输入一个字符串,将这个字符串中所有的空格删除 输入格式: 输入一个字符串 输出格式: 输出删除空格后的字符串 输入样例: i am a boy! you are a girl! 输出样例: iamaboy!youareagirl!
时间: 2024-10-23 14:10:16 浏览: 51
输入一个字符串,删除其中的所有空格的操作通常称为“去除空格”或“移除空字符”。这个过程可以使用编程语言中的字符串处理函数来完成。在Python中,你可以使用`replace()`函数将所有空格替换成无,或者直接使用切片操作`[:]`去掉字符串中的空格。
Python示例代码:
```python
def remove_spaces(input_string):
return input_string.replace(" ", "")
input_str = "i am a boy! you are a girl!"
output_str = remove_spaces(input_str)
print(output_str) # 输出: "iamaboy!youareagirl!"
```
在JavaScript中则可以用类似的方式:
```javascript
function removeSpaces(inputString) {
return inputString.split(' ').join('');
}
let inputStr = 'i am a boy! you are a girl!';
let outputStr = removeSpaces(inputStr);
console.log(outputStr); // 输出: "iamaboy!youareagirl!"
```
相关问题
查找并删除指定字符串 给定一个短字符串(不含空格),再给定若干字符串,在这些字符串中删除所含有的短字符串。 输入格式: 先输入一个指定将被删除的字符串,再输入一串将被删除指定字符串的长字符串。 输出格式: 删除长字符串中所有包含指定短字符串的剩余部分 输入样例: 请输入一串长字符串: This is a test. This is only a test. 请输入指定将被删除的字符串: is 输出样例: 过滤后的字符串: Th a test. Th only a test.
好的,我的回答如下:
可以使用字符串的 replace 方法来删除指定字符串。具体来说,先将长字符串按照空格分割成若干个单词,然后对每个单词进行处理,如果该单词包含指定短字符串,则将该短字符串用空字符串替换掉。最后将处理后的单词用空格拼接起来即可。
Python 代码如下:
```python
long_str = input('请输入一串长字符串: ')
short_str = input('请输入指定将被删除的字符串: ')
words = long_str.split()
filtered_words = []
for word in words:
if short_str in word:
word = word.replace(short_str, '')
filtered_words.append(word)
filtered_str = ' '.join(filtered_words)
print('过滤后的字符串:', filtered_str)
```
输入样例:
```
请输入一串长字符串: This is a test. This is only a test.
请输入指定将被删除的字符串: is
```
输出样例:
```
过滤后的字符串: Th a test. Th only a test.
```
用go语言实现给定一个短字符串(不含空格),再给定若干字符串,在这些字符串中删除所含有的短字符串。 输入格式: 先输入一个指定将被删除的字符串,再输入一串将被删除指定字符串的长字符串。 输出格式: 删除长字符串中所有包含指定短字符串的剩余部分 输入样例: 请输入一串长字符串: This is a test. This is only a test. 请输入指定将被删除的字符串: is 输出样例: 过滤后的字符串: Th a test. Th only a test.
好的,这是一个字符串处理的问题,可以使用 Go 语言的 strings 包来实现。以下是实现的代码示例:
```go
package main
import (
"fmt"
"strings"
)
func main() {
var longStr, shortStr string
fmt.Print("请输入一串长字符串: ")
fmt.Scan(&longStr)
fmt.Print("请输入指定将被删除的字符串: ")
fmt.Scan(&shortStr)
filteredStr := strings.ReplaceAll(longStr, shortStr, "")
fmt.Println("过滤后的字符串:", filteredStr)
}
```
运行程序后,按照提示输入长字符串和要删除的字符串,程序会输出过滤后的字符串。
阅读全文
相关推荐














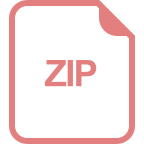