设计一个名为MyInteger的类,类包含: 一个名为value的int型数据域,保存此对象表示的int型值。 一个用指定的int型值创建一个MyInteger对象的构造函数。 一个返回int型值的get函数。 只读函数isEven()、isOdd()和isPrime(),分别在整数为偶数、奇数或素数的情况下返回真。 静态函数isEven(int)、isOdd(int)以及isPrime(int),分别在给定整数为偶数、奇数或素数的情况下返回真。 静态函数isEven(const MyInteger&)、isOdd(const MyInteger&)和isPrime(const MyInteger&),分别在给定对象表示的整数为偶数、奇数或素数的情况下返回真。 只读函数equals(int)和equals(const MyInteger&),在本对象表示的整数值等于给定值的情况下返回真。 一个静态函数parseInt(const string&).将一个字符串转换为一个int型值。 实现类。在main()中,测试类的所有函数。
时间: 2024-02-22 10:58:08 浏览: 126
好的,这个问题需要我们设计一个类 MyInteger,具体的实现如下:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class MyInteger {
private:
int value;
public:
MyInteger(int v) : value(v) {}
int get() const {
return value;
}
bool isEven() const {
return value % 2 == 0;
}
bool isOdd() const {
return value % 2 == 1;
}
bool isPrime() const {
if (value < 2) {
return false;
}
int limit = sqrt(value);
for (int i = 2; i <= limit; i++) {
if (value % i == 0) {
return false;
}
}
return true;
}
static bool isEven(int n) {
return n % 2 == 0;
}
static bool isOdd(int n) {
return n % 2 == 1;
}
static bool isPrime(int n) {
if (n < 2) {
return false;
}
int limit = sqrt(n);
for (int i = 2; i <= limit; i++) {
if (n % i == 0) {
return false;
}
}
return true;
}
bool equals(int n) const {
return value == n;
}
bool equals(const MyInteger& other) const {
return value == other.value;
}
static int parseInt(const string& s) {
int result = 0;
for (int i = 0; i < s.size(); i++) {
if (isdigit(s[i])) {
result = result * 10 + (s[i] - '0');
}
}
return result;
}
};
```
上面的代码中,我们定义了一个类 MyInteger,包含一个数据成员 value,一个构造函数和一些成员函数和静态函数。具体的说明如下:
- 构造函数 MyInteger(int v):用指定的 int 值创建一个 MyInteger 对象。
- 成员函数 int get() const:返回 MyInteger 对象的 int 值。
- 成员函数 bool isEven() const:判断 MyInteger 对象的 int 值是否为偶数,如果是,返回 true;否则,返回 false。
- 成员函数 bool isOdd() const:判断 MyInteger 对象的 int 值是否为奇数,如果是,返回 true;否则,返回 false。
- 成员函数 bool isPrime() const:判断 MyInteger 对象的 int 值是否为素数,如果是,返回 true;否则,返回 false。
- 静态函数 bool isEven(int n):判断指定的 int 值是否为偶数,如果是,返回 true;否则,返回 false。
- 静态函数 bool isOdd(int n):判断指定的 int 值是否为奇数,如果是,返回 true;否则,返回 false。
- 静态函数 bool isPrime(int n):判断指定的 int 值是否为素数,如果是,返回 true;否则,返回 false。
- 成员函数 bool equals(int n) const:判断 MyInteger 对象的 int 值是否等于指定的 int 值,如果相等,返回 true;否则,返回 false。
- 成员函数 bool equals(const MyInteger& other) const:判断 MyInteger 对象的 int 值是否等于另一个 MyInteger 对象的 int 值,如果相等,返回 true;否则,返回 false。
- 静态函数 int parseInt(const string& s):将一个字符串转换为一个 int 值。
在 main 函数中,我们可以测试 MyInteger 类的所有函数,具体的代码如下:
```cpp
int main() {
MyInteger a(5);
cout << a.get() << endl;
cout << a.isEven() << endl;
cout << a.isOdd() << endl;
cout << a.isPrime() << endl;
cout << MyInteger::isEven(6) << endl;
cout << MyInteger::isOdd(7) << endl;
cout << MyInteger::isPrime(7) << endl;
MyInteger b(7);
cout << a.equals(b) << endl;
string s = "123";
cout << MyInteger::parseInt(s) << endl;
return 0;
}
```
上面的代码中,我们先创建了一个 MyInteger 对象 a,并调用了它的一些成员函数。然后,我们调用了 MyInteger 类的一些静态函数。接着,我们创建了另一个 MyInteger 对象 b,并测试了 equals 函数。最后,我们测试了 parseInt 函数。
阅读全文
相关推荐
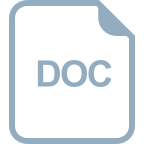
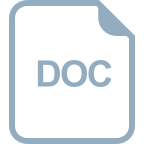
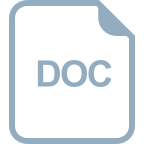


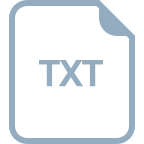
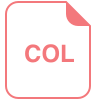
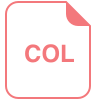
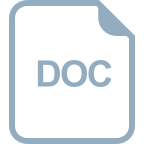
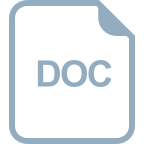
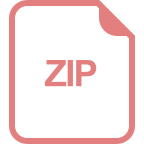
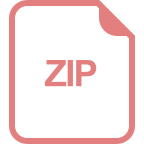
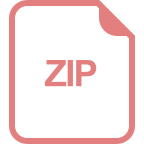
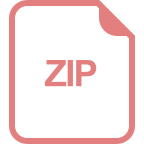