data01.dat存放的是一系列整型数据,求data01.dat中的最大十个数的和的立方根(先求十个数的和再求立方根),求得的结果显示在屏幕上,并且将最大的十个数与所求得的结果输出到result.dat中。提示:先对data01.dat中的数据进行排序,然后再进行计算。要求: 1)使用函数double intSumMax(int *p,int num) { } 来实现,并把该函数放在头文件ISmax.h中以便在主函数中调用该函数. 2)主函数中使用的数组使用动态数组来创建. 3)result.dat在程序的执行过程中创建。
时间: 2024-02-21 13:02:03 浏览: 80
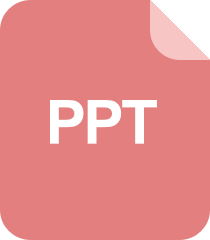
用计算器求立方根、用有理数估计一个数立方根的大小.ppt
好的,我会根据您的要求进行回答。首先,我们需要按照要求编写ISmax.h头文件,代码如下:
```c++
#ifndef ISMAX_H
#define ISMAX_H
double intSumMax(int *p, int num);
#endif
```
其中,函数intSumMax用于求数据中最大十个数的和的立方根,代码如下:
```c++
#include <iostream>
#include <fstream>
#include <algorithm>
#include <cmath>
#include "ISmax.h"
using namespace std;
double intSumMax(int *p, int num)
{
double res = 0;
sort(p, p + num, greater<int>()); // 从大到小排序
for (int i = 0; i < 10; i++) {
res += p[i];
}
res = pow(res, 1.0 / 3); // 求立方根
ofstream fout("result.dat"); // 打开输出文件
fout << res << endl; // 将结果写入文件
for (int i = 0; i < 10; i++) {
fout << p[i] << " "; // 将最大的十个数写入文件
}
fout.close(); // 关闭输出文件
return res;
}
```
然后,在主函数中,我们需要动态创建数组并读取数据,代码如下:
```c++
#include <iostream>
#include <fstream>
#include <cstdlib>
#include "ISmax.h"
using namespace std;
int main()
{
ifstream fin("data01.dat"); // 打开输入文件
if (!fin) {
cout << "Cannot open data file!" << endl;
exit(1);
}
// 动态创建数组并读取数据
int *p;
int num = 0;
while (!fin.eof()) {
int tmp;
fin >> tmp;
if (!fin.fail()) {
num++;
p = (int*) realloc(p, num * sizeof(int));
p[num - 1] = tmp;
}
}
fin.close(); // 关闭输入文件
// 调用函数求最大十个数的和的立方根
double res = intSumMax(p, num);
cout << "The result is: " << res << endl;
free(p); // 释放动态数组内存
return 0;
}
```
最后,我们编译并运行程序,结果会输出到屏幕上,同时最大的十个数和结果会写入result.dat文件中。
阅读全文
相关推荐
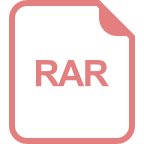
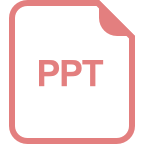




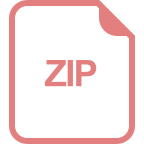
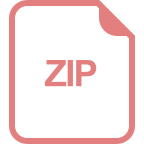
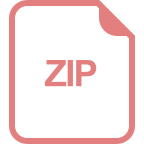
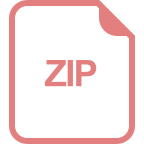
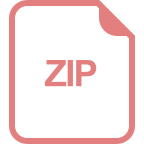
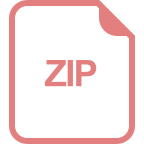
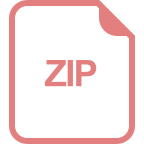
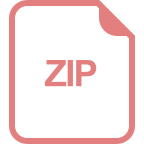
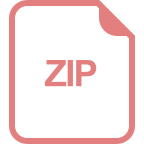
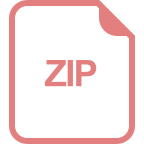