求矩形和圆形的面积周长
时间: 2023-12-31 21:23:27 浏览: 32
以下是使用C#和Python分别求解矩形和圆形的面积和周长的例子:
C#代码:
```csharp
using System;
public abstract class Shape
{
public abstract double GetArea();
public abstract double GetPerimeter();
}
public class Rectangle : Shape
{
private double length;
private double width;
public Rectangle(double l, double w)
{
length = l;
width = w;
}
public override double GetArea()
{
return length * width;
}
public override double GetPerimeter()
{
return 2 * (length + width);
}
}
public class Circle : Shape
{
private double radius;
public Circle(double r)
{
radius = r;
}
public override double GetArea()
{
return Math.PI * radius * radius;
}
public override double GetPerimeter()
{
return 2 * Math.PI * radius;
}
}
class Program
{
static void Main(string[] args)
{
// 使用多态求矩形的面积和周长,求圆形的面积和周长
Shape shape = new Circle(5);
double area = shape.GetArea();
double perimeter = shape.GetPerimeter();
Console.WriteLine("圆形的周长是{0},面积是{1}", perimeter, area);
shape = new Rectangle(3, 4);
area = shape.GetArea();
perimeter = shape.GetPerimeter();
Console.WriteLine("矩形的周长是{0},面积是{1}", perimeter, area);
}
}
```
Python代码:
```python
import math
class Shape:
def get_area(self):
pass
def get_perimeter(self):
pass
class Rectangle(Shape):
def __init__(self, length, width):
self.length = length
self.width = width
def get_area(self):
return self.length * self.width
def get_perimeter(self):
return 2 * (self.length + self.width)
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def get_area(self):
return math.pi * self.radius * self.radius
def get_perimeter(self):
return 2 * math.pi * self.radius
# 使用多态求矩形的面积和周长,求圆形的面积和周长
shape = Circle(5)
area = shape.get_area()
perimeter = shape.get_perimeter()
print("圆形的周长是{0},面积是{1}".format(perimeter, area))
shape = Rectangle(3, 4)
area = shape.get_area()
perimeter = shape.get_perimeter()
print("矩形的周长是{0},面积是{1}".format(perimeter, area))
```
相关推荐
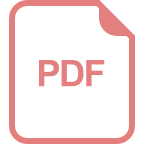














