Tensorflow如何调用ResNet18预训练模型
时间: 2024-04-25 13:05:45 浏览: 251
要调用ResNet18预训练模型,可以使用TensorFlow的keras.applications模块,其中包含了一系列预训练模型,包括ResNet18。可以通过以下代码来调用ResNet18模型:
```python
from keras.applications.resnet import ResNet18
model = ResNet18(include_top=True, weights='imagenet')
```
其中,include_top为True表示保留模型的全连接层,weights='imagenet'表示加载ImageNet上预训练的权重。调用完之后,就可以使用model对图像进行分类或特征提取等任务了。
相关问题
tensorflow如何调用resnet18预训练模型
在TensorFlow中调用ResNet18预训练模型一般有以下步骤:
1. 首先需要导入TensorFlow和对应的预训练模型库,比如Keras。Keras是一个高级神经网络API,它能够以TensorFlow为后端运行。
```python
import tensorflow as tf
from tensorflow.keras.applications import ResNet18
```
2. 加载预训练的ResNet18模型。在加载时,可以通过`weights`参数指定使用预训练权重,通常有'imagenet'、None等选项,'imagenet'表示加载在ImageNet数据集上预训练的权重。
```python
pretrained_model = ResNet18(weights='imagenet')
```
3. 如果需要使用预训练模型进行迁移学习或特征提取,可以通过`include_top=False`参数去掉ResNet18顶部的全连接层,然后对模型进行修改或添加新的层来适应新的任务。
```python
pretrained_model = ResNet18(weights='imagenet', include_top=False)
```
4. 在实际使用模型之前,需要对输入数据进行预处理,以匹配预训练模型所使用的输入格式。通常预训练模型会期望输入图片归一化到一定的范围,并且进行尺寸调整。
```python
from tensorflow.keras.preprocessing import image
import numpy as np
def preprocess_image(image_path):
img = image.load_img(image_path, target_size=(224, 224))
img_array = image.img_to_array(img)
img_array = np.expand_dims(img_array, axis=0)
img_array /= 255.0
return img_array
# 假设有一个图片路径
img_path = 'path_to_your_image.jpg'
input_data = preprocess_image(img_path)
```
5. 使用预处理后的数据进行预测。
```python
predictions = pretrained_model.predict(input_data)
```
6. 解析模型输出的预测结果。通常,输出的第一维包含批次大小,第二维表示类别数量,最后一维通常有1000个类别(对应ImageNet的分类数)。使用`pretrained_model.layers[-1].output_shape`可以查看最后一层的输出形状。
```python
# 假设最后有一个函数用于获取类别名称
predicted_class = decode_predictions(predictions)
```
注意,从TensorFlow 2.x开始,Keras已经是TensorFlow的一部分,因此可以直接使用tf.keras进行操作。
tensorflow调用resnet作为骨干网络
在 TensorFlow 中调用 ResNet 作为骨干网络可以通过使用 TensorFlow 提供的 `tf.keras.applications` 模块来实现。该模块已经包含了多种常用的预训练模型,包括 ResNet 等。
以下是一个使用 ResNet50 作为骨干网络的示例代码:
```python
import tensorflow as tf
from tensorflow.keras.applications.resnet50 import ResNet50
# 加载 ResNet50 模型
resnet = ResNet50(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# 冻结 ResNet50 的权重
for layer in resnet.layers:
layer.trainable = False
# 添加自定义层
x = resnet.output
x = tf.keras.layers.GlobalAveragePooling2D()(x)
x = tf.keras.layers.Dense(1024, activation='relu')(x)
predictions = tf.keras.layers.Dense(num_classes, activation='softmax')(x)
# 定义新的模型
model = tf.keras.models.Model(inputs=resnet.input, outputs=predictions)
```
在代码中,首先通过 `ResNet50` 函数加载预训练的 ResNet50 模型,然后通过设置 `include_top=False` 参数来移除模型的顶部分类层。接着,我们冻结了 ResNet50 的所有权重,以便在训练过程中不会更新这些权重。然后,我们添加了一个全局平均池化层、一个全连接层和一个分类层,用于将 ResNet50 的输出转换为我们想要的输出。最后,我们定义了一个新的模型,将 ResNet50 的输入和输出连接起来。
需要注意的是,这里的 `input_shape` 参数必须与你的输入数据的形状相匹配,`num_classes` 参数是分类数。
阅读全文
相关推荐
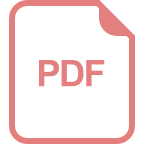
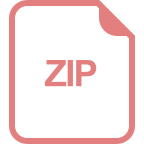
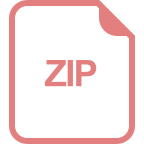













