resnet50代码tensorflow
时间: 2023-09-22 10:01:58 浏览: 138

resnet50_tensorflow

### 回答1:
以下是使用TensorFlow实现的ResNet50代码:
```python
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv2D, BatchNormalization, Activation, MaxPooling2D, AveragePooling2D, Flatten, Dense, Add
from tensorflow.keras.models import Model
def conv_block(input_tensor, kernel_size, filters, stage, block, strides=(2, 2)):
"""A convolution block for ResNet50."""
filters1, filters2, filters3 = filters
conv_name_base = 'res' + str(stage) + block + '_branch'
bn_name_base = 'bn' + str(stage) + block + '_branch'
x = Conv2D(filters1, (1, 1), strides=strides, name=conv_name_base + '2a')(input_tensor)
x = BatchNormalization(name=bn_name_base + '2a')(x)
x = Activation('relu')(x)
x = Conv2D(filters2, kernel_size, padding='same', name=conv_name_base + '2b')(x)
x = BatchNormalization(name=bn_name_base + '2b')(x)
x = Activation('relu')(x)
x = Conv2D(filters3, (1, 1), name=conv_name_base + '2c')(x)
x = BatchNormalization(name=bn_name_base + '2c')(x)
shortcut = Conv2D(filters3, (1, 1), strides=strides, name=conv_name_base + '1')(input_tensor)
shortcut = BatchNormalization(name=bn_name_base + '1')(shortcut)
x = Add()([x, shortcut])
x = Activation('relu')(x)
return x
def identity_block(input_tensor, kernel_size, filters, stage, block):
"""An identity block for ResNet50."""
filters1, filters2, filters3 = filters
conv_name_base = 'res' + str(stage) + block + '_branch'
bn_name_base = 'bn' + str(stage) + block + '_branch'
x = Conv2D(filters1, (1, 1), name=conv_name_base + '2a')(input_tensor)
x = BatchNormalization(name=bn_name_base + '2a')(x)
x = Activation('relu')(x)
x = Conv2D(filters2, kernel_size, padding='same', name=conv_name_base + '2b')(x)
x = BatchNormalization(name=bn_name_base + '2b')(x)
x = Activation('relu')(x)
x = Conv2D(filters3, (1, 1), name=conv_name_base + '2c')(x)
x = BatchNormalization(name=bn_name_base + '2c')(x)
x = Add()([x, input_tensor])
x = Activation('relu')(x)
return x
def ResNet50(input_shape=(224, 224, 3), classes=1000):
"""ResNet50 model."""
img_input = Input(shape=input_shape)
x = Conv2D(64, (7, 7), strides=(2, 2), padding='same', name='conv1')(img_input)
x = BatchNormalization(name='bn_conv1')(x)
x = Activation('relu')(x)
x = MaxPooling2D((3, 3), strides=(2, 2))(x)
x = conv_block(x, 3, [64, 64, 256], stage=2, block='a', strides=(1, 1))
x = identity以下是在 TensorFlow 中实现 ResNet50 的代码示例:
```
import tensorflow as tf
from tensorflow.keras import layers
def identity_block(input_tensor, kernel_size, filters, stage, block):
"""ResNet50 中的恒等块"""
# 定义命名空间
conv_name_base = f"res{stage}_{block}_branch"
bn_name_base = f"bn{stage}_{block}_branch"
# 获取过滤器的数量
filters1, filters2, filters3 = filters
# 第一层
x = layers.Conv2D(filters1, (1, 1), name=conv_name_base + "2a")(input_tensor)
x = layers.BatchNormalization(name=bn_name_base + "2a")(x)
x = layers.Activation("relu")(x)
