canvas 3d运动小球
时间: 2023-09-09 18:00:33 浏览: 61
Canvas 3D运动小球是一种使用HTML5的Canvas元素和JavaScript编程技术创建的动画效果。通过在Canvas上绘制一个球体,并通过JavaScript控制球体的位置和运动轨迹,可以实现一个具有3D效果的小球运动动画。
实现这个效果的关键是使用Canvas绘图API来绘制球体的形状和颜色,并通过JavaScript代码实时控制球体在Canvas中的位置和速度。
首先,我们可以创建一个Canvas元素,并设置其宽度和高度。接下来,使用Canvas的绘图API绘制一个圆或球体的形状,并选择一个适当的颜色进行填充。
然后,我们需要使用JavaScript来控制球体的运动。可以使用定时器函数(如setInterval)每隔一定时间间隔更新球体的位置。可以通过改变球体的坐标来移动球体,并在每次更新位置后重新绘制球体。
在移动球体的过程中,可以使用数学公式来计算球体的新位置。例如,可以使用球体的速度、加速度和时间来计算球体在每个时间间隔内的位移,并更新球体的坐标。
此外,还可以添加鼠标或键盘事件监听器,以便通过用户的交互来控制球体的运动。例如,当用户按下某个键盘按键时,可以增加球体的速度或改变其方向。
通过结合Canvas的绘图功能和JavaScript的编程能力,我们可以创建一个3D运动小球的动画效果。这种效果不仅可以增加网页的交互性和趣味性,还可以展示出Canvas和JavaScript的强大功能。
相关问题
canvas实现动态小球碰撞
实现动态小球碰撞需要用到Canvas的2D绘图API,具体步骤如下:
1. 创建Canvas元素和2D绘图上下文
```html
<canvas id="canvas"></canvas>
```
```javascript
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
```
2. 定义小球对象
```javascript
class Ball {
constructor(x, y, r, vx, vy, color) {
this.x = x; // 圆心x坐标
this.y = y; // 圆心y坐标
this.r = r; // 半径
this.vx = vx; // 水平速度
this.vy = vy; // 垂直速度
this.color = color; // 颜色
}
draw() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.r, 0, 2 * Math.PI);
ctx.closePath();
ctx.fillStyle = this.color;
ctx.fill();
}
}
```
3. 创建多个小球对象,并绘制到Canvas上
```javascript
const balls = []; // 存储小球对象的数组
const colors = ['#ff0000', '#00ff00', '#0000ff']; // 小球颜色
for (let i = 0; i < 3; i++) {
const ball = new Ball(
Math.random() * canvas.width, // 随机x坐标
Math.random() * canvas.height, // 随机y坐标
20, // 半径
Math.random() * 4 - 2, // 随机水平速度
Math.random() * 4 - 2, // 随机垂直速度
colors[i] // 随机颜色
);
balls.push(ball);
}
function drawBalls() {
balls.forEach(ball => ball.draw());
}
drawBalls();
```
4. 实现小球碰撞
```javascript
function updateBalls() {
balls.forEach(ball => {
ball.x += ball.vx;
ball.y += ball.vy;
// 碰到边界反弹
if (ball.x < ball.r || ball.x > canvas.width - ball.r) {
ball.vx = -ball.vx;
}
if (ball.y < ball.r || ball.y > canvas.height - ball.r) {
ball.vy = -ball.vy;
}
// 碰撞检测
balls.forEach(otherBall => {
if (ball !== otherBall) {
const dx = ball.x - otherBall.x;
const dy = ball.y - otherBall.y;
const distance = Math.sqrt(dx * dx + dy * dy);
if (distance < ball.r + otherBall.r) {
// 碰撞
const angle = Math.atan2(dy, dx);
const sin = Math.sin(angle);
const cos = Math.cos(angle);
// 旋转坐标系
const x1 = 0;
const y1 = 0;
const x2 = dx * cos + dy * sin;
const y2 = dy * cos - dx * sin;
// 旋转速度向量
const vx1 = ball.vx * cos + ball.vy * sin;
const vy1 = ball.vy * cos - ball.vx * sin;
const vx2 = otherBall.vx * cos + otherBall.vy * sin;
const vy2 = otherBall.vy * cos - otherBall.vx * sin;
// 碰撞后的速度向量
const vx1Final = ((ball.r - otherBall.r) * vx1 + 2 * otherBall.r * vx2) / (ball.r + otherBall.r);
const vx2Final = ((otherBall.r - ball.r) * vx2 + 2 * ball.r * vx1) / (ball.r + otherBall.r);
const vy1Final = vy1;
const vy2Final = vy2;
// 旋转回原坐标系
const x1Final = x1 * cos - y1 * sin;
const y1Final = y1 * cos + x1 * sin;
const x2Final = x2 * cos - y2 * sin;
const y2Final = y2 * cos + x2 * sin;
// 更新位置和速度向量
ball.x = ball.x + (x1Final - x1);
ball.y = ball.y + (y1Final - y1);
ball.vx = vx1Final * cos - vy1Final * sin;
ball.vy = vy1Final * cos + vx1Final * sin;
otherBall.x = ball.x + (x2Final - x1);
otherBall.y = ball.y + (y2Final - y1);
otherBall.vx = vx2Final * cos - vy2Final * sin;
otherBall.vy = vy2Final * cos + vx2Final * sin;
}
}
});
});
}
```
5. 清空Canvas并更新小球位置
```javascript
function clearCanvas() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
}
function loop() {
