能不能用 python 做一个转盘
时间: 2024-12-17 14:30:50 浏览: 7
当然可以使用 Python 来创建一个转盘游戏。你可以通过随机数生成器和交互式用户界面来模拟这个过程。下面是一个简单的例子,演示如何使用 Tkinter 库创建一个基本的转盘:
```python
import tkinter as tk
from random import randint
# 定义转盘区域和数字
numbers = [str(i) for i in range(1, 7)] # 可以设置成你想要的范围,这里设为1到6
class WheelSpin(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.create_widgets()
def create_widgets(self):
self.canvas = tk.Canvas(self, width=400, height=400, bg='white')
self.canvas.pack(pady=20)
# 创建转盘圆环
outer_circle = self.canvas.create_oval(50, 50, 350, 350, fill='gray', outline='black', width=5)
# 创建指针
self.spinning_marker = self.canvas.create_oval(100, 100, 150, 150, fill='red', outline='black')
# 鼠标移动事件
self.canvas.bind("<B1-Motion>", self.move_spinning_marker)
def move_spinning_marker(self, event):
x = (event.x - self.winfo_rootx()) / float(self.winfo_width())
y = (event.y - self.winfo_rooty()) / float(self.winfo_height())
new_x = int((350 - 50) * x + 50)
new_y = int((350 - 50) * y + 50)
self.canvas.coords(self.spinning_marker, new_x, new_y, new_x+50, new_y+50)
if self.is_over_number(new_x, new_y):
index = self.get_number(new_x, new_y)
self.show_winner(index)
def is_over_number(self, x, y):
radius = 150
for number in numbers:
x0, y0, x1, y1 = self.canvas.bbox(f"{number}_circle")
if ((x > x0 and x < x1) or (x == x0 or x == x1)) and ((y > y0 and y < y1) or (y == y0 or y == y1)):
return True
return False
def get_number(self, x, y):
for i, number in enumerate(numbers):
x0, y0, x1, y1 = self.canvas.bbox(f"{number}_circle")
if ((x > x0 and x < x1) or (x == x0 or x == x1)) and ((y > y0 and y < y1) or (y == y0 or y == y1)):
return i + 1 # 因为我们从1开始计数
def show_winner(self, index):
winner_label = f"恭喜,你赢了! 中奖号码是 {index}"
self.canvas.delete("winner_label") # 如果之前有赢家显示,先删除
self.canvas.create_text(200, 370, text=winner_label, font=("Arial", 24), fill="green")
if __name__ == "__main__":
root = tk.Tk()
game = WheelSpin(root)
game.mainloop()
```
阅读全文
相关推荐
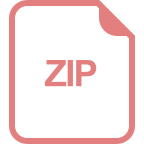
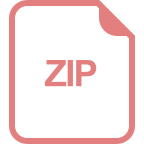
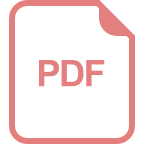



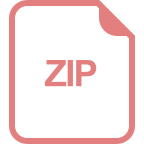











