c++ map添加元素的方法
时间: 2023-10-31 19:55:37 浏览: 91
在 C++ 中,要向 `std::map` 添加元素,你可以使用 `insert` 函数或者使用下标操作符 `[]`。下面是两种方法的示例代码:
1. 使用 `insert` 函数:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
// 使用 insert 函数添加元素
myMap.insert(std::make_pair(1, "One"));
myMap.insert(std::make_pair(2, "Two"));
myMap.insert(std::make_pair(3, "Three"));
// 遍历 map 并打印元素
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
2. 使用下标操作符 `[]`:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
// 使用下标操作符添加元素
myMap[1] = "One";
myMap[2] = "Two";
myMap[3] = "Three";
// 遍历 map 并打印元素
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
无论你使用哪种方法,都可以成功向 `std::map` 添加元素。希望这能帮到你!如果你有更多问题,请继续提问。
阅读全文
相关推荐
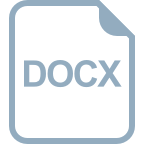
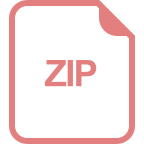
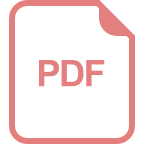










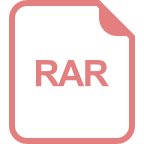



