【Clion配置OpenCV:从入门到精通】:打造你的高效C++开发环境
发布时间: 2024-08-09 01:55:23 阅读量: 346 订阅数: 39 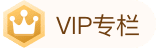
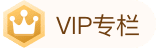

# 1. OpenCV简介**
OpenCV(Open Source Computer Vision Library)是一个开源计算机视觉库,提供广泛的图像处理和计算机视觉算法。它被广泛用于各种应用中,包括图像处理、视频分析、人脸识别和机器人技术。OpenCV具有跨平台兼容性,可在Windows、Linux和Mac OS X等多个平台上运行。
OpenCV提供了多种功能,包括图像读取和显示、图像转换和增强、图像分割、特征提取、图像识别、物体检测、人脸识别和视频处理。这些功能使开发人员能够轻松构建强大的计算机视觉应用程序。
# 2. Clion配置OpenCV
### 2.1 安装OpenCV
#### 安装步骤
1. **下载OpenCV库:**从OpenCV官方网站下载适用于您操作系统和Clion版本的OpenCV库。
2. **解压库:**将下载的库解压到您选择的目录中。
3. **设置环境变量:**在系统环境变量中添加以下变量:
- `OPENCV_DIR`:指向解压后的OpenCV库目录
- `PATH`:将`OPENCV_DIR/bin`添加到`PATH`变量中
### 2.2 配置Clion
#### 设置项目
1. **创建新项目:**在Clion中创建一个新的C++项目。
2. **添加OpenCV库:**右键单击项目文件,选择"Project Structure"。在"Libraries"选项卡中,单击"Add"按钮并选择"From File"。浏览并选择OpenCV库的`.dll`或`.so`文件。
#### 设置编译器和链接器
1. **设置编译器选项:**在"Project Structure"窗口中,转到"C/C++ Compiler"选项卡。在"Additional Include Directories"字段中,添加OpenCV库的include目录(例如,`OPENCV_DIR/include`)。
2. **设置链接器选项:**在"Project Structure"窗口中,转到"Linker"选项卡。在"Additional Library Directories"字段中,添加OpenCV库的lib目录(例如,`OPENCV_DIR/lib`)。
3. **添加链接库:**在"Linker"选项卡中,在"Libraries"字段中添加OpenCV库的名称(例如,`opencv_core`、`opencv_imgproc`)。
### 2.3 编写第一个OpenCV程序
#### 创建主函数
```cpp
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
// ...
}
```
#### 读取图像
```cpp
Mat image = imread("image.jpg");
```
#### 显示图像
```cpp
imshow("Image", image);
waitKey(0);
```
#### 代码逻辑分析
- `imread`函数读取指定路径的图像并将其存储在`Mat`对象中。
- `imshow`函数创建一个窗口并显示`Mat`对象中的图像。
- `waitKey`函数等待用户按下任意键,然后继续执行程序。
#### 参数说明
- `imread`函数:
- `filename`:要读取的图像文件的路径。
- `imshow`函数:
- `windowName`:窗口的名称。
- `image`:要显示的`Mat`对象。
- `waitKey`函数:
- `delay`:等待用户输入的毫秒数(0表示无限等待)。
# 3. OpenCV基础
### 3.1 图像处理基础
图像处理是OpenCV的核心功能之一。它涉及对图像进行各种操作,以增强其视觉效果或提取有价值的信息。OpenCV提供了广泛的图像处理函数,可用于执行各种任务,包括:
- **图像转换:**将图像从一种格式转换为另一种格式,例如从RGB到灰度。
- **图像增强:**改善图像的视觉质量,例如调整对比度或亮度。
- **图像滤波:**使用卷积核对图像进行滤波,以平滑噪声或增强边缘。
- **图像形态学:**使用结构元素对图像进行形态学操作,例如膨胀或腐蚀。
### 3.2 图像读取和显示
在OpenCV中,可以使用`cv2.imread()`函数读取图像。该函数接受图像文件的路径并返回一个NumPy数组,其中包含图像数据。图像数据是一个三维数组,其中第一维表示高度,第二维表示宽度,第三维表示通道数(对于RGB图像为3)。
读取图像后,可以使用`cv2.imshow()`函数显示图像。该函数接受图像数组和窗口名称作为参数,并在窗口中显示图像。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 显示图像
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 3.3 图像转换和增强
OpenCV提供了多种函数来转换和增强图像。以下是一些常用的函数:
- **cv2.cvtColor():**将图像从一种颜色空间转换为另一种颜色空间。
- **cv2.resize():**调整图像的大小。
- **cv2.flip():**翻转图像。
- **cv2.rotate():**旋转图像。
- **cv2.equalizeHist():**均衡图像的直方图。
- **cv2.blur():**对图像进行高斯模糊。
- **cv2.Canny():**检测图像中的边缘。
```python
import cv2
# 将图像转换为灰度
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 调整图像大小
resized_image = cv2.resize(image, (500, 500))
# 翻转图像
flipped_image = cv2.flip(image, 1)
