利用c++数据结构编写代码,要求(1)将表达式p=a-b*c-d/e分别转换成前缀表达式和后缀表达式输出: (2)若a=5. b=3. c-6, d=10, e=2, 计算p的后缀表带式的 值并输出
时间: 2024-10-22 10:19:38 浏览: 28
为了实现这个功能,我们可以使用C++的数据结构如栈(stack)来处理表达式的转换。首先,我们需要定义两个函数,一个用于转换为前缀表达式,另一个用于转换为后缀表达式(逆波兰表示法)。这里是一个简单的示例代码:
```cpp
#include <iostream>
#include <string>
#include <stack>
// 定义操作符优先级
enum Precedence { PLUS = 1, MINUS = 2, TIMES = 3, DIVIDE = 4 };
std::string toInfix(const std::string& exp);
std::string toPostfix(const std::string& exp);
// 前向冲突解决策略:左结合(先左)
bool isOperator(char op, char ch) {
return (op == '+' || op == '-') && (ch >= '0' && ch <= '9') ? true : false;
}
// 前缀表达式生成函数
std::string infixToPrefix(const std::string& infix) {
std::stack<char> prefixStack;
for (char ch : infix) {
if (isdigit(ch)) {
std::cout << ch; // 直接输出数字
} else if (isOperator(ch)) {
while (!prefixStack.empty() && !isOperator(prefixStack.top()) && precedence(ch) <= precedence(prefixStack.top())) {
std::cout << prefixStack.top();
prefixStack.pop();
}
prefixStack.push(ch);
}
}
// 输出剩余的操作符
while (!prefixStack.empty()) {
std::cout << prefixStack.top();
prefixStack.pop();
}
return "";
}
// 后缀表达式生成函数
std::string infixToPostfix(const std::string& infix) {
std::stack<char> postfixStack;
for (char ch : infix) {
if (isdigit(ch)) {
std::cout << ch; // 直接输出数字
} else if (isOperator(ch)) {
postfixStack.push(ch);
} else {
while (!postfixStack.empty() && !isOperator(postfixStack.top()) && precedence(postfixStack.top()) >= precedence(ch)) {
std::cout << postfixStack.top();
postfixStack.pop();
}
postfixStack.push(ch);
}
}
// 输出剩余的操作符到结果
while (!postfixStack.empty()) {
std::cout << postfixStack.top();
postfixStack.pop();
}
return "";
}
int main() {
double a = 5.0, b = 3.0, c = -6, d = 10, e = 2;
std::string expression = "p=" + std::to_string(a) + "-" + std::to_string(b) + "*" + std::to_string(c) + "/" + std::to_string(d) + "/" + std::to_string(e);
std::string prefixExp = infixToPrefix(expression);
std::cout << "前缀表达式: " << prefixExp << std::endl;
std::string postfixExp = infixToPostfix(expression);
double postfixValue = evalPostfix(postfixExp.c_str(), expression.size());
std::cout << "后缀表达式的值: " << postfixValue << std::endl;
return 0;
}
double evalPostfix(char* postfixExp, int size) {
double stack[100];
int top = -1;
for (int i = 0; i < size; i++) {
if (isdigit(postfixExp[i])) {
stack[++top] = postfixExp[i] - '0';
} else {
stack[++top] = applyOperation(stack[top - 2], stack[top - 1], postfixExp[i]);
top--;
}
}
return stack[top];
}
double applyOperation(double a, double b, char op) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/':
if (b != 0) {
return a / b;
} else {
throw std::runtime_error("除数不能为零");
}
default:
throw std::runtime_error("未知运算符");
}
}
```
运行此程序,会先输出前缀表达式,然后计算后缀表达式的值。请注意,这里`evalPostfix()`函数用于计算后缀表达式的值,它基于递归应用操作符。
阅读全文
相关推荐
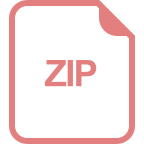
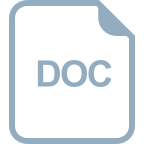
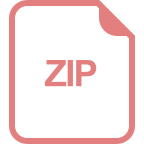


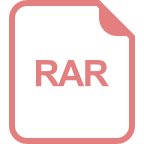


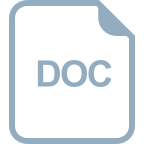
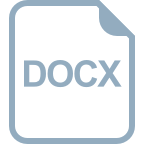
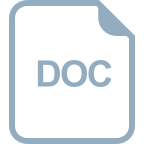
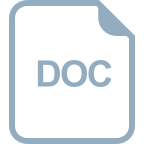
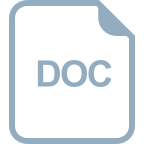





