基于GA-BP神经网络回归预测的Python代码实现
时间: 2024-09-23 13:13:53 浏览: 84
在Python中,结合遗传算法(GA,Genetic Algorithm)和BP(Back Propagation)神经网络进行回归预测,通常会涉及到以下几个步骤。这里我们以`scikit-learn`作为基础库来展示一个简化的例子:
首先,安装所需库:
```bash
pip install numpy pandas scikit-learn matplotlib
```
然后,假设你有一个包含数据集的CSV文件,我们可以按照以下步骤操作:
1. **数据预处理**:
```python
import pandas as pd
df = pd.read_csv('data.csv') # 假设有'features'列和'target'列
X = df['features'].values.reshape(-1, 1) # 特征
y = df['target'] # 目标变量
```
2. **初始化GA**:
```python
from sklearn.model_selection import train_test_split
from deap import base, creator, tools
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
toolbox = base.Toolbox()
toolbox.register("attr_float", random.uniform, -1.0, 1.0)
toolbox.register("individual", tools.initRepeat, creator.Individual,
toolbox.attr_float, n=X.shape[1])
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
```
3. **定义适应度函数**(基于BP网络的MSE):
```python
def eval_fitness(individual, X, y):
# 使用individual参数更新神经网络权重
... (设置神经网络权重)
# 计算预测结果和真实值之间的MSE
error = mean_squared_error(y, predictions)
return error,
toolbox.register("evaluate", eval_fitness, X=X, y=y)
```
4. **遗传算法循环**:
```python
pop = toolbox.population(n=50) # 初始化种群大小
for gen in range(100): # 进行若干代迭代
offspring = tools.selTournament(pop, k=3) # 筛选
offspring = [toolbox.clone(ind) for ind in offspring] # 深拷贝个体避免共享内存
for ind in offspring:
toolbox.mutate(ind) # 变异
invalid_ind = [ind for ind in offspring if not ind.fitness.valid]
fit_values = toolbox.map(toolbox.evaluate, invalid_ind)
for ind, fit in zip(invalid_ind, fit_values):
ind.fitness.values = fit
pop[:] = offspring # 更新种群
best_solution = pop[0] # 最优解
```
5. **基于最优解建立BP神经网络**:
```python
# 根据best_solution更新BP神经网络的权重
... (设置神经网络权重)
```
6. **测试和预测**:
```python
predictions = model.predict(X_test)
```
请注意,这只是一个简化版本,实际应用中可能需要更复杂的编码和参数调整。此外,遗传算法的具体实现可能会根据`deap`库有所不同。
阅读全文
相关推荐
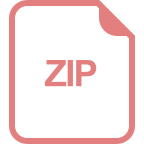
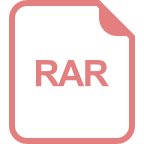
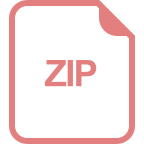
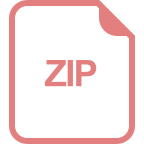
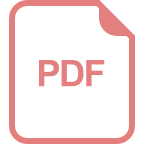
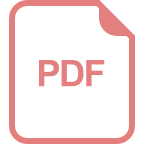



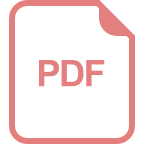
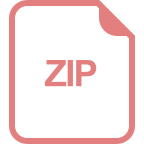
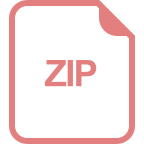
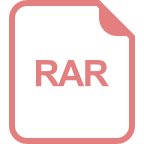
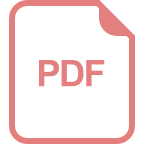
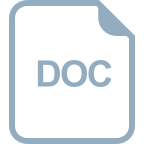
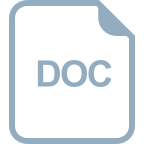
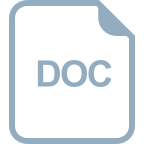