# 显示转换后的图像
cv2.imshow('Gray Image', gray_image)
cv2.imshow('Resized Image', resized_image)
cv2.imshow('Flipped Image', flipped_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
# 4. OpenCV图像处理
### 4.1 图像分割
图像分割是将图像分解为具有不同特征(如颜色、纹理或形状)的区域的过程。在OpenCV中,有许多用于图像分割的算法,包括:
- **阈值分割:**将像素分类为前景或背景,基于其灰度值与阈值之间的关系。
```cpp
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 应用阈值分割
thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)[1]
# 显示分割后的图像
cv2.imshow('Thresholded Image', thresh)
cv2.waitKey(0)
```
- **区域增长:**从种子点开始,将具有相似特征的相邻像素分组到同一区域中。
```cpp
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 定义种子点
seed = (100, 100)
# 应用区域增长分割
segmented = cv2.watershed(gray, np.zeros((gray.shape[0], gray.shape[1]), dtype=np.uint8), seed)
# 显示分割后的图像
cv2.imshow('Segmented Image', segmented)
cv2.waitKey(0)
```
- **聚类:**将像素聚类到基于相似性度量的不同组中。
```cpp
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 重新整形为一维数组
pixels = gray.reshape((-1, 1))
# 应用 K-Means 聚类
kmeans = cv2.kmeans(pixels, 3, None, (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 10, 1.0))
# 获取聚类标签
labels = kmeans[1].reshape(gray.shape)
# 显示分割后的图像
cv2.imshow('Segmented Image', labels)
cv2.waitKey(0)
```
### 4.2 特征提取
特征提取是识别图像中重要特征的过程,这些特征可以用于图像识别、分类或其他任务。OpenCV中常用的特征提取算法包括:
- **直方图:**计算图像中像素值在不同范围内的分布。
```cpp
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 计算直方图
hist = cv2.calcHist([image], [0], None, [256], [0, 256])
# 归一化直方图
hist = hist / np.sum(hist)
# 绘制直方图
plt.plot(hist)
plt.show()
```
- **SIFT:**检测图像中的尺度不变特征点。
```cpp
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 检测 SIFT 特征点
sift = cv2.SIFT_create()
keypoints, descriptors = sift.detectAndCompute(image, None)
# 绘制特征点
cv2.drawKeypoints(image, keypoints, image)
# 显示图像
cv2.imshow('SIFT Features', image)
cv2.waitKey(0)
```
- **HOG:**计算图像中梯度方向直方图。
```cpp
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 计算 HOG 特征
hog = cv2.HOGDescriptor()
features = hog.compute(image)
# 打印特征
print(features)
```
### 4.3 图像识别
图像识别是使用图像中提取的特征来识别对象或场景的过程。OpenCV中常用的图像识别算法包括:
- **模板匹配:**将模板图像与目标图像进行比较,以找到模板在目标图像中的位置。
```cpp
import cv2
# 读取模板图像
template = cv2.imread('template.jpg')
# 读取目标图像
image = cv2.imread('image.jpg')
# 应用模板匹配
result = cv2.matchTemplate(image, template, cv2.TM_CCOEFF_NORMED)
# 找到匹配位置
min_val, max_val, min_loc, max_loc = cv2.minMaxLoc(result)
# 绘制匹配框
cv2.rectangle(image, max_loc, (max_loc[0] + template.shape[1], max_loc[1] + template.shape[0]), (0, 255, 0), 2)
# 显示图像
cv2.imshow('Image Recognition', image)
cv2.waitKey(0)
```
- **KNN:**使用 K 最近邻算法对图像进行分类。
```cpp
import cv2
import numpy as np
# 训练数据
train_data = np.array([[0, 0, 0], [0, 255, 0], [255, 0, 0]])
train_labels = np.array([0, 1, 2])
