Python绘制圆指北针
时间: 2024-06-02 12:06:37 浏览: 192
Python有很多绘图库可以用来绘制图形,其中比较常用的是matplotlib。如果要绘制一个圆形指北针,可以按照以下步骤进行:
1. 导入绘图库matplotlib和numpy:
```python
import matplotlib.pyplot as plt
import numpy as np
```
2. 设置绘图区域大小、颜色和坐标轴范围:
```python
fig, ax = plt.subplots(figsize=(6,6))
ax.set_facecolor('xkcd:light blue')
ax.set_xlim(-1.2, 1.2)
ax.set_ylim(-1.2, 1.2)
```
3. 绘制圆形:
```python
circle = plt.Circle((0, 0), 1, color='w', fill=False, lw=3)
ax.add_artist(circle)
```
4. 绘制指针:
```python
angle = 45
pointer = np.radians(angle)
arrow_x = [0, 0.9 * np.cos(pointer)]
arrow_y = [0, 0.9 * np.sin(pointer)]
ax.plot(arrow_x, arrow_y, lw=5, color='r', zorder=10)
```
完整代码如下:
```python
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(figsize=(6,6))
ax.set_facecolor('xkcd:light blue')
ax.set_xlim(-1.2, 1.2)
ax.set_ylim(-1.2, 1.2)
circle = plt.Circle((0, 0), 1, color='w', fill=False, lw=3)
ax.add_artist(circle)
angle = 45
pointer = np.radians(angle)
arrow_x = [0, 0.9 * np.cos(pointer)]
arrow_y = [0, 0.9 * np.sin(pointer)]
ax.plot(arrow_x, arrow_y, lw=5, color='r', zorder=10)
plt.show()
```
阅读全文
相关推荐
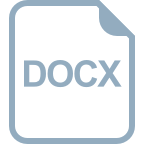

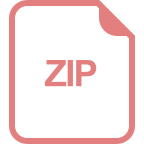
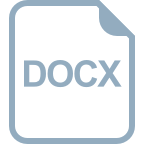
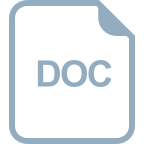
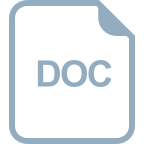
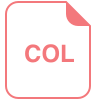
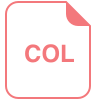
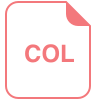
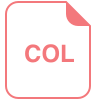
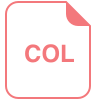
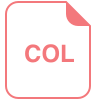
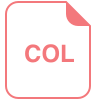
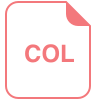

