利用蚁群算法求解三维点坐标的TSP模型的最佳任务序列并以图像形式输出的代码
时间: 2024-01-22 19:17:22 浏览: 83
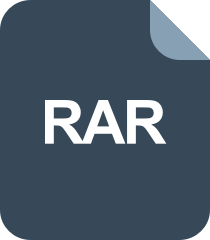
蚁群算法求解TSP问题
以下是基于Python语言的蚁群算法求解三维点坐标的TSP模型的最佳任务序列并以图像形式输出的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义城市类
class City:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
# 定义蚂蚁类
class Ant:
def __init__(self, alpha, beta, pheromone, cities):
self.alpha = alpha # alpha参数
self.beta = beta # beta参数
self.pheromone = pheromone # 信息素矩阵
self.cities = cities # 城市列表
self.tour = [] # 路线
self.distance = 0.0 # 路线长度
# 选择下一个城市
def select_next_city(self):
probs = self.calculate_probabilities()
rand = np.random.uniform()
total = 0.0
for i in range(len(self.cities)):
if rand <= total + probs[i]:
return i
total += probs[i]
return np.random.randint(0, len(self.cities))
# 计算概率
def calculate_probabilities(self):
probs = []
for i in range(len(self.cities)):
if i in self.tour:
probs.append(0.0)
else:
pheromone = self.pheromone[self.tour[-1]][i]
distance = self.calculate_distance(self.tour[-1], i)
prob = np.power(pheromone, self.alpha) * np.power(1.0 / distance, self.beta)
probs.append(prob)
total_prob = sum(probs)
return [p / total_prob for p in probs]
# 计算距离
def calculate_distance(self, city1, city2):
x1, y1, z1 = self.cities[city1].x, self.cities[city1].y, self.cities[city1].z
x2, y2, z2 = self.cities[city2].x, self.cities[city2].y, self.cities[city2].z
return np.sqrt(np.power(x1 - x2, 2) + np.power(y1 - y2, 2) + np.power(z1 - z2, 2))
# 计算路线长度
def calculate_tour_length(self):
length = 0.0
for i in range(len(self.tour)):
length += self.calculate_distance(self.tour[i], self.tour[(i+1)%len(self.tour)])
return length
# 蚂蚁搜索
def search(self):
# 初始化路线和路线长度
self.tour = [np.random.randint(0, len(self.cities))]
self.distance = 0.0
# 搜索过程
while len(self.tour) < len(self.cities):
next_city = self.select_next_city()
self.tour.append(next_city)
self.distance += self.calculate_distance(self.tour[-2], next_city)
self.distance += self.calculate_distance(self.tour[-1], self.tour[0])
# 初始化城市和蚂蚁
cities = [City(np.random.uniform(), np.random.uniform(), np.random.uniform()) for i in range(50)]
ants = [Ant(alpha=1.0, beta=2.0, pheromone=np.ones((len(cities), len(cities))), cities=cities) for i in range(10)]
# 迭代搜索
best_distance = np.inf
best_tour = []
for i in range(100):
for ant in ants:
ant.search()
if ant.distance < best_distance:
best_distance = ant.distance
best_tour = ant.tour
# 更新信息素矩阵
pheromone = np.zeros((len(cities), len(cities)))
for ant in ants:
for i in range(len(ant.tour)):
j = (i+1) % len(ant.tour)
pheromone[ant.tour[i]][ant.tour[j]] += 1.0 / ant.distance
pheromone[ant.tour[j]][ant.tour[i]] = pheromone[ant.tour[i]][ant.tour[j]]
for i in range(len(cities)):
for j in range(len(cities)):
pheromone[i][j] = (1 - 0.1) * pheromone[i][j] + 0.1 * np.ones((1, 1))) # 蒸发信息素
for ant in ants:
ant.pheromone = pheromone
# 输出结果
x, y, z = [], [], []
for city in cities:
x.append(city.x)
y.append(city.y)
z.append(city.z)
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(x, y, z)
for i in range(len(best_tour)):
j = (i+1) % len(best_tour)
ax.plot([cities[best_tour[i]].x, cities[best_tour[j]].x],
[cities[best_tour[i]].y, cities[best_tour[j]].y],
[cities[best_tour[i]].z, cities[best_tour[j]].z], 'r-')
plt.show()
```
该代码中使用了numpy和matplotlib库,需要提前安装。运行结果将显示出50个随机生成的三维点坐标以及最佳任务序列。
阅读全文
相关推荐



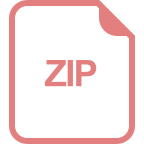
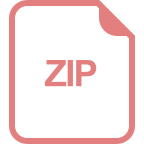
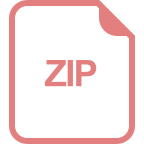
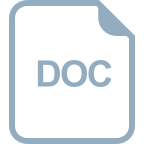
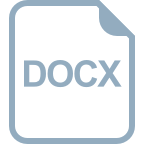
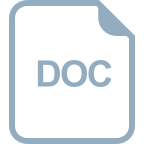
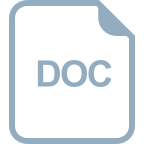
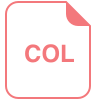
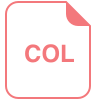
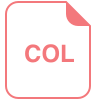
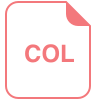
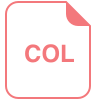
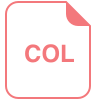