python如何调用ResNet50模型
时间: 2024-11-09 21:11:22 浏览: 12
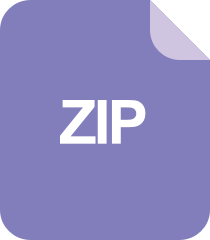
基于Keras预训练模型VGG16、ResNet50、InceptionV3,使用Python的HTTP框架Flask搭建图像识
在Python中,要调用预训练的ResNet50模型,通常需要以下步骤:
1. **安装必要的库**[^1]:
```bash
pip install tensorflow keras flask
```
2. **加载预训练模型**:
```python
from keras.applications.resnet50 import ResNet50
resnet_model = ResNet50(weights='imagenet') # 加载预训练权重
```
3. **模型前向传播(预测)**:
```python
def predict_image(image_path):
img = preprocess_image(image_path) # 对输入图像进行预处理
predictions = resnet_model.predict(img)
return predictions
```
4. **预处理函数**[^2],这可能包括转换成Tensor、调整大小等:
```python
def preprocess_image(image_path):
transform = transforms.Compose([
transforms.Resize(224), # 调整图片尺寸
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]) # 归一化
])
image = Image.open(image_path)
return transform(image).unsqueeze(0) # 添加batch dimension (1, channels, height, width)
```
5. **将模型集成到Flask应用中**,例如创建一个API端点来接收请求并返回预测结果:
```python
app = Flask(__name__)
@app.route('/predict', methods=['POST'])
def predict_api():
file = request.files['image']
prediction = predict_image(file.stream)
return jsonify(prediction)
```
请注意,上述代码示例假设你已经有一个Flask应用并且`request`对象可以访问上传的文件。实际部署时,可能还需要考虑多线程/进程安全以及错误处理。
阅读全文
相关推荐
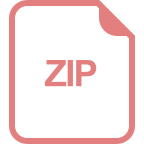
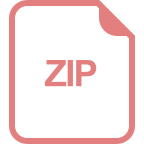

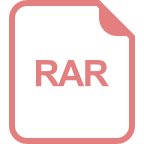
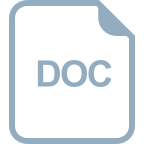












