CSHarp从INI文件里读取每行数据存在数据结构里
时间: 2024-05-15 19:16:30 浏览: 15
这里提供一个使用C#读取INI文件并将每行数据存储到数据结构中的示例代码:
1. 首先,需要导入System.IO和System.Collections.Generic命名空间:
```
using System.IO;
using System.Collections.Generic;
```
2. 创建一个结构体来存储INI文件中每行数据的键值对:
```
struct KeyValue
{
public string Key;
public string Value;
}
```
3. 创建一个函数来读取INI文件中的每行数据,并将其存储到List<KeyValue>中:
```
static List<KeyValue> ReadINIFile(string filePath)
{
List<KeyValue> keyValueList = new List<KeyValue>();
if (File.Exists(filePath))
{
using (StreamReader reader = new StreamReader(filePath))
{
string line;
while ((line = reader.ReadLine()) != null)
{
line = line.Trim();
if (line.StartsWith(";") || line.StartsWith("#") || line.Length == 0)
{
// Skip comments and empty lines
continue;
}
int separatorIndex = line.IndexOf("=");
if (separatorIndex == -1)
{
// Invalid line format, skip it
continue;
}
string key = line.Substring(0, separatorIndex).Trim();
string value = line.Substring(separatorIndex + 1).Trim();
keyValueList.Add(new KeyValue { Key = key, Value = value });
}
}
}
return keyValueList;
}
```
4. 调用ReadINIFile函数并打印读取到的数据:
```
List<KeyValue> keyValueList = ReadINIFile("example.ini");
foreach (KeyValue keyValue in keyValueList)
{
Console.WriteLine("Key: {0}, Value: {1}", keyValue.Key, keyValue.Value);
}
```
这样就可以读取INI文件中的每行数据,并将其存储到List<KeyValue>中。可以根据需要修改KeyValue结构体的定义,以及ReadINIFile函数中的处理逻辑,来适应不同的INI文件格式。
相关推荐
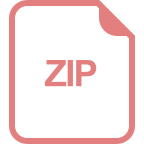
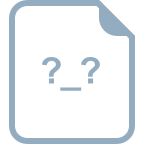














