C++将中缀表达式转化为前缀表达式并输出
时间: 2024-01-24 18:05:32 浏览: 90
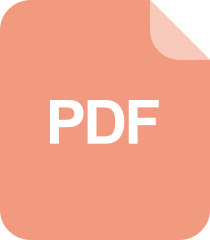
C++实现中缀表达式转后缀表达式

中缀表达式转前缀表达式的过程可以通过将中缀表达式反转,然后交换左右括号的位置,再使用后缀表达式的转换方法得到前缀表达式。具体实现可以使用栈来完成。
以下是C++代码实现:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
// 判断是否为操作符
bool isOperator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
// 判断操作符优先级
int getPriority(char op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
} else {
return 0;
}
}
// 中缀表达式转前缀表达式
string infixToPrefix(string infix) {
stack<char> s;
string prefix = "";
// 反转中缀表达式
reverse(infix.begin(), infix.end());
for (int i = 0; i < infix.length(); i++) {
char c = infix[i];
if (isOperator(c)) {
// 如果是操作符,弹出栈中优先级大于等于该操作符的元素
while (!s.empty() && getPriority(s.top()) >= getPriority(c)) {
prefix += s.top();
s.pop();
}
s.push(c);
} else if (c == ')') {
// 如果是右括号,直接入栈
s.push(c);
} else if (c == '(') {
// 如果是左括号,弹出栈中右括号前的元素
while (!s.empty() && s.top() != ')') {
prefix += s.top();
s.pop();
}
s.pop(); // 弹出右括号
} else {
// 如果是操作数,直接加入前缀表达式
prefix += c;
}
}
// 弹出栈中剩余元素
while (!s.empty()) {
prefix += s.top();
s.pop();
}
// 再次反转前缀表达式
reverse(prefix.begin(), prefix.end());
return prefix;
}
int main() {
string infix = "1+2*3-(4/5+6)*7";
string prefix = infixToPrefix(infix);
cout << "Infix expression: " << infix << endl;
cout << "Prefix expression: " << prefix << endl;
return 0;
}
```
阅读全文
相关推荐







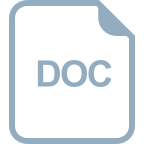
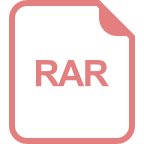
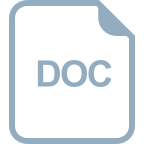





