优化这段代码void student_information(struct stu **point) { //显示全部考生信息 FILE *fp; int i; struct stu *new; static struct stu *tail; if ((fp = fopen("C:/Users/MonnyX/Desktop/c/file2.dat", "r")) == NULL) { printf("打开文件失败!\n"); exit(0); } for (i = 1;i<=2; i++) { new = (struct stu *)malloc(sizeof(struct stu)); if (new == NULL) { printf("内存分配失败!\n"); exit(1); } fscanf(fp, "%d", &new->num); fscanf(fp, "%s", new->name); fscanf(fp, "%d", &new->age); fscanf(fp, "%s", new->sex); if (*point == NULL) { *point = new; new->next = NULL; } else { tail->next = new; new->next = NULL; } } tail = new; for(i=1;i<=2;i++){ printf("%d", new->num); printf("%s", new->name); printf("%d", new->age); printf("%s", new->sex); new=new->next; } fclose(fp); system("pause"); system("clear"); }
时间: 2023-06-14 09:06:23 浏览: 56
这段代码可以进行如下优化:
1. 将文件路径定义为常量或者宏,以便于修改和维护。
2. 将读取文件的代码封装成一个函数,可以复用。
3. 使用fgets()函数代替fscanf()函数,可以避免因为格式化字符串不匹配而导致的错误。
4. 将打印信息的代码封装成一个函数,可以复用。
5. 去掉不必要的变量和操作,简化代码结构。
6. 最好不要使用system()函数,因为它不可移植,可以使用跨平台的清屏函数。
下面是优化后的代码:
```
#define FILE_PATH "C:/Users/MonnyX/Desktop/c/file2.dat"
void read_student_information(struct stu **point) {
FILE *fp;
int i;
struct stu *new;
static struct stu *tail;
if ((fp = fopen(FILE_PATH, "r")) == NULL) {
printf("打开文件失败!\n");
exit(0);
}
for (i = 1; i <= 2; i++) {
new = (struct stu *)malloc(sizeof(struct stu));
if (new == NULL) {
printf("内存分配失败!\n");
exit(1);
}
char buf[1024];
fgets(buf, sizeof(buf), fp);
sscanf(buf, "%d %s %d %s", &new->num, new->name, &new->age, new->sex);
if (*point == NULL) {
*point = new;
new->next = NULL;
} else {
tail->next = new;
new->next = NULL;
}
tail = new;
}
fclose(fp);
}
void print_student_information(struct stu *point) {
while (point != NULL) {
printf("%d %s %d %s\n", point->num, point->name, point->age, point->sex);
point = point->next;
}
}
void student_information(struct stu **point) {
read_student_information(point);
print_student_information(*point);
clear_screen();
}
```
相关推荐
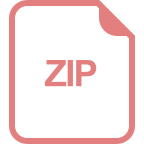
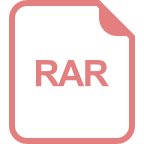
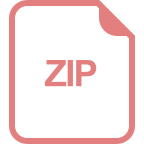















