Qt实现中缀表达式转为前缀表达式,中缀表达式中含有正整数,加减乘除,左右括号
时间: 2023-07-28 18:06:49 浏览: 100
要实现中缀表达式转为前缀表达式,可以采用以下步骤:
1. 将中缀表达式翻转,得到一个反向的表达式,这样左右括号的位置就会发生变化。
2. 将反向表达式中的左右括号互换,得到一个新的表达式。
3. 对新表达式进行前缀表达式的求解。
例如,将中缀表达式"5+(6-3)*2/3"转换为前缀表达式的过程如下:
1. 将中缀表达式翻转得到反向表达式"3/2*(3-6)+5"。
2. 将反向表达式中的左右括号互换得到新表达式"5+(6-3)*2/3".
3. 对新表达式进行前缀表达式的求解,得到前缀表达式"+ 5 / * - 6 3 2 3"。
下面是一个C++的实现代码:
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <algorithm>
using namespace std;
bool isOperator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
int priority(char op) {
if (op == '+' || op == '-') return 1;
if (op == '*' || op == '/') return 2;
return 0;
}
string infixToPrefix(string infix) {
reverse(infix.begin(), infix.end());
stack<char> s;
string prefix;
for (int i = 0; i < infix.length(); i++) {
char c = infix[i];
if (isOperator(c)) {
while (!s.empty() && priority(s.top()) > priority(c)) {
prefix += s.top();
s.pop();
}
s.push(c);
} else if (c == ')') {
s.push(c);
} else if (c == '(') {
while (!s.empty() && s.top() != ')') {
prefix += s.top();
s.pop();
}
s.pop();
} else {
prefix += c;
}
}
while (!s.empty()) {
prefix += s.top();
s.pop();
}
reverse(prefix.begin(), prefix.end());
return prefix;
}
int main() {
string infix = "5+(6-3)*2/3";
string prefix = infixToPrefix(infix);
cout << prefix << endl; // output: + 5 / * - 6 3 2 3
return 0;
}
```
阅读全文
相关推荐
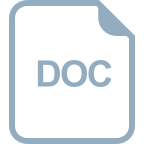
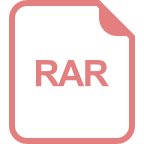
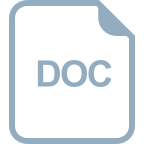













