sklearn实现ga-bp神经网络
时间: 2023-12-21 18:05:30 浏览: 57
可以使用sklearn中的MLPRegressor类来实现GA-BP神经网络。GA-BP神经网络是一种结合了遗传算法和反向传播算法的神经网络,可以用于回归问题。
具体实现步骤如下:
1. 定义GA-BP神经网络模型,包括输入层、隐藏层和输出层的神经元个数、激活函数、遗传算法参数等。
2. 使用遗传算法初始化神经网络的权重和偏置。
3. 使用反向传播算法训练神经网络,得到最优的权重和偏置。
4. 使用训练好的神经网络进行预测。
以下是一个简单的示例代码:
```python
from sklearn.neural_network import MLPRegressor
from sklearn.metrics import mean_squared_error
import numpy as np
import random
# 定义GA-BP神经网络模型
class GABP:
def __init__(self, input_size, hidden_size, output_size, activation='relu', max_iter=100, pop_size=50, mutation_rate=0.1):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
self.activation = activation
self.max_iter = max_iter
self.pop_size = pop_size
self.mutation_rate = mutation_rate
# 遗传算法初始化权重和偏置
def init_weights(self):
weights = []
for i in range(self.pop_size):
w1 = np.random.randn(self.input_size, self.hidden_size)
b1 = np.random.randn(self.hidden_size)
w2 = np.random.randn(self.hidden_size, self.output_size)
b2 = np.random.randn(self.output_size)
weights.append((w1, b1, w2, b2))
return weights
# 计算适应度函数
def fitness(self, X, y, weights):
mse_list = []
for w1, b1, w2, b2 in weights:
model = MLPRegressor(hidden_layer_sizes=(self.hidden_size,), activation=self.activation, max_iter=self.max_iter)
model.coefs_ = [w1, w2]
model.intercepts_ = [b1, b2]
y_pred = model.predict(X)
mse = mean_squared_error(y, y_pred)
mse_list.append(mse)
fitness_list = [1 / (mse + 1e-6) for mse in mse_list]
return fitness_list
# 遗传算法选择
def selection(self, weights, fitness_list):
idx = random.choices(range(self.pop_size), weights=fitness_list, k=2)
return weights[idx[0]], weights[idx[1]]
# 遗传算法交叉
def crossover(self, parent1, parent2):
w1_1, b1_1, w2_1, b2_1 = parent1
w1_2, b1_2, w2_2, b2_2 = parent2
child1 = (w1_1, b1_2, w2_1, b2_2)
child2 = (w1_2, b1_1, w2_2, b2_1)
return child1, child2
# 遗传算法变异
def mutation(self, child):
w1, b1, w2, b2 = child
if random.random() < self.mutation_rate:
w1 += np.random.randn(*w1.shape) * 0.1
if random.random() < self.mutation_rate:
b1 += np.random.randn(*b1.shape) * 0.1
if random.random() < self.mutation_rate:
w2 += np.random.randn(*w2.shape) * 0.1
if random.random() < self.mutation_rate:
b2 += np.random.randn(*b2.shape) * 0.1
return w1, b1, w2, b2
# 训练GA-BP神经网络
def fit(self, X, y):
weights = self.init_weights()
for i in range(self.max_iter):
fitness_list = self.fitness(X, y, weights)
new_weights = []
for j in range(self.pop_size // 2):
parent1 = self.selection(weights, fitness_list)
parent2 = self.selection(weights, fitness_list)
child1, child2 = self.crossover(parent1, parent2)
child1 = self.mutation(child1)
child2 = self.mutation(child2)
new_weights.append(child1)
new_weights.append(child2)
weights = new_weights
fitness_list = self.fitness(X, y, weights)
best_idx = np.argmax(fitness_list)
w1, b1, w2, b2 = weights[best_idx]
self.model = MLPRegressor(hidden_layer_sizes=(self.hidden_size,), activation=self.activation, max_iter=self.max_iter)
self.model.coefs_ = [w1, w2]
self.model.intercepts_ = [b1, b2]
# 预测
def predict(self, X):
return self.model.predict(X)
# 示例
X = np.random.randn(100, 10)
y = np.random.randn(100)
model = GABP(input_size=10, hidden_size=5, output_size=1)
model.fit(X, y)
y_pred = model.predict(X)
print(mean_squared_error(y, y_pred))
```
相关推荐
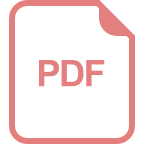














