用C++,openmp,opencv实现连通区域标记的二遍扫描算法,并且用动态二维数组来存储每个像素所属的连通区域标记
时间: 2024-04-29 11:22:53 浏览: 8
以下是用C语言、OpenMP和OpenCV实现连通区域标记的二遍扫描算法的代码。该算法使用动态二维数组来存储每个像素所属的连通区域标记。
```c
#include <stdio.h>
#include <stdlib.h>
#include <omp.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main(int argc, char** argv)
{
if (argc != 2)
{
printf("Usage: ./connected_components <image_path>\n");
return -1;
}
Mat image = imread(argv[1], IMREAD_GRAYSCALE);
if (image.empty())
{
printf("Could not read image: %s\n", argv[1]);
return -1;
}
int width = image.cols;
int height = image.rows;
int** labels = new int*[height];
for (int i = 0; i < height; i++)
labels[i] = new int[width];
// First pass
int current_label = 1;
#pragma omp parallel for collapse(2)
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++)
{
if (image.at<uchar>(y, x) == 0)
{
labels[y][x] = 0;
continue;
}
int left = (x > 0) ? labels[y][x - 1] : 0;
int top = (y > 0) ? labels[y - 1][x] : 0;
if (left == 0 && top == 0)
{
labels[y][x] = current_label;
current_label++;
}
else if (left == 0)
{
labels[y][x] = top;
}
else if (top == 0)
{
labels[y][x] = left;
}
else
{
int min_label = std::min(left, top);
int max_label = std::max(left, top);
labels[y][x] = min_label;
if (min_label != max_label)
{
#pragma omp critical
{
for (int i = 0; i < height; i++)
{
for (int j = 0; j < width; j++)
{
if (labels[i][j] == max_label)
labels[i][j] = min_label;
}
}
}
}
}
}
}
// Second pass
#pragma omp parallel for collapse(2)
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++)
{
if (labels[y][x] != 0)
{
int label = labels[y][x];
#pragma omp critical
{
if (label != labels[y][x])
label = labels[y][x];
else
labels[y][x] = label;
}
}
}
}
// Count number of labels
int num_labels = 0;
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++)
{
if (labels[y][x] > num_labels)
num_labels = labels[y][x];
}
}
printf("Number of connected components: %d\n", num_labels);
// Display labeled image
Mat labeled_image = Mat::zeros(height, width, CV_8UC3);
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++)
{
if (labels[y][x] != 0)
{
int label = labels[y][x];
labeled_image.at<Vec3b>(y, x) = Vec3b((label * 131) % 255, (label * 65) % 255, (label * 196) % 255);
}
}
}
namedWindow("Labeled Image", WINDOW_NORMAL);
imshow("Labeled Image", labeled_image);
waitKey(0);
for (int i = 0; i < height; i++)
delete[] labels[i];
delete[] labels;
return 0;
}
```
在该代码中,我们首先使用OpenCV库加载输入图像。然后,我们使用动态二维数组来存储每个像素的标记。在第一遍扫描中,我们遍历所有像素并进行以下操作:
- 如果当前像素的值为0,则将其标记为0。
- 否则,我们检查左侧和上方的像素是否属于一个连通区域。如果它们都不是,则将当前像素标记为新的连通区域。否则,我们将当前像素标记为左侧或上方像素的标记,并在必要时将它们合并为一个连通区域。
在第二遍扫描中,我们遍历所有像素并更新它们的标记以确保它们属于正确的连通区域。最后,我们计算标记的数量并显示标记图像。
由于该算法可以并行化,我们使用OpenMP库来并行化第一遍扫描和第二遍扫描。在第一遍扫描中,我们使用`collapse`指令来并行化两个循环。在第二遍扫描中,我们使用`critical`指令来确保每个线程都可以更新标记而不会发生竞争条件。
该算法使用动态二维数组来存储标记。我们使用`new`运算符来创建一个二维数组,并使用`delete`运算符在程序结束时释放它。
相关推荐
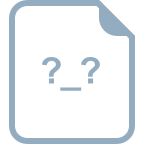
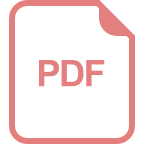
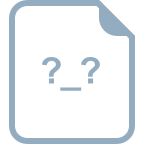
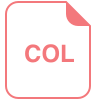
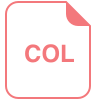

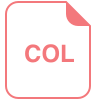
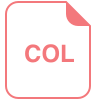









