python的json模块配置
时间: 2023-03-06 09:53:57 浏览: 94
JSON模块是Python中一种用于读取、编写和操作JSON格式数据的非常有用的工具。它使用模块内置的函数,可以轻松地将JSON数据转换为Python数据类型,以及将Python数据类型转换为JSON格式的数据。
相关问题
python 读取json配置文件
Python的json模块可以用来读取JSON格式的配置文件。下面是一个简单的示例代码:
```python
import json
# 读取JSON配置文件
with open('config.json') as f:
config = json.load(f)
# 输出配置项
print('Host:', config['host'])
print('Port:', config['port'])
print('Username:', config['username'])
print('Password:', config['password'])
```
假设我们有一个名为config.json的JSON配置文件,其中包含以下内容:
```json
{
"host": "localhost",
"port": 3306,
"username": "admin",
"password": "password123"
}
```
运行上述代码将输出:
```
Host: localhost
Port: 3306
Username: admin
Password: password123
```
这样就可以方便地读取和使用JSON格式的配置文件了。
python json库
Python 中的 json 库是用于处理 JSON 数据的内置模块。它提供了一种将 Python 对象转换为 JSON 格式的方法,并且还提供了一种将 JSON 格式转换为 Python 对象的方法。这对于在 Python 程序中处理 API 响应或配置文件等情况非常有用。
以下是一些常见的使用方法:
1. 将 Python 对象转换为 JSON 格式:
```python
import json
data = {'name': 'John', 'age': 30}
json_str = json.dumps(data) # 将 Python 对象转换为 JSON 字符串
```
2. 将 JSON 格式转换为 Python 对象:
```python
import json
json_str = '{"name": "John", "age": 30}'
data = json.loads(json_str) # 将 JSON 字符串转换为 Python 对象
```
3. 从文件中读取 JSON 数据并转换为 Python 对象:
```python
import json
with open('data.json') as f:
data = json.load(f) # 从文件中读取 JSON 数据并转换为 Python 对象
```
4. 将 Python 对象写入文件中:
```python
import json
data = {'name': 'John', 'age': 30}
with open('data.json', 'w') as f:
json.dump(data, f) # 将 Python 对象写入文件中
```
以上是一些常见的使用方法,更多详细的用法可以参考官方文档。
阅读全文
相关推荐
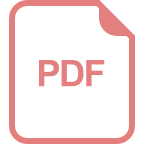
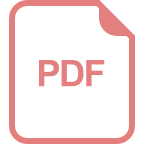
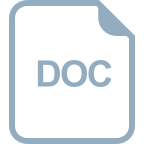
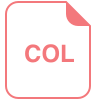
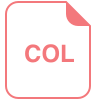
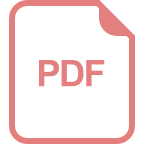
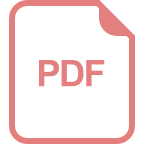
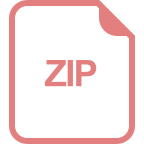
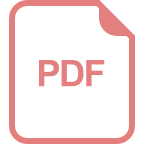
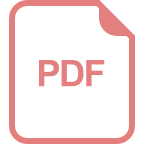
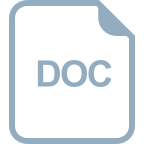
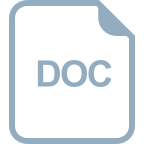
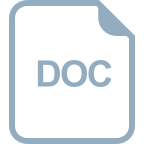


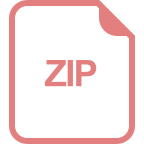