# 第二层
x = layers.Conv2D(filters2, kernel_size, padding="same", name=conv_name_base + "2b")(x)
x = layers.BatchNormalization(name=bn_name_base + "2b")(x)
x = layers.Activation("relu")(x)
# 第三层
x = layers.Conv2D(filters3, (1, 1), name=conv_name_base + "2c")(x)
x = layers.BatchNormalization(name=bn_name_base + "2c")(x)
# 添加恒等连接
x = layers.add([x, input_tensor])
x = layers.Activation("relu")(x)
return x
def conv_block(input_tensor, kernel_size, filters, stage, block, strides=(2, 2)):
"""ResNet50 中的卷积块"""
# 定义命名空间
conv_name_base = f"res{stage}_{block}_branch"
bn_name_base = f"bn{stage}_{block}_branch"
# 获取过滤器的数量
filters1, filters2, filters3 = filters
# 第一层
x = layers.Conv2D(filters1, (1, 1), strides=strides, name=conv_name_base + "2a")(input_tensor)
x = layers.BatchNormalization(name=bn_name_base + "2a")(x)
x = layers.Activation("relu")(x)
# 第二层
x = layers.Conv2D(filters2, kernel_size, padding="same", name=conv_name_base + "2b")(x)
x = layers.BatchNormalization(name=bn_name_base + "2b")(x)
x = layers.Activation("relu")(x)
# 第三层
x = layers.Conv2D(filters3, (1, 1), name=conv_name_base + "2c")(x)
x = layers.BatchNormalization(name=bn_name_base + "2c")(x)
# 添加卷积连接
shortcut = layers.Conv2D(filters3, (1, 1), strides=strides, name=conv_name_base + "1")(input_tensor)
shortcut = layers.BatchNormalization(name=bn_name_base + "1")(shortcut)
x = layers.add([x, shortcut])
x = layers.Activation("relu")(x)
return x
def ResNet50(input_shape=(224, 224, 3), classes=1000):
"""创建 ResNet50 网络模型"""
# 定义输入张量
input_tensor = layers.Input(shape=input以下是使用 TensorFlow 实现的 ResNet50 代码示例:
```python
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv2D, BatchNormalization, Activation, MaxPooling2D, AveragePooling2D, ZeroPadding2D, Dense, Flatten, Add
from tensorflow.keras.models import Model
def conv_bn_relu(x, filters, kernel_size, strides=1, padding='same'):
x = Conv2D(filters=filters, kernel_size=kernel_size, strides=strides, padding=padding)(x)
x = BatchNormalization()(x)
x = Activation('relu')(x)
return x
def identity_block(x, filters):
shortcut = x
x = conv_bn_relu(x, filters=filters, kernel_size=1)
x = conv_bn_relu(x, filters=filters, kernel_size=3)
x = conv_bn_relu(x, filters=4 * filters, kernel_size=1)
x = Add()([shortcut, x])
x = Activation('relu')(x)
return x
def projection_block(x, filters, strides):
shortcut = x
x = conv_bn_relu(x, filters=filters, kernel_size=1, strides=strides)
x = conv_bn_relu(x, filters=filters, kernel_size=3)
x = conv_bn_relu(x, filters=4 * filters, kernel_size=1)
shortcut = Conv2D(filters=4 * filters, kernel_size=1, strides=strides)(shortcut)
shortcut = BatchNormalization()(shortcut)
x = Add()([shortcut, x])
x = Activation('relu')(x)
return x
def resnet50(input_shape=(224, 224, 3), num_classes=1000):
inputs = Input(shape=input_shape)
x = ZeroPadding2D(padding=(3, 3))(inputs)
x = conv_bn_relu(x, filters=64, kernel_size=7, strides=2)
