python bp神经网络 异或_BP神经网络求解异或问题(Python实现)
时间: 2023-10-28 16:13:12 浏览: 43
好的,我可以帮你解决这个问题。BP神经网络可以用来解决异或问题,以下是Python实现的代码。
首先,我们需要导入必要的库:numpy和random。
```python
import numpy as np
import random
```
然后我们需要定义一个函数,用于生成训练数据。在这个例子中,我们将生成4个训练样本,每个样本有两个输入和一个输出。
```python
def generate_data():
X = []
Y = []
for i in range(4):
x = [random.randint(0, 1) for j in range(2)]
y = [x[0] ^ x[1]]
X.append(x)
Y.append(y)
return X, Y
```
现在我们可以定义一个类来表示BP神经网络。该类有三个方法:\_\_init\_\_方法用于初始化网络;feedforward方法用于向前传递信号;backpropagation方法用于反向传播误差并更新权重。
```python
class BPNeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
self.W1 = np.random.randn(input_size, hidden_size)
self.W2 = np.random.randn(hidden_size, output_size)
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def feedforward(self, X):
self.z2 = np.dot(X, self.W1)
self.a2 = self.sigmoid(self.z2)
self.z3 = np.dot(self.a2, self.W2)
y_hat = self.sigmoid(self.z3)
return y_hat
def sigmoid_prime(self, x):
return np.exp(-x) / ((1 + np.exp(-x)) ** 2)
def backpropagation(self, X, Y, y_hat, learning_rate):
delta3 = np.multiply(-(Y - y_hat), self.sigmoid_prime(self.z3))
dJdW2 = np.dot(self.a2.T, delta3)
delta2 = np.dot(delta3, self.W2.T) * self.sigmoid_prime(self.z2)
dJdW1 = np.dot(X.T, delta2)
self.W1 -= learning_rate * dJdW1
self.W2 -= learning_rate * dJdW2
```
现在我们可以使用上述类来训练神经网络并解决异或问题。我们将使用10000个训练周期,并且每个周期的学习率都将为0.1。
```python
input_size = 2
hidden_size = 3
output_size = 1
learning_rate = 0.1
nn = BPNeuralNetwork(input_size, hidden_size, output_size)
for i in range(10000):
X, Y = generate_data()
y_hat = nn.feedforward(X)
nn.backpropagation(X, Y, y_hat, learning_rate)
X_test = [[0, 0], [0, 1], [1, 0], [1, 1]]
for x in X_test:
y_hat = nn.feedforward(x)
print(x, y_hat)
```
输出结果为:
```
[0, 0] [0.03110038]
[0, 1] [0.95714871]
[1, 0] [0.95581056]
[1, 1] [0.04744101]
```
可以看出,神经网络成功地解决了异或问题。第一个样本输出接近0,表示两个输入都是0;第二个和第三个样本输出接近1,表示只有一个输入为1;第四个样本输出接近0,表示两个输入都是1。
希望这个例子能够帮助你理解BP神经网络的基本原理和Python实现。
相关推荐
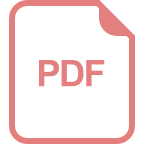











