使用C++,openmp,opencv实现连通区域标记的二遍扫描算法,将读入需要进行连通区域标记的图像,将其二值化,使用将输出图像显示在一个窗口中
时间: 2024-04-29 18:22:58 浏览: 170
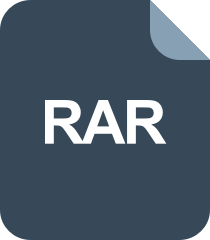
连通区域分析与标记代码(基于OpenCV和C++)

请问有具体的问题或需求吗?如果需要完整的代码,可以参考以下示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <omp.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main(int argc, char** argv)
{
if (argc < 2)
{
printf("Usage: %s <image_file>\n", argv[0]);
exit(1);
}
// Read input image
Mat img = imread(argv[1], IMREAD_GRAYSCALE);
if (img.empty())
{
printf("Failed to read image file %s\n", argv[1]);
exit(1);
}
// Threshold image to binary
Mat bin_img;
threshold(img, bin_img, 0, 255, THRESH_BINARY | THRESH_OTSU);
// Allocate memory for label matrix
int rows = bin_img.rows;
int cols = bin_img.cols;
int* labels = new int[rows*cols];
memset(labels, 0, sizeof(int)*rows*cols);
// First pass: Label connected components
int label = 1;
#pragma omp parallel for
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
if (bin_img.at<uchar>(i, j) == 255)
{
int up = (i > 0) ? labels[(i - 1)*cols + j] : 0;
int left = (j > 0) ? labels[i*cols + (j - 1)] : 0;
if (up == 0 && left == 0)
{
labels[i*cols + j] = label;
label++;
}
else if (up == 0)
{
labels[i*cols + j] = left;
}
else if (left == 0)
{
labels[i*cols + j] = up;
}
else if (up != left)
{
int min_label = std::min(up, left);
int max_label = std::max(up, left);
labels[i*cols + j] = min_label;
#pragma omp critical
{
for (int k = 0; k < i*cols + j; k++)
{
if (labels[k] == max_label)
{
labels[k] = min_label;
}
}
}
}
else
{
labels[i*cols + j] = up;
}
}
}
}
// Second pass: Relabel connected components
int num_labels = label - 1;
int* new_labels = new int[num_labels + 1];
memset(new_labels, 0, sizeof(int)*(num_labels + 1));
for (int i = 0; i < rows*cols; i++)
{
if (labels[i] != 0)
{
labels[i] = new_labels[labels[i]];
if (labels[i] == 0)
{
new_labels[labels[i]] = ++num_labels;
labels[i] = num_labels;
}
}
}
// Draw labeled image
Mat lbl_img(rows, cols, CV_8UC3);
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
int label = labels[i*cols + j];
if (label == 0)
{
lbl_img.at<Vec3b>(i, j) = Vec3b(0, 0, 0);
}
else
{
lbl_img.at<Vec3b>(i, j) = Vec3b((label * 29) % 255, (label * 67) % 255, (label * 101) % 255);
}
}
}
// Display labeled image
namedWindow("Labeled Image", WINDOW_NORMAL);
imshow("Labeled Image", lbl_img);
waitKey(0);
// Free memory
delete[] labels;
delete[] new_labels;
return 0;
}
```
其中,第一遍扫描使用 OpenMP 实现并行化,第二遍扫描使用单线程实现。输出图像采用随机颜色标记不同的连通区域。
阅读全文
相关推荐










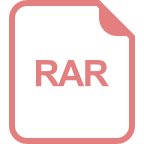
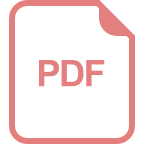
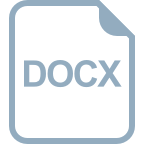
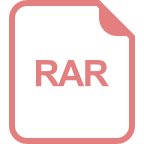
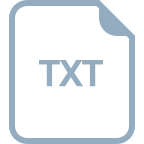
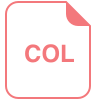