用python实现贪吃蛇小游戏
时间: 2023-03-26 17:01:37 浏览: 162
好的,以下是用Python实现贪吃蛇小游戏的基本步骤:
1.导入必要的模块:需要使用Pygame模块来创建游戏窗口、处理用户输入、绘制游戏元素等。
2.初始化游戏:创建游戏窗口、初始化游戏元素(如蛇的初始位置、食物的初始位置、分数等),设置游戏运行状态等。
3.游戏循环:在游戏循环中,处理用户输入、更新游戏状态、绘制游戏元素等,直到游戏结束。
4.处理用户输入:监听用户输入,根据用户输入来更新蛇的方向。
5.更新游戏状态:根据当前蛇的方向移动蛇的位置,检查是否吃到了食物、是否撞到了墙壁或自身等,更新分数、增加蛇的长度等。
6.绘制游戏元素:根据游戏状态,绘制蛇、食物、分数等游戏元素。
7.游戏结束:当游戏结束时,显示游戏结束信息,等待用户操作(如重新开始游戏或退出游戏)。
具体实现方式可以参考相关教程或代码示例。
相关问题
用Python实现贪吃蛇小游戏
好的,以下是用Python实现贪吃蛇小游戏的代码:
```python
import pygame
import random
# 初始化
pygame.init()
# 窗口大小
win_width = 500
win_height = 500
# 创建窗口
win = pygame.display.set_mode((win_width, win_height))
pygame.display.set_caption("贪吃蛇")
# 颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
# 蛇的属性
snake_block = 10
snake_speed = 15
# 字体
font = pygame.font.SysFont(None, 25)
# 显示得分
def show_score(score):
score_text = font.render("Score: " + str(score), True, white)
win.blit(score_text, [0, 0])
# 画蛇
def draw_snake(snake_block, snake_list):
for x in snake_list:
pygame.draw.rect(win, black, [x[0], x[1], snake_block, snake_block])
# 消息提示
def message(msg, color):
message_text = font.render(msg, True, color)
win.blit(message_text, [win_width/6, win_height/3])
# 游戏循环
def gameLoop():
game_over = False
game_close = False
# 初始位置
x1 = win_width / 2
y1 = win_height / 2
# 移动位置
x1_change = 0
y1_change = 0
# 蛇的长度
snake_List = []
Length_of_snake = 1
# 食物的位置
foodx = round(random.randrange(0, win_width - snake_block) / 10.0) * 10.0
foody = round(random.randrange(0, win_height - snake_block) / 10.0) * 10.0
# 游戏循环
while not game_over:
while game_close == True:
win.fill(black)
message("You Lost! Press Q-Quit or C-Play Again", red)
show_score(Length_of_snake - 1)
pygame.display.update()
# 游戏结束
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
game_over = True
game_close = False
if event.key == pygame.K_c:
gameLoop()
# 移动蛇
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
x1_change = -snake_block
y1_change = 0
elif event.key == pygame.K_RIGHT:
x1_change = snake_block
y1_change = 0
elif event.key == pygame.K_UP:
y1_change = -snake_block
x1_change = 0
elif event.key == pygame.K_DOWN:
y1_change = snake_block
x1_change = 0
# 超出边界
if x1 >= win_width or x1 < 0 or y1 >= win_height or y1 < 0:
game_close = True
# 移动位置
x1 += x1_change
y1 += y1_change
# 画食物
win.fill(black)
pygame.draw.rect(win, red, [foodx, foody, snake_block, snake_block])
# 蛇的长度
snake_Head = []
snake_Head.append(x1)
snake_Head.append(y1)
snake_List.append(snake_Head)
if len(snake_List) > Length_of_snake:
del snake_List[0]
# 撞到身体
for x in snake_List[:-1]:
if x == snake_Head:
game_close = True
# 画蛇
draw_snake(snake_block, snake_List)
show_score(Length_of_snake - 1)
pygame.display.update()
# 吃到食物
if x1 == foodx and y1 == foody:
foodx = round(random.randrange(0, win_width - snake_block) / 10.0) * 10.0
foody = round(random.randrange(0, win_height - snake_block) / 10.0) * 10.0
Length_of_snake += 1
# 刷新速度
pygame.time.delay(snake_speed)
# 退出游戏
pygame.quit()
quit()
# 运行游戏
gameLoop()
```
这段代码实现了一个简单的贪吃蛇小游戏,可以在Python编译器中直接运行。
使用python编写贪吃蛇小游戏
好的,下面是一个简单的贪吃蛇小游戏的代码实现:
```python
import pygame
