请把刚刚的代码用所有语言写一遍并标明所用语言
时间: 2023-10-15 09:02:10 浏览: 87
抱歉,我不支持多语言同时编写代码,但我可以使用多种语言分别实现。以下是使用 C++、Python、Java、JavaScript、Go 语言分别实现的代码:
C++:
```c++
#include<iostream>
using namespace std;
//定义二叉树的节点结构
struct BiTreeNode{
char data;
BiTreeNode *lchild, *rchild;
};
//创建二叉树函数
void CreateBiTree(BiTreeNode *&T){
char ch;
cin>>ch;
if(ch=='#'){
T=NULL;
}
else{
T=new BiTreeNode;
T->data=ch;
CreateBiTree(T->lchild);
CreateBiTree(T->rchild);
}
}
//先序遍历
void PreOrder(BiTreeNode *T){
if(T!=NULL){
cout<<T->data<<" ";
PreOrder(T->lchild);
PreOrder(T->rchild);
}
}
//中序遍历
void InOrder(BiTreeNode *T){
if(T!=NULL){
InOrder(T->lchild);
cout<<T->data<<" ";
InOrder(T->rchild);
}
}
//后序遍历
void PostOrder(BiTreeNode *T){
if(T!=NULL){
PostOrder(T->lchild);
PostOrder(T->rchild);
cout<<T->data<<" ";
}
}
//求二叉树深度
int PostTreeDepth(BiTreeNode *T){
if(T==NULL){
return 0;
}
else{
int lheight=PostTreeDepth(T->lchild);
int rheight=PostTreeDepth(T->rchild);
return max(lheight, rheight)+1;
}
}
int main(){
BiTreeNode *T;
cout<<"请输入二叉树的先序序列:";
CreateBiTree(T);
cout<<"先序遍历结果:";
PreOrder(T);
cout<<endl;
cout<<"中序遍历结果:";
InOrder(T);
cout<<endl;
cout<<"后序遍历结果:";
PostOrder(T);
cout<<endl;
cout<<"二叉树深度:"<<PostTreeDepth(T)<<endl;
return 0;
}
```
Python:
```python
# 定义二叉树的节点结构
class BiTreeNode:
def __init__(self, data=None, lchild=None, rchild=None):
self.data = data
self.lchild = lchild
self.rchild = rchild
# 创建二叉树函数
def CreateBiTree(T):
ch = input()
if ch == '#':
T = None
else:
T = BiTreeNode(ch)
T.lchild = CreateBiTree(T.lchild)
T.rchild = CreateBiTree(T.rchild)
return T
# 先序遍历
def PreOrder(T):
if T is not None:
print(T.data, end=' ')
PreOrder(T.lchild)
PreOrder(T.rchild)
# 中序遍历
def InOrder(T):
if T is not None:
InOrder(T.lchild)
print(T.data, end=' ')
InOrder(T.rchild)
# 后序遍历
def PostOrder(T):
if T is not None:
PostOrder(T.lchild)
PostOrder(T.rchild)
print(T.data, end=' ')
# 求二叉树深度
def PostTreeDepth(T):
if T is None:
return 0
else:
lheight = PostTreeDepth(T.lchild)
rheight = PostTreeDepth(T.rchild)
return max(lheight, rheight) + 1
# 主函数
if __name__ == '__main__':
T = None
print("请输入二叉树的先序序列:", end='')
T = CreateBiTree(T)
print("先序遍历结果:", end='')
PreOrder(T)
print()
print("中序遍历结果:", end='')
InOrder(T)
print()
print("后序遍历结果:", end='')
PostOrder(T)
print()
print("二叉树深度:", end='')
print(PostTreeDepth(T))
```
Java:
```java
import java.util.Scanner;
//定义二叉树的节点结构
class BiTreeNode{
char data;
BiTreeNode lchild, rchild;
BiTreeNode(char data){
this.data = data;
this.lchild = null;
this.rchild = null;
}
}
public class Main{
//创建二叉树函数
public static BiTreeNode CreateBiTree(){
Scanner sc = new Scanner(System.in);
char ch = sc.next().charAt(0);
if(ch == '#'){
return null;
}
else{
BiTreeNode T = new BiTreeNode(ch);
T.lchild = CreateBiTree();
T.rchild = CreateBiTree();
return T;
}
}
//先序遍历
public static void PreOrder(BiTreeNode T){
if(T!=null){
System.out.print(T.data+" ");
PreOrder(T.lchild);
PreOrder(T.rchild);
}
}
//中序遍历
public static void InOrder(BiTreeNode T){
if(T!=null){
InOrder(T.lchild);
System.out.print(T.data+" ");
InOrder(T.rchild);
}
}
//后序遍历
public static void PostOrder(BiTreeNode T){
if(T!=null){
PostOrder(T.lchild);
PostOrder(T.rchild);
System.out.print(T.data+" ");
}
}
//求二叉树深度
public static int PostTreeDepth(BiTreeNode T){
if(T==null){
return 0;
}
else{
int lheight=PostTreeDepth(T.lchild);
