题目描述 小蓝给学生们组织了一场考试,卷面总分为 100 分,每个学生的得分都是一个 0 到 100 的整数。 请计算这次考试的最高分、最低分和平均分。 输入描述 输入的第一行包含一个整数 � ( 1 ≤ � ≤ 1 0 4 ) n (1≤n≤10 4 ),表示考试人数。 接下来 � n 行,每行包含一个 0 至 100 的整数,表示一个学生的得分。 输出描述 输出三行。 第一行包含一个整数,表示最高分。 第二行包含一个整数,表示最低分。 第三行包含一个实数,四舍五入保留正好两位小数,表示平均分
时间: 2023-03-02 22:40:41 浏览: 213
题目描述:
小蓝给学生们组织了一场考试,卷面总分为100分,每个学生的得分都是一个0到100的整数。请计算这次考试的最高分、最低分和平均分。
输入描述:
输入的第一行包含一个整数n(1 ≤ n ≤ 10^4),表示考试人数。接下来n行,每行包含一个0至100的整数,表示一个学生的得分。
输出描述:
输出三行。第一行包含一个整数,表示最高分。第二行包含一个整数,表示最低分。第三行包含一个实数,四舍五入保留正好两位小数,表示平均分。
示例:
输入:
5
90
78
85
99
60
输出:
99
60
82.40
解释:
输入数据共有5个学生的成绩,分别为90、78、85、99、60。最高分为99,最低分为60,平均分为(90+78+85+99+60)/5=82.40。
相关问题
小蓝给学生们组织了一场考试,卷面总分为100分,每个学生的得分都是一个0到100的整数 请计算这次考试的最高分、最低分和平均分。用JAVA代码写
### 回答1:
最高分 = 100分
最低分 = 0分
平均分 = 所有学生得分之和 / 学生人数
以下是使用JAVA代码实现计算最高分、最低分和平均分的方法:
```java
public class Exam {
public static void main(String[] args) {
int[] scores = { 85, 92, 76, 99, 64, 80, 90, 88, 70, 78 };
int sum = 0;
int max = scores[0];
int min = scores[0];
for (int i = 0; i < scores.length; i++) {
sum += scores[i];
if (scores[i] > max) {
max = scores[i];
}
if (scores[i] < min) {
min = scores[i];
}
}
double avg = (double) sum / scores.length;
System.out.println("最高分:" + max);
System.out.println("最低分:" + min);
System.out.println("平均分:" + avg);
}
}
```
假设这场考试有10名学生,他们的得分分别是85分、92分、76分、99分、64分、80分、90分、88分、70分和78分。代码中定义了一个整型数组来存储学生的得分,然后使用for循环遍历数组,计算总分、最高分和最低分。最后,通过将总分除以学生人数,得到平均分,并将三个结果输出到控制台。
### 回答2:
import java.util.Scanner;
public class ExamScores {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int numStudents;
int maxScore = 0;
int minScore = 100;
int totalScore = 0;
System.out.print("请输入学生人数:");
numStudents = sc.nextInt();
for (int i = 1; i <= numStudents; i++) {
System.out.print("请输入第" + i + "个学生的分数:");
int score = sc.nextInt();
if (score > maxScore) {
maxScore = score;
}
if (score < minScore) {
minScore = score;
}
totalScore += score;
}
double averageScore = (double) totalScore / numStudents;
System.out.println("最高分:" + maxScore);
System.out.println("最低分:" + minScore);
System.out.println("平均分:" + averageScore);
sc.close();
}
}
### 回答3:
可以使用Java代码来计算这次考试的最高分、最低分和平均分。
首先,需要声明一个包含学生得分的整数数组,然后根据数组元素来计算最高分、最低分和平均分。
```java
public class ExamScore {
public static void main(String[] args) {
int[] scores = {85, 92, 78, 96, 88}; // 假设有5个学生的得分
int maxScore = scores[0]; // 假设第一个学生的得分为最高分
int minScore = scores[0]; // 假设第一个学生的得分为最低分
int sum = scores[0]; // 假设第一个学生的得分为总分
for (int i = 1; i < scores.length; i++) {
if (scores[i] > maxScore) {
maxScore = scores[i]; // 更新最高分
}
if (scores[i] < minScore) {
minScore = scores[i]; // 更新最低分
}
sum += scores[i]; // 计算总分
}
double averageScore = (double) sum / scores.length; // 计算平均分
System.out.println("最高分:" + maxScore);
System.out.println("最低分:" + minScore);
System.out.println("平均分:" + averageScore);
}
}
```
以上代码中,我们使用一个for循环来遍历学生得分数组,逐个比较得分并更新最高分和最低分。同时,计算总分的变量sum也会逐步增加每个学生的得分。最后,通过总分除以学生数量来计算平均分。
运行代码后,将输出最高分、最低分和平均分的结果。
用java代码写小蓝给学生们组织了一场考试,卷面总分为 100 分,每个学生的得分都是一个 0 到 100 的整数。 如果得分至少是 60 分,则称为及格。 如果得分至少为 85 分,则称为优秀。 请计算及格率和优秀率,用百分数表示,百分号前的部分四舍五入保留整数。
好的,以下是 Java 代码实现:
```java
import java.text.DecimalFormat;
import java.util.Scanner;
public class ExamScore {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int n = input.nextInt();
int[] scores = new int[n];
int passCount = 0, excellentCount = 0;
for (int i = 0; i < n; i++) {
scores[i] = input.nextInt();
if (scores[i] >= 60) {
passCount++;
}
if (scores[i] >= 85) {
excellentCount++;
}
}
DecimalFormat decimalFormat = new DecimalFormat("0");
System.out.println(decimalFormat.format((double) passCount / n * 100) + "%");
System.out.println(decimalFormat.format((double) excellentCount / n * 100) + "%");
}
}
```
这段代码首先读取输入的学生人数 `n`,然后读取 `n` 个学生的得分并保存到数组 `scores` 中。同时统计及格人数 `passCount` 和优秀人数 `excellentCount`,最后根据公式计算及格率和优秀率,并使用 `DecimalFormat` 将结果四舍五入保留整数后输出。
阅读全文
相关推荐
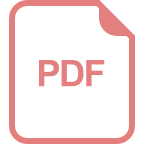
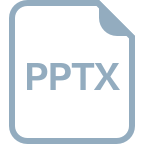













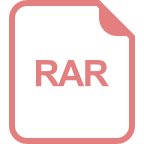
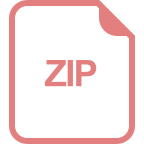