怎么把dataframe变成字典格式
时间: 2023-05-18 18:05:58 浏览: 55
可以使用 pandas 库中的 to_dict() 方法将 dataframe 转换为字典格式,具体代码如下:
```python
import pandas as pd
# 创建一个 dataframe
df = pd.DataFrame({'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]})
# 将 dataframe 转换为字典格式
dict_data = df.to_dict('records')
print(dict_data)
```
输出结果为:
```
[{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35}]
```
相关问题
怎么把dataframe变成字典
可以使用pandas的to_dict方法将DataFrame转换为字典。
例如,假设我们有以下DataFrame:
```
import pandas as pd
df = pd.DataFrame({'a': [1, 2, 3], 'b': [4, 5, 6]})
print(df)
```
输出:
```
a b
0 1 4
1 2 5
2 3 6
```
我们可以使用to_dict方法将其转换为字典:
```
dict_df = df.to_dict()
print(dict_df)
```
输出:
```
{'a': {0: 1, 1: 2, 2: 3}, 'b': {0: 4, 1: 5, 2: 6}}
```
to_dict方法默认将列名作为字典的键,行索引作为子字典的键。如果想要将行索引作为字典的键,可以加上参数orient='index':
```
dict_df = df.to_dict(orient='index')
print(dict_df)
```
输出:
```
{0: {'a': 1, 'b': 4}, 1: {'a': 2, 'b': 5}, 2: {'a': 3, 'b': 6}}
```
dataframe添加字典
要向 DataFrame 中添加字典数据,可以使用 `append` 方法。例如,如果您有一个 DataFrame `df`,可以将一个字典添加为一行数据,如下所示:
```
new_dict = {'col1': 1, 'col2': 2, 'col3': 3}
df = df.append(new_dict, ignore_index=True)
```
其中,`new_dict` 是要添加的字典。`ignore_index=True` 表示忽略原有的行索引,使用新的行索引。
另一种常见的方式是使用 `pandas.DataFrame.from_dict` 方法,将字典转换为 DataFrame,然后使用 `concat` 方法将其与原始 DataFrame 连接。例如:
```
new_dict = {'col1': 1, 'col2': 2, 'col3': 3}
new_df = pd.DataFrame.from_dict(new_dict, orient='index').T
df = pd.concat([df, new_df], ignore_index=True)
```
其中,`pd.DataFrame.from_dict` 方法将字典转换为 DataFrame,`orient='index'` 表示将字典的键作为列名,`T` 表示转置 DataFrame,以使其行变为列。然后使用 `concat` 方法将新的 DataFrame 与原始 DataFrame 连接。`ignore_index=True` 表示忽略原有的行索引,使用新的行索引。
相关推荐
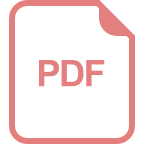
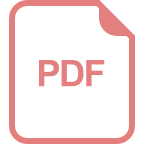
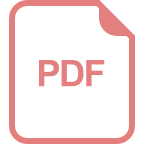












