用python运行贪吃蛇
时间: 2024-03-09 07:50:42 浏览: 19
你可以使用Python编写一个贪吃蛇游戏,以下是一个简单的示例代码:
```python
import tkinter as tk
import random
# 游戏设置
CELL_SIZE = 20
NUM_ROWS = 20
NUM_COLS = 20
SNAKE_INIT_LEN = 3
SNAKE_INIT_POS = (NUM_ROWS // 2, NUM_COLS // 2)
MOVE_INTERVAL = 200 # 毫秒
# 创建窗口
window = tk.Tk()
window.title('Snake')
# 创建游戏面板
board = tk.Canvas(window, width=NUM_COLS * CELL_SIZE, height=NUM_ROWS * CELL_SIZE, bg='white')
board.pack()
# 创建贪吃蛇
snake = [SNAKE_INIT_POS]
for i in range(SNAKE_INIT_LEN - 1):
snake.append((snake[-1][0], snake[-1][1] - 1))
snake_head = snake[-1]
snake_direction = 'right'
# 创建食物
food_pos = (random.randint(0, NUM_ROWS - 1), random.randint(0, NUM_COLS - 1))
food = board.create_rectangle(food_pos[1] * CELL_SIZE, food_pos[0] * CELL_SIZE,
(food_pos[1] + 1) * CELL_SIZE, (food_pos[0] + 1) * CELL_SIZE,
fill='red')
# 移动贪吃蛇
def move_snake():
global snake_head, snake_direction, food_pos, food
# 计算贪吃蛇头部的新位置
if snake_direction == 'up':
new_head = (snake_head[0] - 1, snake_head[1])
elif snake_direction == 'down':
new_head = (snake_head[0] + 1, snake_head[1])
elif snake_direction == 'left':
new_head = (snake_head[0], snake_head[1] - 1)
elif snake_direction == 'right':
new_head = (snake_head[0], snake_head[1] + 1)
# 如果贪吃蛇头部碰到边界或自己的身体,则游戏结束
if new_head[0] < 0 or new_head[0] >= NUM_ROWS or new_head[1] < 0 or new_head[1] >= NUM_COLS or new_head in snake:
tk.messagebox.showerror('Game Over', 'You hit the wall or the snake body!')
window.destroy()
return
snake_head = new_head
snake.append(snake_head)
# 如果贪吃蛇头部碰到食物,则增加贪吃蛇的长度并重新生成食物
if snake_head == food_pos:
board.delete(food)
food_pos = (random.randint(0, NUM_ROWS - 1), random.randint(0, NUM_COLS - 1))
food = board.create_rectangle(food_pos[1] * CELL_SIZE, food_pos[0] * CELL_SIZE,
(food_pos[1] + 1) * CELL_SIZE, (food_pos[0] + 1) * CELL_SIZE,
fill='red')
else:
snake.pop(0)
# 更新贪吃蛇的位置
board.delete('snake')
for pos in snake:
board.create_rectangle(pos[1] * CELL_SIZE, pos[0] * CELL_SIZE,
(pos[1] + 1) * CELL_SIZE, (pos[0] + 1) * CELL_SIZE,
fill='green', tags='snake')
# 设置下一次移动
window.after(MOVE_INTERVAL, move_snake)
# 按键事件
def key_pressed(event):
global snake_direction
if event.keysym == 'Up' and snake_direction != 'down':
snake_direction = 'up'
elif event.keysym == 'Down' and snake_direction != 'up':
snake_direction = 'down'
elif event.keysym == 'Left' and snake_direction != 'right':
snake_direction = 'left'
elif event.keysym == 'Right' and snake_direction != 'left':
snake_direction = 'right'
# 绑定按键事件
window.bind('<KeyPress>', key_pressed)
# 开始游戏
move_snake()
window.mainloop()
```
这个示例代码创建了一个20x20的贪吃蛇游戏,其中贪吃蛇初始长度为3,每次移动的时间间隔为200毫秒。玩家可以使用方向键来控制贪吃蛇的移动方向,并尝试吃掉食物以增加贪吃蛇的长度。如果贪吃蛇碰到边界或自己的身体,则游戏结束。
请注意,这只是一个简单的示例,实际上你可以根据自己的需求来修改和扩展这个代码。
相关推荐
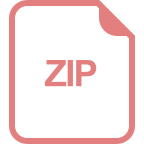
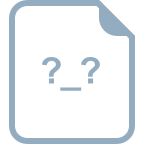
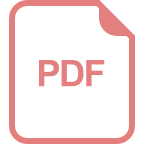