# 测试数据
test_data = np.array([[127, 127, 127]])
# 创建 KNN 分类器
knn = cv2.ml.KNearest_create()
knn.train(train_data, cv2.ml.ROW_SAMPLE, train_labels)
# 预测测试数据
ret, result, neighbours, dist = knn.findNearest(test_data, 1)
# 打印预测结果
print(result)
```
- **SVM:**使用支持向量机算法对图像进行分类。
```cpp
import cv2
import numpy as np
# 训练数据
train_data = np.array([[0, 0, 0], [0, 255, 0], [255, 0, 0]])
train_labels = np.array([0, 1, 2])
# 测试数据
test_data = np.array([[127, 127, 127]])
# 创建 SVM 分类器
svm = cv2.ml.SVM_create()
svm.train(train_data, cv2.ml.ROW_SAMPLE, train_labels)
# 预测测试数据
ret, result = svm.predict(test_data)
# 打印预测结果
print(result)
```
# 5.1 物体检测
物体检测是计算机视觉中一项基本任务,其目标是识别图像或视频帧中的对象并确定其位置。OpenCV 提供了一系列强大的算法来执行物体检测,包括:
### 5.1.1 Haar 级联分类器
Haar 级联分类器是一种基于机器学习的物体检测算法,它使用预训练的模型来识别特定对象。它通过在图像中搜索一系列矩形特征来工作,这些特征是针对特定对象进行训练的。
**优点:**
* 快速且高效
* 对小物体检测效果良好
**缺点:**
* 需要大量训练数据
* 仅限于检测预先训练的特定对象
### 5.1.2 HOG 描述符
HOG(梯度直方图)描述符是一种基于图像梯度的物体检测算法。它通过计算图像中局部区域的梯度方向和大小来工作,然后将这些梯度信息编码为直方图。
**优点:**
* 对光照变化和背景杂波具有鲁棒性
* 可用于检测各种对象
**缺点:**
* 比 Haar 级联分类器慢
* 对大物体检测效果不佳
### 5.1.3 深度学习方法
深度学习方法,如卷积神经网络(CNN),是物体检测的最新技术。它们通过使用多层神经网络从图像数据中学习特征,从而实现更高的准确性和鲁棒性。
**优点:**
* 准确性高
* 可用于检测各种对象
**缺点:**
* 需要大量训练数据
* 计算成本高
### 代码示例
以下代码示例演示了如何使用 OpenCV 中的 Haar 级联分类器检测图像中的面部:
```cpp
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
// 加载 Haar 级联分类器
CascadeClassifier face_cascade;
face_cascade.load("haarcascade_frontalface_default.xml");
// 加载图像
Mat image = imread("image.jpg");
// 转换图像为灰度图像
cvtColor(image, image, COLOR_BGR2GRAY);
// 检测图像中的面部
std::vector<Rect> faces;
face_cascade.detectMultiScale(image, faces, 1.1, 3, 0|CV_HAAR_SCALE_IMAGE, Size(30, 30));
// 在图像中绘制检测到的面部
for (Rect face : faces) {
rectangle(image, face, Scalar(0, 255, 0), 2);
}
// 显示检测结果
imshow("Faces", image);
waitKey(0);
return 0;
}
```
**代码逻辑:**
1. 加载 Haar 级联分类器。
2. 加载图像并将其转换为灰度图像。
3. 使用 `detectMultiScale` 函数检测图像中的面部。
4. 在图像中绘制检测到的面部。
5. 显示检测结果。
**参数说明:**
* `face_cascade.detectMultiScale` 函数的参数:
* `image`:要检测的图像。
* `faces`:检测到的面部矩形列表。
* `1.1`:缩放因子。
* `3`:最小邻居数。
* `0|CV_HAAR_SCALE_IMAGE`:缩放图像以提高检测准确性。
* `Size(30, 30)`:最小面部尺寸。
# 6.1 性能优化
在Clion中使用OpenCV时,性能优化至关重要,因为它可以显着提高应用程序的效率和响应能力。以下是一些优化OpenCV代码的最佳实践:
### 1. 使用多线程
OpenCV支持多线程,这可以通过将任务分配给多个内核来提高性能。可以使用`OpenMP`或`pthreads`等库实现多线程。
```cpp
#include <opencv2/opencv.hpp>
#include <omp.h>
int main() {
cv::Mat image = cv::imread("image.jpg");
// 使用OpenMP并行处理图像
#pragma omp parallel for
for (int i = 0; i < image.rows; i++) {
for (int j = 0; j < image.cols; j++) {
// 对图像像素进行处理
}
}
return 0;
}
```
### 2. 使用OpenCL
OpenCL是一个异构编程框架,允许在CPU和GPU上并行执行代码。通过利用GPU的并行处理能力,OpenCV可以显著提高图像处理任务的性能。
```cpp
#include <opencv2/opencv.hpp>
#include <CL/cl.h>
int main() {
cv::Mat image = cv::imread("image.jpg");
