死锁避免算法的实现,用 Java 语言
时间: 2024-03-14 08:46:01 浏览: 67
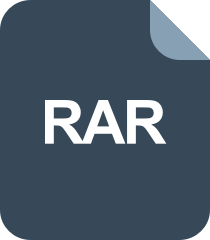
用java语言编程实现银行家算法BankerAlgorithm
Java中实现死锁避免算法可以使用银行家算法(Banker's Algorithm),以下是一个简单的 Java 代码实现:
```java
import java.util.Scanner;
public class BankersAlgorithm {
// 资源数量
private int m;
// 进程数量
private int n;
// 已分配资源矩阵
private int[][] allocation;
// 最大需求矩阵
private int[][] max;
// 可用资源向量
private int[] available;
// 需求矩阵
private int[][] need;
// 初始化资源数据
public void init() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入资源数量m:");
m = scanner.nextInt();
System.out.print("请输入进程数量n:");
n = scanner.nextInt();
allocation = new int[n][m];
max = new int[n][m];
available = new int[m];
need = new int[n][m];
System.out.println("请输入各进程已分配的资源数:");
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
allocation[i][j] = scanner.nextInt();
}
}
System.out.println("请输入各进程最大需求量:");
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
max[i][j] = scanner.nextInt();
}
}
System.out.println("请输入各类资源的总数量:");
for (int i = 0; i < m; i++) {
available[i] = scanner.nextInt();
}
scanner.close();
}
// 计算需求矩阵
public void calculateNeed() {
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
}
// 检查某个进程是否满足条件
public boolean checkProcess(int processIndex, int[] work, boolean[] finish) {
for (int i = 0; i < m; i++) {
if (need[processIndex][i] > work[i]) {
return false;
}
}
for (int i = 0; i < m; i++) {
work[i] += allocation[processIndex][i];
}
finish[processIndex] = true;
return true;
}
// 执行银行家算法
public void run() {
calculateNeed();
int[] work = new int[m];
System.arraycopy(available, 0, work, 0, m);
boolean[] finish = new boolean[n];
for (int i = 0; i < n; i++) {
finish[i] = false;
}
int count = 0;
while (count < n) {
boolean flag = false;
for (int i = 0; i < n; i++) {
if (!finish[i] && checkProcess(i, work, finish)) {
count++;
flag = true;
}
}
if (!flag) {
System.out.println("系统不安全!");
return;
}
}
System.out.println("系统安全,安全序列为:");
for (int i = 0; i < n; i++) {
System.out.print("P" + i + " ");
}
}
public static void main(String[] args) {
BankersAlgorithm algorithm = new BankersAlgorithm();
algorithm.init();
algorithm.run();
}
}
```
在上述代码中,BankersAlgorithm类表示银行家算法的实现类。在init()方法中,初始化资源数据。在calculateNeed()方法中,计算需求矩阵。在checkProcess()方法中,检查某个进程是否满足条件。在run()方法中,执行银行家算法,计算系统的安全序列。在main()方法中,创建BankersAlgorithm对象并调用init()和run()方法,执行银行家算法。
需要注意的是,银行家算法只能检测系统当前状态是否安全,不能保证系统一定处于安全状态。因此,在使用银行家算法时,需要谨慎处理。
阅读全文
相关推荐
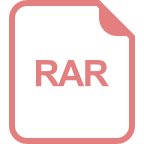
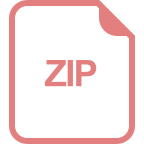














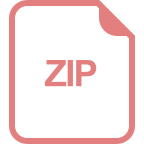
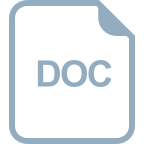