#include<stdio.h> typdef struct SqStack{ int *base; int *top; int stacksize; }SqStack void InitStack(SqStack*s){ (*S).base=(int*)malloc(MAXSIZE*sizeof(int)); s->top=S->base; s->stacksize=MAXSIZE; } void Push(SqStack*S,int e){ *(S->top)=e; S->top++; } int Pop(SqStack*S,int e){ S->top=S->top-1; e=*(S->top); return e; } int StackEmpty(SqStack*S){ if(S->top==S->base) return 1; else return 0; } int main(){ int N; int e; SqStack S; InitStack(&S); printf("Please input N:"); scanf("%d",&N); while(N){ Push(&S,N%8); N=N/8; } while(!StackEmpty(&S)){ e=Pop(&S,e); printf("%d",e); } return 0; }改下bug
时间: 2024-03-11 12:50:43 浏览: 83
在函数Pop中,参数列表中不需要传入int e,因为这个变量是用来接收栈顶元素的,应该改为:
```c
int Pop(SqStack*S){
S->top=S->top-1;
int e=*(S->top);
return e;
}
```
另外,函数调用Pop(&S,e)中第二个参数e是多余的,应该改为:
```c
e = Pop(&S);
```
完整代码如下:
```c
#include<stdio.h>
#include<stdlib.h>
#define MAXSIZE 100
typedef struct SqStack{
int *base;
int *top;
int stacksize;
}SqStack;
void InitStack(SqStack*s){
(*s).base=(int*)malloc(MAXSIZE*sizeof(int));
s->top=s->base;
s->stacksize=MAXSIZE;
}
void Push(SqStack*S ,int e){
*(S->top)=e;
S->top++;
}
int Pop(SqStack*S){
S->top=S->top-1;
int e=*(S->top);
return e;
}
int StackEmpty(SqStack*S){
if(S->top==S->base)
return 1;
else
return 0;
}
int main(){
int N;
int e;
SqStack S;
InitStack(&S);
printf("Please input N:");
scanf("%d",&N);
while(N){
Push(&S,N%8);
N=N/8;
}
while(!StackEmpty(&S)){
e=Pop(&S);
printf("%d",e);
}
return 0;
}
```
阅读全文
相关推荐
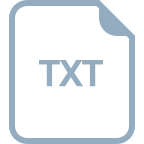
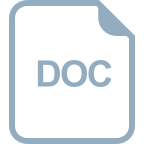
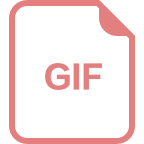
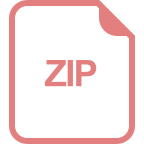
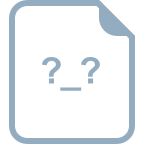
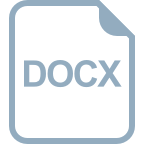
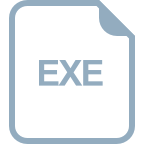
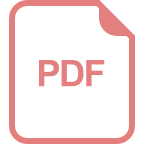







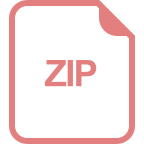
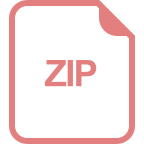