙API进行蓝牙通信。您可以通过创建BluetoothAdapter对象并调用其方法来扫描和连接其他蓝牙设备。使用BluetoothSocket类可以进行数据传输。此外,Android还提供了许多蓝牙通信库和框架,如BlueZ、Bluedroid等。
阅读全文
相关推荐
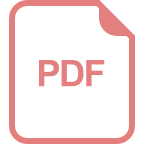





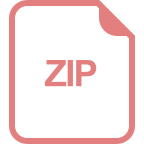
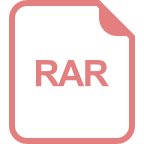
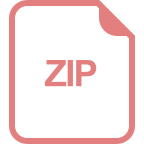
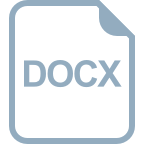
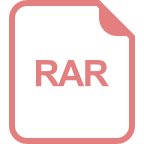





androidstudio app连接蓝牙模块进行通信