// 获取OpenCL平台和设备
cl_platform_id platform_id = clGetPlatformIDs(1, NULL, NULL);
cl_device_id device_id = clGetDeviceIDs(platform_id, CL_DEVICE_TYPE_GPU, 1, NULL, NULL);
// 创建OpenCL上下文和命令队列
cl_context context = clCreateContext(NULL, 1, &device_id, NULL, NULL, NULL);
cl_command_queue queue = clCreateCommandQueue(context, device_id, 0, NULL);
// 将图像数据传输到GPU
cl_mem image_buffer = clCreateBuffer(context, CL_MEM_READ_WRITE, image.size(), NULL, NULL);
clEnqueueWriteBuffer(queue, image_buffer, CL_TRUE, 0, image.size(), image.data, 0, NULL, NULL);
// 在GPU上执行图像处理内核
cl_program program = clCreateProgramWithSource(context, 1, &kernel_source, NULL, NULL);
clBuildProgram(program, 1, &device_id, NULL, NULL, NULL);
cl_kernel kernel = clCreateKernel(program, "image_processing_kernel", NULL);
// 设置内核参数
clSetKernelArg(kernel, 0, sizeof(cl_mem), &image_buffer);
// 执行内核
clEnqueueNDRangeKernel(queue, kernel, 2, NULL, image.size(), NULL, 0, NULL, NULL);
// 将处理后的图像数据从GPU传输回CPU
clEnqueueReadBuffer(queue, image_buffer, CL_TRUE, 0, image.size(), image.data, 0, NULL, NULL);
// 释放OpenCL资源
clReleaseKernel(kernel);
clReleaseProgram(program);
clReleaseCommandQueue(queue);
clReleaseContext(context);
return 0;
}
```
### 3. 使用图像金字塔
图像金字塔是一种将图像表示为一系列不同分辨率版本的技术。通过在较低分辨率的版本上执行图像处理操作,可以减少计算成本。
```cpp
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("image.jpg");
// 创建图像金字塔
std::vector<cv::Mat> pyramid;
cv::buildPyramid(image, pyramid, 5);
// 在金字塔的不同层上执行图像处理操作
for (int i = 0; i < pyramid.size(); i++) {
// 对图像金字塔的第i层进行处理
}
return 0;
}
```
### 4. 使用缓存
缓存可以存储频繁访问的数据,从而减少内存访问的延迟。在OpenCV中,可以使用`cv::Mat::clone()`函数创建图像的缓存。
```cpp
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("image.jpg");
// 创建图像的缓存
cv::Mat image_cache = image.clone();
// 对缓存的图像进行处理
// ...
return 0;
}
```
### 5. 使用优化库
existem várias bibliotecas otimizadas que podem ser usadas para acelerar operações de processamento de imagem em OpenCV. Algumas bibliotecas populares incluem:
- [Eigen](https://eigen.tuxfamily.org/)
- [OpenBLAS](https://www.openblas.net/)
- [Intel IPP](https://software.intel.com/content/www/us/en/develop/tools/intel-integrated-performance-primitives.html)
```cpp
#include <opencv2/opencv.hpp>
#include <Eigen/Eigen>
int main() {
cv::Mat image = cv::imread("image.jpg");
// 使用Eigen库对图像进行处理
Eigen::MatrixXf image_eigen = Eigen::Map<Eigen::MatrixXf>(image.data, image.rows, image.cols);
// ...
return 0;
}
```
0
0
相关推荐
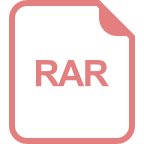
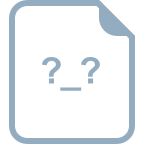
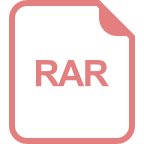
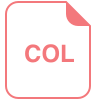
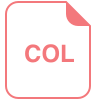
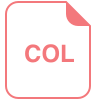
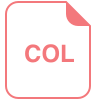