x = MaxPooling2D(pool_size=(3, 3), strides=2)(x)
x = projection_block(x, filters=64, strides=1)
x = identity_block(x, filters=64)
x = identity_block(x, filters=64)
x = projection_block(x, filters=128, strides=2)
x = identity_block(x, filters=128)
x = identity_block(x, filters=128)
x = identity_block(x, filters=128)
x = projection_block(x, filters=256, strides=2)
x = identity_block(x, filters=256)
x = identity_block(x, filters=256)
x = identity_block(x, filters=256)
x = identity_block(x, filters=256)
x = identity_block(x, filters=256)
x = projection_block(x, filters=512, strides=2)
x = identity_block(x, filters=512)
x = identity_block(x, filters=512)
x = AveragePooling2D(pool_size=(7, 7))(x)
x = Flatten()(x)
outputs = Dense(units=num_classes, activation='softmax')(x)
model = Model(inputs=inputs, outputs=outputs)
return model
```
这是一个定义 ResNet50 模型的代码。你可以通过调用 `resnet50()` 函数来创建一个 ResNet50 模型。在调用该函数时,你可以传入输入张量的形状和分类的数量,以创建一个适合你的模型。
### 回答2:
ResNet-50是一个非常流行的深度学习模型,用于图像分类和目标检测任务。为了在TensorFlow中实现ResNet-50模型,需要按照以下步骤进行编码:
1. 导入所需的库和模块:
```python
import tensorflow as tf
from tensorflow.keras.applications.resnet50 import ResNet50
from tensorflow.keras.preprocessing import image
from tensorflow.keras.applications.resnet50 import preprocess_input, decode_predictions
```
2. 加载预训练的ResNet-50模型:
```python
model = ResNet50(weights='imagenet')
```
3. 加载并预处理待分类的图像:
```python
img_path = 'path_to_image.jpg'
img = image.load_img(img_path, target_size=(224, 224))
x = image.img_to_array(img)
x = np.expand_dims(x, axis=0)
x = preprocess_input(x)
```
4. 使用加载的模型做出预测:
```python
preds = model.predict(x)
```
5. 解码预测结果:
```python
decoded_preds = decode_predictions(preds, top=3)[0] # 解码前三个最可能的结果
for pred in decoded_preds:
print(pred[1], pred[2]) # 打印类别和相应的概率
```
这些步骤将允许你使用TensorFlow中的ResNet-50模型进行图像分类和预测。请注意,这只是一个简单的示例,具体的实现可能因项目需求而有所不同。
### 回答3:
ResNet-50是深度学习中一种非常流行的卷积神经网络模型,广泛应用于图像分类和目标检测等任务。以下是使用TensorFlow实现ResNet-50代码的简要介绍。
首先,我们需要导入所需的库。
import tensorflow as tf
from tensorflow.keras.applications.resnet50 import ResNet50
from tensorflow.keras.preprocessing import image
from tensorflow.keras.applications.resnet50 import preprocess_input, decode_predictions
接着,我们可以加载预训练的ResNet-50模型。
model = ResNet50(weights='imagenet')
然后,我们可以使用此模型进行图像分类。假设我们有一张名为"image.jpg"的图像。
img_path = 'image.jpg'
img = image.load_img(img_path, target_size=(224, 224))
x = image.img_to_array(img)
x = np.expand_dims(x, axis=0)
x = preprocess_input(x)
使用ResNet-50模型进行预测。
preds = model.predict(x)
print('Predicted:', decode_predictions(preds, top=3)[0])
最后,我们可以打印出预测结果,显示前三个最可能的类别。decode_predictions函数将数值预测结果转换为可读的标签。
这是使用TensorFlow实现ResNet-50的简要代码示例。请注意,上述代码可能需要在运行前先安装相关库,并根据实际需求进行适当调整。
阅读全文
相关推荐
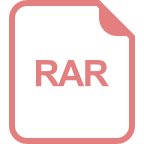
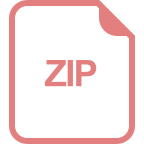


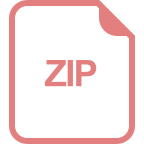
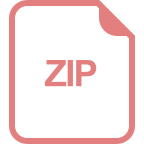








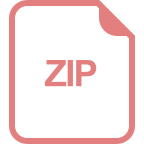


