使用C++,openmp,opencv实现连通区域标记的二遍扫描算法,将读入需要进行连通区域标记的图像,将其二值化,使用动态二维数组来存储每个像素所属的连通区域标记,将输出图像显示在一个窗口中
时间: 2024-06-01 18:09:40 浏览: 102
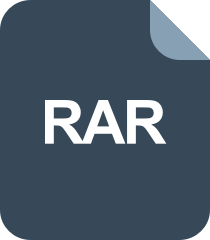
基于vs2013的连通区域标记
#include <stdio.h>
#include <stdlib.h>
#include <omp.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main(int argc, char** argv)
{
if (argc != 2)
{
printf("Usage: ./connected_components <image_path>\n");
return -1;
}
Mat image, gray_image, binary_image;
image = imread(argv[1], IMREAD_COLOR);
if (!image.data)
{
printf("Could not open or find the image.\n");
return -1;
}
cvtColor(image, gray_image, COLOR_BGR2GRAY);
threshold(gray_image, binary_image, 128, 255, THRESH_BINARY);
int rows = binary_image.rows;
int cols = binary_image.cols;
int **label = new int*[rows];
for (int i = 0; i < rows; i++)
label[i] = new int[cols];
// Initialize label matrix to all zeros
for (int i = 0; i < rows; i++)
for (int j = 0; j < cols; j++)
label[i][j] = 0;
int label_count = 1;
// First pass
#pragma omp parallel for
for (int i = 1; i < rows-1; i++)
for (int j = 1; j < cols-1; j++)
if (binary_image.at<uchar>(i,j) == 255)
{
int min_label = 255;
if (label[i-1][j-1] != 0 && label[i-1][j-1] < min_label)
min_label = label[i-1][j-1];
if (label[i-1][j] != 0 && label[i-1][j] < min_label)
min_label = label[i-1][j];
if (label[i-1][j+1] != 0 && label[i-1][j+1] < min_label)
min_label = label[i-1][j+1];
if (label[i][j-1] != 0 && label[i][j-1] < min_label)
min_label = label[i][j-1];
if (min_label == 255)
label[i][j] = label_count++;
else
label[i][j] = min_label;
if (label[i-1][j-1] != 0 && label[i-1][j-1] != min_label)
label[label[i-1][j-1]][j-1] = min_label;
if (label[i-1][j] != 0 && label[i-1][j] != min_label)
label[label[i-1][j]][j] = min_label;
if (label[i-1][j+1] != 0 && label[i-1][j+1] != min_label)
label[label[i-1][j+1]][j+1] = min_label;
if (label[i][j-1] != 0 && label[i][j-1] != min_label)
label[label[i][j-1]][j-1] = min_label;
}
// Second pass
#pragma omp parallel for
for (int i = 1; i < rows-1; i++)
for (int j = 1; j < cols-1; j++)
if (label[i][j] != 0)
{
int root = label[i][j];
while (root != label[root/cols][root%cols])
root = label[root/cols][root%cols];
label[i][j] = root;
}
// Color each connected component with a different color
Vec3b colors[label_count];
for (int i = 0; i < label_count; i++)
colors[i] = Vec3b(rand()%256, rand()%256, rand()%256);
Mat output_image(rows, cols, CV_8UC3);
for (int i = 0; i < rows; i++)
for (int j = 0; j < cols; j++)
output_image.at<Vec3b>(i,j) = colors[label[i][j]];
namedWindow("Output Image", WINDOW_AUTOSIZE);
imshow("Output Image", output_image);
waitKey(0);
return 0;
}
阅读全文
相关推荐
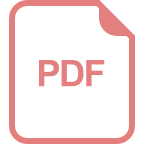
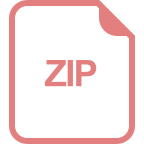















