利用writable接口进行Hadoop序列化和反序列化,并在此基础上加入readfields方法的调用
时间: 2024-10-20 19:06:28 浏览: 30
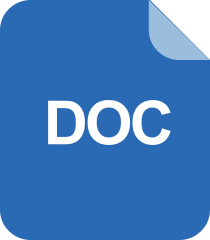
java-Hadoop序列化
在Hadoop生态系统中,Writable接口是一个核心的设计模式,它允许自定义数据类型能够被序列化到磁盘以及在网络通信中传输。为了利用这个接口进行序列化和反序列化,你需要做以下几个步骤:
1. **实现Writable接口**:首先,你的自定义类需要实现`org.apache.hadoop.io.Writable`接口。这意味着你需要提供两个方法:`write(DataOutput out)`用于将对象的状态写入`DataOutput`流中,如`ObjectOutputStream`;`readFields(DataInput in)`则从`DataInput`流,比如`ObjectInputStream`,恢复对象状态。
```java
public class YourCustomClass implements Writable {
// ... 类体 ...
@Override
public void write(DataOutput out) throws IOException {
// 实现写入逻辑,如字段值的序列化
}
@Override
public void readFields(DataInput in) throws IOException {
// 实现读取逻辑,从输入流中恢复字段值
// 这里通常会调用readFields()方法来逐个字段地解析
this.field1 = in.readInt(); // 假设field1是个整数
this.field2 = in.readUTF(); // 字符串类型的field2
}
}
```
2. **序列化与反序列化**:当你需要保存或发送`YourCustomClass`实例时,可以先将其转换为`Writable`兼容的数据结构(通常是`BytesWritable`),然后通过`Writables.writeTo()`方法序列化到字节数组。接收方通过创建`YourCustomClass`实例并调用`readFields()`方法,从字节数组还原对象。
```java
// 序列化
DataOutputBuffer buffer = new DataOutputBuffer();
yourCustomClass.write(buffer);
byte[] serializedBytes = buffer.getData();
// 反序列化
YourCustomClass restoredInstance = new YourCustomClass();
BytesWritable writable = new BytesWritable(serializedBytes);
writable.readFields(new DataInputStream(new ByteArrayInputStream(serializedBytes)));
```
3. **`readfields`方法**:`readFields()`方法是`Writable`接口的主要成员,它负责处理整个对象的字段。当从文件或网络读取时,你会先调用此方法开始解码数据。每个字段在`readFields`内部通常会对应调用自己的`readField()`方法,这样能确保按结构解析所有信息。
阅读全文
相关推荐
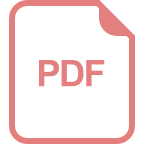
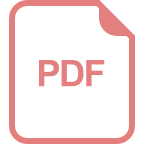
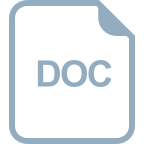
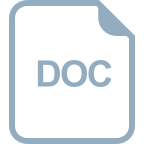
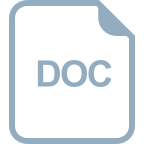
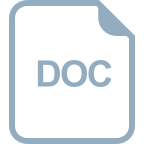
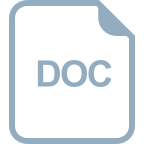
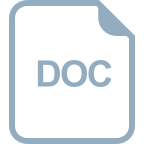
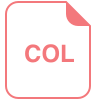
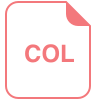
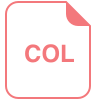
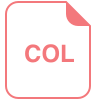
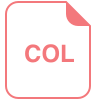
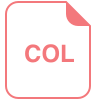