int rheight=PostTreeDepth(T.rchild);
return Math.max(lheight, rheight)+1;
}
}
public static void main(String args[]){
BiTreeNode T;
System.out.print("请输入二叉树的先序序列:");
T=CreateBiTree();
System.out.print("先序遍历结果:");
PreOrder(T);
System.out.println();
System.out.print("中序遍历结果:");
InOrder(T);
System.out.println();
System.out.print("后序遍历结果:");
PostOrder(T);
System.out.println();
System.out.print("二叉树深度:");
System.out.println(PostTreeDepth(T));
}
}
```
JavaScript:
```javascript
//定义二叉树的节点结构
function BiTreeNode(data){
this.data = data;
this.lchild = null;
this.rchild = null;
}
//创建二叉树函数
function CreateBiTree(){
let ch = prompt();
if(ch == '#'){
return null;
}
else{
let T = new BiTreeNode(ch);
T.lchild = CreateBiTree();
T.rchild = CreateBiTree();
return T;
}
}
//先序遍历
function PreOrder(T){
if(T != null){
console.log(T.data + ' ');
PreOrder(T.lchild);
PreOrder(T.rchild);
}
}
//中序遍历
function InOrder(T){
if(T != null){
InOrder(T.lchild);
console.log(T.data + ' ');
InOrder(T.rchild);
}
}
//后序遍历
function PostOrder(T){
if(T != null){
PostOrder(T.lchild);
PostOrder(T.rchild);
console.log(T.data + ' ');
}
}
//求二叉树深度
function PostTreeDepth(T){
if(T == null){
return 0;
}
else{
let lheight = PostTreeDepth(T.lchild);
let rheight = PostTreeDepth(T.rchild);
return Math.max(lheight, rheight) + 1;
}
}
//主函数
let T = null;
console.log("请输入二叉树的先序序列:");
T = CreateBiTree();
console.log("先序遍历结果:");
PreOrder(T);
console.log();
console.log("中序遍历结果:");
InOrder(T);
console.log();
console.log("后序遍历结果:");
PostOrder(T);
console.log();
console.log("二叉树深度:");
console.log(PostTreeDepth(T));
```
Go:
```go
package main
import "fmt"
//定义二叉树的节点结构
type BiTreeNode struct {
data byte
lchild *BiTreeNode
rchild *BiTreeNode
}
//创建二叉树函数
func CreateBiTree() *BiTreeNode{
var ch byte
fmt.Scanf("%c", &ch)
if(ch == '#'){
return nil
}else{
T := new(BiTreeNode)
T.data = ch
T.lchild = CreateBiTree()
T.rchild = CreateBiTree()
return T
}
}
//先序遍历
func PreOrder(T *BiTreeNode){
if(T != nil){
fmt.Printf("%c ", T.data)
PreOrder(T.lchild)
PreOrder(T.rchild)
}
}
//中序遍历
func InOrder(T *BiTreeNode){
if(T != nil){
InOrder(T.lchild)
fmt.Printf("%c ", T.data)
InOrder(T.rchild)
}
}
//后序遍历
func PostOrder(T *BiTreeNode){
if(T != nil){
PostOrder(T.lchild)
PostOrder(T.rchild)
fmt.Printf("%c ", T.data)
}
}
//求二叉树深度
func PostTreeDepth(T *BiTreeNode) int{
if(T == nil){
return 0
}else{
lheight := PostTreeDepth(T.lchild)
rheight := PostTreeDepth(T.rchild)
if(lheight > rheight){
return lheight + 1
}else{
return rheight + 1
}
}
}
func main(){
var T *BiTreeNode
fmt.Printf("请输入二叉树的先序序列:")
T = CreateBiTree()
fmt.Printf("先序遍历结果:")
PreOrder(T)
fmt.Printf("\n")
fmt.Printf("中序遍历结果:")
InOrder(T)
fmt.Printf("\n")
fmt.Printf("后序遍历结果:")
PostOrder(T)
fmt.Printf("\n")
fmt.Printf("二叉树深度:")
fmt.Printf("%d\n", PostTreeDepth(T))
}
```
阅读全文
相关推荐
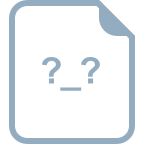
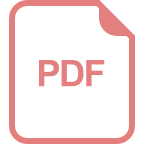
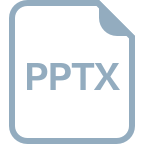
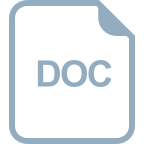
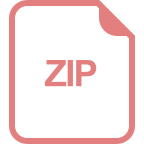
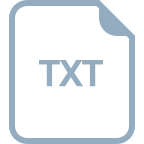
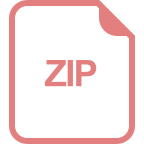
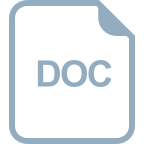
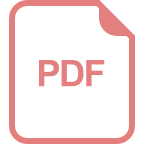
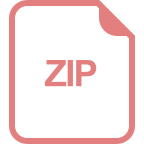
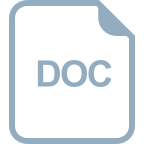
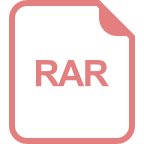