clearCanvas();
updateBalls();
drawBalls();
requestAnimationFrame(loop);
}
loop();
```
完整代码如下:
```html
<canvas id="canvas"></canvas>
```
```javascript
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
class Ball {
constructor(x, y, r, vx, vy, color) {
this.x = x; // 圆心x坐标
this.y = y; // 圆心y坐标
this.r = r; // 半径
this.vx = vx; // 水平速度
this.vy = vy; // 垂直速度
this.color = color; // 颜色
}
draw() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.r, 0, 2 * Math.PI);
ctx.closePath();
ctx.fillStyle = this.color;
ctx.fill();
}
}
const balls = []; // 存储小球对象的数组
const colors = ['#ff0000', '#00ff00', '#0000ff']; // 小球颜色
for (let i = 0; i < 3; i++) {
const ball = new Ball(
Math.random() * canvas.width, // 随机x坐标
Math.random() * canvas.height, // 随机y坐标
20, // 半径
Math.random() * 4 - 2, // 随机水平速度
Math.random() * 4 - 2, // 随机垂直速度
colors[i] // 随机颜色
);
balls.push(ball);
}
function drawBalls() {
balls.forEach(ball => ball.draw());
}
function updateBalls() {
balls.forEach(ball => {
ball.x += ball.vx;
ball.y += ball.vy;
// 碰到边界反弹
if (ball.x < ball.r || ball.x > canvas.width - ball.r) {
ball.vx = -ball.vx;
}
if (ball.y < ball.r || ball.y > canvas.height - ball.r) {
ball.vy = -ball.vy;
}
// 碰撞检测
balls.forEach(otherBall => {
if (ball !== otherBall) {
const dx = ball.x - otherBall.x;
const dy = ball.y - otherBall.y;
const distance = Math.sqrt(dx * dx + dy * dy);
if (distance < ball.r + otherBall.r) {
// 碰撞
const angle = Math.atan2(dy, dx);
const sin = Math.sin(angle);
const cos = Math.cos(angle);
// 旋转坐标系
const x1 = 0;
const y1 = 0;
const x2 = dx * cos + dy * sin;
const y2 = dy * cos - dx * sin;
// 旋转速度向量
const vx1 = ball.vx * cos + ball.vy * sin;
const vy1 = ball.vy * cos - ball.vx * sin;
const vx2 = otherBall.vx * cos + otherBall.vy * sin;
const vy2 = otherBall.vy * cos - otherBall.vx * sin;
// 碰撞后的速度向量
const vx1Final = ((ball.r - otherBall.r) * vx1 + 2 * otherBall.r * vx2) / (ball.r + otherBall.r);
const vx2Final = ((otherBall.r - ball.r) * vx2 + 2 * ball.r * vx1) / (ball.r + otherBall.r);
const vy1Final = vy1;
const vy2Final = vy2;
// 旋转回原坐标系
const x1Final = x1 * cos - y1 * sin;
const y1Final = y1 * cos + x1 * sin;
const x2Final = x2 * cos - y2 * sin;
const y2Final = y2 * cos + x2 * sin;
// 更新位置和速度向量
ball.x = ball.x + (x1Final - x1);
ball.y = ball.y + (y1Final - y1);
ball.vx = vx1Final * cos - vy1Final * sin;
ball.vy = vy1Final * cos + vx1Final * sin;
otherBall.x = ball.x + (x2Final - x1);
otherBall.y = ball.y + (y2Final - y1);
otherBall.vx = vx2Final * cos - vy2Final * sin;
otherBall.vy = vy2Final * cos + vx2Final * sin;
}
}
});
});
}
function clearCanvas() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
}
function loop() {
clearCanvas();
updateBalls();
drawBalls();
requestAnimationFrame(loop);
}
loop();
```
qml canvas3d
qml canvas3d是Qt Quick中的一个模块,用于构建基于OpenGL ES 2.0的3D图形应用程序。它允许开发人员在QML中直接使用3D图形和效果,而无需借助其他的库或工具。
qml canvas3d提供了一种声明式的方式来创建3D场景。通过定义场景的组件、模型、纹理和渲染效果,开发人员可以轻松地创建复杂的三维场景。此外,qml canvas3d还支持投影、光照、阴影和材质等高级特性,使开发人员能够实现更加逼真的渲染效果。
qml canvas3d使用Qt Quick的基础语法,并与其他Qt Quick组件无缝集成。它可以与Qt Quick Controls相结合,使用Qt Quick的布局系统来管理3D部件的位置和大小。这使得开发人员可以轻松地创建具有丰富用户界面的3D应用程序。
qml canvas3d还支持与其他Qt模块的集成,例如qml timer、qml animation和qml audio。这些模块可以与canvas3d一起使用,为应用程序增加交互性、动画效果和音频功能。
总之,qml canvas3d为开发人员提供了一种方便、快捷的方式来创建强大的3D图形应用程序。通过提供丰富的特性和与其他Qt模块的无缝集成,qml canvas3d成为了构建现代、易用的3D应用程序的理想选择。
相关推荐
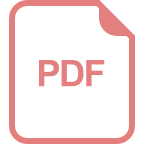
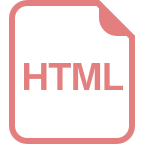
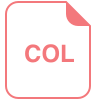