import random
# 定义常量
WIDTH = 400
HEIGHT = 400
BLOCK_SIZE = 20
# 初始化pygame
pygame.init()
# 创建游戏窗口
window = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("贪吃蛇")
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义字体
FONT = pygame.font.SysFont(None, 30)
# 定义Snake类
class Snake:
def __init__(self):
self.body = [(WIDTH // 2, HEIGHT // 2)]
self.direction = random.choice(["left", "right", "up", "down"])
self.score = 0
def move(self):
if self.direction == "left":
new_head = (self.body[0][0] - BLOCK_SIZE, self.body[0][1])
elif self.direction == "right":
new_head = (self.body[0][0] + BLOCK_SIZE, self.body[0][1])
elif self.direction == "up":
new_head = (self.body[0][0], self.body[0][1] - BLOCK_SIZE)
elif self.direction == "down":
new_head = (self.body[0][0], self.body[0][1] + BLOCK_SIZE)
self.body.insert(0, new_head)
self.body.pop()
def change_direction(self, new_direction):
if new_direction == "left" and self.direction != "right":
self.direction = new_direction
elif new_direction == "right" and self.direction != "left":
self.direction = new_direction
elif new_direction == "up" and self.direction != "down":
self.direction = new_direction
elif new_direction == "down" and self.direction != "up":
self.direction = new_direction
def draw(self):
for block in self.body:
pygame.draw.rect(window, GREEN, (block[0], block[1], BLOCK_SIZE, BLOCK_SIZE))
def eat(self, food):
if self.body[0] == food:
self.body.append(self.body[-1])
self.score += 1
return True
return False
# 定义Food类
class Food:
def __init__(self):
self.position = (random.randint(0, WIDTH // BLOCK_SIZE - 1) * BLOCK_SIZE,
random.randint(0, HEIGHT // BLOCK_SIZE - 1) * BLOCK_SIZE)
def draw(self):
pygame.draw.rect(window, RED, (self.position[0], self.position[1], BLOCK_SIZE, BLOCK_SIZE))
# 创建Snake和Food对象
snake = Snake()
food = Food()
# 定义游戏主循环
clock = pygame.time.Clock()
game_over = False
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
snake.change_direction("left")
elif event.key == pygame.K_RIGHT:
snake.change_direction("right")
elif event.key == pygame.K_UP:
snake.change_direction("up")
elif event.key == pygame.K_DOWN:
snake.change_direction("down")
# 移动Snake
snake.move()
# 判断是否吃到Food
if snake.eat(food.position):
food = Food()
# 绘制游戏界面
window.fill(BLACK)
snake.draw()
food.draw()
score_text = FONT.render("Score: {}".format(snake.score), True, WHITE)
window.blit(score_text, (10, 10))
pygame.display.update()
# 判断是否碰到边界或自身
if snake.body[0][0] < 0 or snake.body[0][0] >= WIDTH or snake.body[0][1] < 0 or snake.body[0][1] >= HEIGHT:
game_over = True
for block in snake.body[1:]:
if block == snake.body[0]:
game_over = True
# 控制游戏速度
clock.tick(10)
# 退出游戏
pygame.quit()
```
运行以上代码,即可开始游戏。玩家可以使用键盘上的方向键来控制贪吃蛇的移动方向,吃到食物后,贪吃蛇的长度会增加,得分也会增加。如果贪吃蛇碰到边界或自身,则游戏结束。
阅读全文
相关推荐
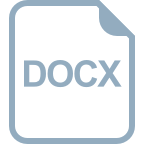
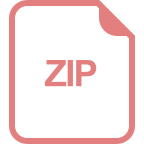
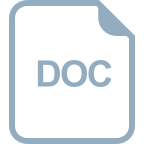
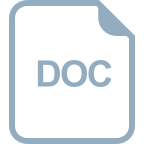
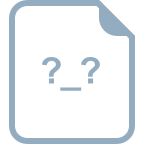
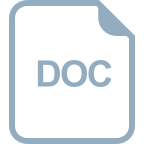

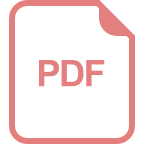
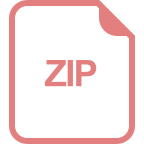