pythontkinter贪吃蛇
时间: 2023-09-06 10:09:16 浏览: 130
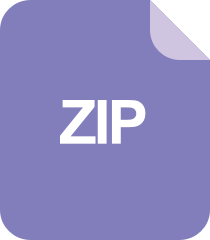
Python+tkinter制作的贪吃蛇小游戏
好的,你想学习如何用 Python 的 tkinter 模块制作贪吃蛇游戏是吗?下面是一个简单的教程,希望对你有帮助。
首先,你需要安装 Python 以及 tkinter 模块。在安装好之后,我们可以开始编写代码了。
1. 导入 tkinter 和 random 模块
```python
from tkinter import *
import random
```
2. 创建游戏窗口和画布
```python
# 创建窗口
window = Tk()
window.title("贪吃蛇")
# 创建画布
canvas = Canvas(window, width=500, height=500)
canvas.pack()
```
3. 定义贪吃蛇和食物
```python
class Snake:
def __init__(self):
self.body = [(250, 250), (240, 250), (230, 250)]
self.direction = "Right"
def move(self):
if self.direction == "Up":
head = (self.body[0][0], self.body[0][1] - 10)
elif self.direction == "Down":
head = (self.body[0][0], self.body[0][1] + 10)
elif self.direction == "Left":
head = (self.body[0][0] - 10, self.body[0][1])
else:
head = (self.body[0][0] + 10, self.body[0][1])
self.body.insert(0, head)
self.body.pop()
def change_direction(self, direction):
if direction == "Up" and self.direction != "Down":
self.direction = direction
elif direction == "Down" and self.direction != "Up":
self.direction = direction
elif direction == "Left" and self.direction != "Right":
self.direction = direction
elif direction == "Right" and self.direction != "Left":
self.direction = direction
def draw(self):
for body_part in self.body:
canvas.create_rectangle(body_part[0], body_part[1], body_part[0]+10, body_part[1]+10, fill="green")
class Food:
def __init__(self):
x = random.randint(0, 49) * 10
y = random.randint(0, 49) * 10
self.position = (x, y)
def draw(self):
canvas.create_rectangle(self.position[0], self.position[1], self.position[0]+10, self.position[1]+10, fill="red")
```
4. 定义游戏循环
```python
snake = Snake()
food = Food()
def game_loop():
canvas.delete(ALL)
# 绘制贪吃蛇和食物
snake.draw()
food.draw()
# 移动贪吃蛇
snake.move()
# 如果贪吃蛇吃到食物,就生成新的食物
if snake.body[0] == food.position:
food = Food()
window.after(100, game_loop)
game_loop()
```
5. 绑定键盘事件
```python
def on_key_press(event):
if event.keysym == "Up":
snake.change_direction("Up")
elif event.keysym == "Down":
snake.change_direction("Down")
elif event.keysym == "Left":
snake.change_direction("Left")
else:
snake.change_direction("Right")
window.bind("<KeyPress>", on_key_press)
```
6. 运行游戏
```python
window.mainloop()
```
好了,以上就是一个简单的用 tkinter 制作贪吃蛇游戏的教程。你可以按照上述步骤,自己动手写一下试试看。
阅读全文
相关推荐
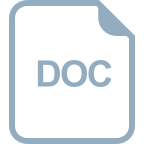
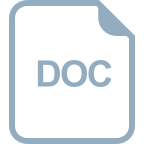
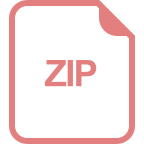

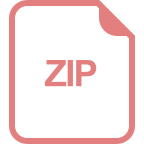
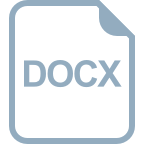
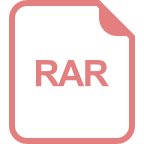
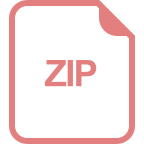
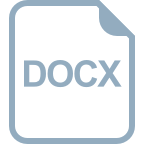
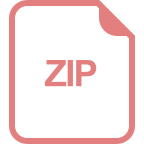
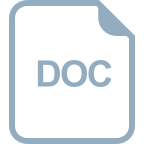




