#include <iostream> #include <vector> #include <cmath> #include <string> using namespace std; // 计算最大公约数 int gcd(int a, int b) { while (b != 0) { int temp = b; b = a % b; a = temp; } return a; } // 获取有理分式的部分分式 vector<string> getPartialFractions(int P[], int Q[], int n) { vector<string> partialFractions; // 计算主次部分 int quotient = P[0] / Q[0]; int remainder = P[0] % Q[0]; if (quotient != 0) { partialFractions.push_back(to_string(quotient)); } if (remainder != 0) { int divisor = gcd(remainder, Q[0]); int numerator = remainder / divisor; int denominator = Q[0] / divisor; partialFractions.push_back("(" + to_string(numerator) + "/" + to_string(denominator) + ")"); } // 计算余项部分 for (int i = 1; i < n; i++) { quotient = P[i] / Q[i]; remainder = P[i] % Q[i]; if (quotient != 0) { partialFractions.push_back(to_string(quotient)); } if (remainder != 0) { int divisor = gcd(remainder, Q[i]); int numerator = remainder / divisor; int denominator = Q[i] / divisor; partialFractions.push_back("(" + to_string(numerator) + "/" + to_string(denominator) + ")/(x-" + to_string(-1 * i) + ")"); } } return partialFractions; } int main() { int P[] = { 0, 0, 0, 1, 0 }; // 分子系数数组 int Q[] = { 1, 2, -3, 0, 0 }; // 分母系数数组 int n = sizeof(P) / sizeof(P[0]); // 系数数组的长度 vector<string> partialFractions = getPartialFractions(P, Q, n); for (int i = 0; i < partialFractions.size(); i++) { cout << partialFractions[i]; if (i != partialFractions.size() - 1) { cout << "+"; } } return 0; } 注释
时间: 2024-04-17 14:22:52 浏览: 118
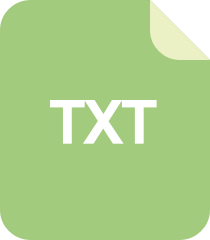
#include_iostream.h_

这是一个用于计算有理分式的程序。它接受两个系数数组P和Q以及一个整数n,其中P是分子系数数组,Q是分母系数数组,n是数组的长度。
程序首先定义了一个计算最大公约数的函数gcd。然后它定义了一个名为getPartialFractions的函数,用于获取有理分式的部分分式。该函数返回一个存储部分分式的字符串向量。
在主函数中,程序初始化了两个示例系数数组P和Q,并通过调用getPartialFractions函数获取部分分式。然后,它使用循环打印输出每个部分分式,并在每个部分分式之间加上"+"号。
最后,程序返回0表示正常运行结束。
如果你有任何问题,请随时问我。
阅读全文
相关推荐
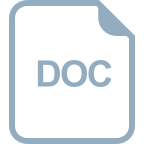
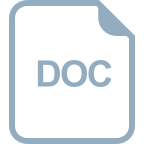
















