写个银行家算法代码 JAVA语言
时间: 2023-08-31 15:39:18 浏览: 121
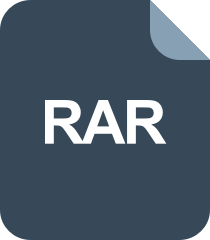
java编写银行家算法
### 回答1:
public class BankerAlgorithm {
static int m, n;
static int allocation[][] = new int[10][10];
static int max[][] = new int[10][10];
static int need[][] = new int[10][10];
static int available[] = new int[10];
static int sequence[] = new int[10];
static int counter = 0;
public static void main(String args[])
{
System.out.println("Enter the number of processes and resources : ");
Scanner sc = new Scanner(System.in);
m = sc.nextInt();
n = sc.nextInt();
System.out.println("Enter the Allocation Matrix : ");
for (int i = 0; i < m; i++)
for (int j = 0; j < n; j++)
allocation[i][j] = sc.nextInt();
System.out.println("Enter the Max Matrix : ");
for (int i = 0; i < m; i++)
for (int j = 0; j < n; j++)
max[i][j] = sc.nextInt();
System.out.println("Enter the Available Vector : ");
for (int j = 0; j < n; j++)
available[j] = sc.nextInt();
//calculate the need matrix
for (int i = 0; i < m; i++)
for (int j = 0; j < n; j++)
need[i][j] = max[i][j] - allocation[i][j];
//run the algorithm
boolean done[] = new boolean[m];
int j = 0;
while (j < m) {
boolean allocated = false;
for (int i = 0; i < m; i++)
{
if (!done[i] && check(i))
{
for (int k = 0; k < n; k++)
available[k] = available[k] - need[i][k] + max[i][k];
System.out.println("Allocated process : " + i);
allocated = done[i] = true;
sequence[counter++] = i;
j++;
}
}
if (!allocated) break;
}
if (j == m)
{
System.out.println("\nSafely allocated");
System.out.println("Safe Sequence is : ");
for (int i = 0; i < m - 1; i++)
System.out.print(sequence[i] + " -> ");
System.out.print(sequence[m - 1]);
}
else
System.out.println("All proceess cant be allocated safely");
}
static boolean check(int i)
{
for (int j = 0; j < n; j++)
if (need[i][j] > available[j])
return false;
return true;
}
}
### 回答2:
银行家算法是一种确保系统资源分配安全的算法,用于避免死锁的发生。下面给出一个简单的银行家算法的示例代码(使用JAVA语言):
```java
import java.util.Arrays;
public class BankerAlgorithm {
private int[][] max; // 最大需求矩阵
private int[][] allocation; // 已分配资源矩阵
private int[][] need; // 剩余需求矩阵
private int[] available; // 可用资源向量
private boolean[] finished; // 标记进程是否执行完毕
public BankerAlgorithm(int[][] max, int[][] allocation, int[] available) {
this.max = max;
this.allocation = allocation;
this.available = available;
this.finished = new boolean[max.length];
this.need = new int[max.length][max[0].length];
calculateNeed();
}
// 计算剩余需求矩阵
private void calculateNeed() {
for (int i = 0; i < max.length; i++) {
for (int j = 0; j < max[i].length; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
}
// 判断进程是否能够安全执行
public boolean isSafe() {
int processCount = max.length; // 进程数量
int resourceCount = max[0].length; // 资源数量
int[] work = Arrays.copyOf(available, available.length); // 创建一个可用资源副本
boolean[] finish = Arrays.copyOf(finished, finished.length); // 创建一个finish副本
int[] safeSequence = new int[processCount];
int index = 0; // 安全序列下标
while (index < processCount) {
boolean isSafe = false;
for (int i = 0; i < processCount; i++) {
if (!finish[i]) {
boolean isProcessAvailable = true;
for (int j = 0; j < resourceCount; j++) {
if (need[i][j] > work[j]) {
isProcessAvailable = false;
break;
}
}
if (isProcessAvailable) {
for (int k = 0; k < resourceCount; k++) {
work[k] += allocation[i][k];
}
safeSequence[index++] = i; // 添加到安全序列中
finish[i] = true; // 标记进程已执行完毕
isSafe = true;
}
}
}
if (!isSafe) {
break;
}
}
// 判断是否所有进程都能安全执行
boolean isAllFinished = true;
for (boolean f : finish) {
if (!f) {
isAllFinished = false;
break;
}
}
// 输出安全序列
if (isAllFinished) {
System.out.println("安全序列:");
for (int i = 0; i < processCount - 1; i++) {
System.out.print("P" + safeSequence[i] + " -> ");
}
System.out.print("P" + safeSequence[processCount - 1]);
System.out.println();
} else {
System.out.println("无安全序列,系统处于不安全状态!");
}
return isAllFinished;
}
public static void main(String[] args) {
// 定义三个进程和三个资源
int[][] max = {{7, 5, 3}, {3, 2, 2}, {9, 0, 2}};
int[][] allocation = {{0, 1, 0}, {2, 0, 0}, {3, 0, 2}};
int[] available = {3, 3, 2};
BankerAlgorithm banker = new BankerAlgorithm(max, allocation, available);
banker.isSafe();
}
}
```
以上代码通过银行家算法判断系统是否处于安全状态,如果是,则输出安全序列;如果不是,则输出无安全序列,并提示系统处于不安全状态。
阅读全文
相关推荐
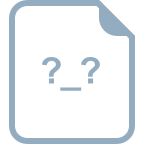
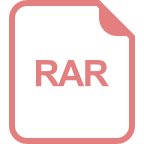
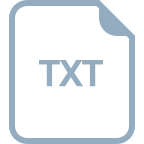
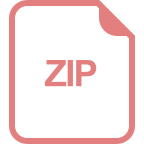
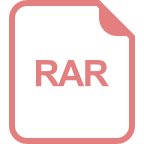
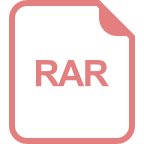
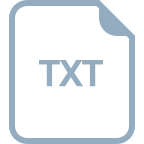
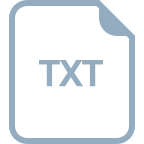
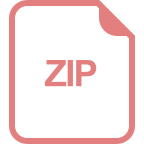
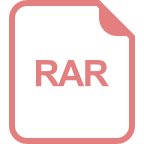
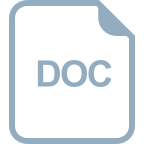
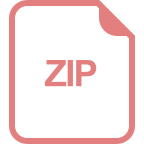
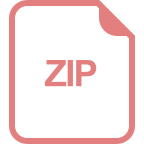
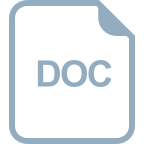
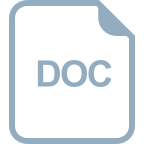

