中缀表达式转前缀表达式用Qt实现代码
时间: 2024-02-03 16:12:37 浏览: 64
以下是使用Qt实现中缀表达式转前缀表达式的代码:
```cpp
#include <QStack>
#include <QString>
QString infixToPrefix(QString infix)
{
QStack<QChar> operators;
QStack<QString> operands;
for(int i = 0; i < infix.length(); i++) {
QChar c = infix[i];
if(c == ' ' || c == '\t') {
continue;
} else if(c.isDigit() || c.isLetter()) {
QString operand;
while(i < infix.length() && (infix[i].isDigit() || infix[i].isLetter())) {
operand.append(infix[i++]);
}
operands.push(operand);
i--;
} else if(c == ')') {
operators.push(c);
} else if(c == '(') {
while(!operators.isEmpty() && operators.top() != ')') {
QString rightOperand = operands.pop();
QString leftOperand = operands.pop();
QChar op = operators.pop();
QString expression = op + leftOperand + rightOperand;
operands.push(expression);
}
operators.pop();
} else {
while(!operators.isEmpty() && precedence(c) <= precedence(operators.top())) {
QString rightOperand = operands.pop();
QString leftOperand = operands.pop();
QChar op = operators.pop();
QString expression = op + leftOperand + rightOperand;
operands.push(expression);
}
operators.push(c);
}
}
while(!operators.isEmpty()) {
QString rightOperand = operands.pop();
QString leftOperand = operands.pop();
QChar op = operators.pop();
QString expression = op + leftOperand + rightOperand;
operands.push(expression);
}
return operands.pop();
}
int precedence(QChar op)
{
switch(op.toLatin1()) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '^':
return 3;
default:
return 0;
}
}
```
该代码使用两个栈来实现中缀表达式转前缀表达式。`operators`栈用于存储运算符,`operands`栈用于存储操作数和子表达式。遍历中缀表达式时,如果当前字符是数字或字母,则将其解析为操作数并压入`operands`栈中;如果当前字符是右括号,则将其压入`operators`栈中;如果当前字符是左括号,则将`operators`栈中的运算符弹出,与`operands`栈中弹出的两个操作数构成一个子表达式,然后将该子表达式压入`operands`栈中,直到`operators`栈中弹出的运算符为左括号;如果当前字符是运算符,则将其与`operators`栈中的运算符比较,如果当前运算符的优先级小于等于`operators`栈顶运算符的优先级,则将`operators`栈中弹出的运算符与`operands`栈中弹出的两个操作数构成一个子表达式,然后将该子表达式压入`operands`栈中,直到`operators`栈为空或弹出的运算符的优先级大于当前运算符的优先级。最后,将`operands`栈中的元素合并成一个前缀表达式并返回。`precedence`函数用于获取运算符的优先级。
阅读全文
相关推荐
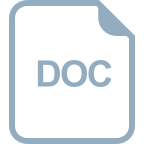
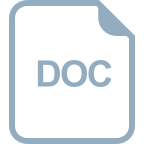
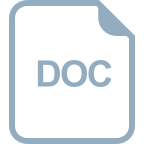












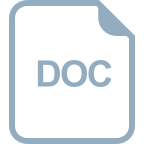


