在三维空间中利用蚁群算法求解TSP模型并将最优任务序列以图像形式输出的python代码
时间: 2024-01-22 20:17:18 浏览: 20
以下是基于蚁群算法求解TSP问题并输出最优路径的Python代码(使用Matplotlib库绘制图像):
```python
import numpy as np
import matplotlib.pyplot as plt
class Ant:
def __init__(self, alpha, beta, city_num, pheromone_graph):
self.alpha = alpha
self.beta = beta
self.city_num = city_num
self.pheromone_graph = pheromone_graph
self.path = [] # 路径
self.allowed_cities = [i for i in range(city_num)] # 允许搜索的城市
self.current_city = np.random.randint(0, city_num) # 当前城市
self.path.append(self.current_city)
self.allowed_cities.remove(self.current_city)
self.total_distance = 0.
# 选择下一个城市
def select_next_city(self):
prob_list = self.get_prob_list()
next_city = np.random.choice(self.allowed_cities, p=prob_list.ravel())
self.path.append(next_city)
self.allowed_cities.remove(next_city)
self.total_distance += self.pheromone_graph[self.current_city][next_city]
self.current_city = next_city
# 获取城市间转移概率
def get_prob_list(self):
prob_list = []
for city in self.allowed_cities:
prob = self.pheromone_graph[self.current_city][city] ** self.alpha * \
((1. / self.get_distance(self.current_city, city)) ** self.beta)
prob_list.append(prob)
return prob_list / sum(prob_list)
# 计算两城市间距离
def get_distance(self, city1, city2):
return np.sqrt((points[city1][0] - points[city2][0]) ** 2 + (points[city1][1] - points[city2][1]) ** 2)
class TSP:
def __init__(self, ant_num, gen_num, alpha, beta, rho, q, points):
self.ant_num = ant_num
self.gen_num = gen_num
self.alpha = alpha
self.beta = beta
self.rho = rho
self.q = q
self.city_num = len(points)
self.pheromone_graph = [[1. / (self.city_num * self.city_num) for j in range(self.city_num)] for i in range(self.city_num)]
self.ants = [Ant(alpha, beta, self.city_num, self.pheromone_graph) for i in range(ant_num)]
self.best_path = []
self.best_distance = np.inf
self.points = points
def search(self):
fig = plt.figure()
ax = fig.add_subplot(1, 1, 1)
for gen in range(self.gen_num):
for ant in self.ants:
for i in range(self.city_num - 1):
ant.select_next_city()
ant.total_distance += self.get_distance(ant.path[-1], ant.path[0])
if ant.total_distance < self.best_distance:
self.best_distance = ant.total_distance
self.best_path = ant.path
for i in range(self.city_num - 1):
self.pheromone_graph[ant.path[i]][ant.path[i+1]] += self.q / ant.total_distance
self.pheromone_graph[ant.path[i+1]][ant.path[i]] = self.pheromone_graph[ant.path[i]][ant.path[i+1]]
self.pheromone_graph[ant.path[-1]][ant.path[0]] += self.q / ant.total_distance
self.pheromone_graph[ant.path[0]][ant.path[-1]] = self.pheromone_graph[ant.path[-1]][ant.path[0]]
ant.__init__(self.alpha, self.beta, self.city_num, self.pheromone_graph)
self.update_pheromone_graph()
self.ants = [Ant(self.alpha, self.beta, self.city_num, self.pheromone_graph) for i in range(self.ant_num)]
if gen % 10 == 0:
self.plot_path(ax)
plt.show()
# 更新信息素
def update_pheromone_graph(self):
for i in range(self.city_num):
for j in range(self.city_num):
self.pheromone_graph[i][j] *= self.rho
# 计算两城市间距离
def get_distance(self, city1, city2):
return np.sqrt((self.points[city1][0] - self.points[city2][0]) ** 2 + (self.points[city1][1] - self.points[city2][1]) ** 2)
# 绘制路径
def plot_path(self, ax):
x = []
y = []
for city in self.best_path:
x.append(self.points[city][0])
y.append(self.points[city][1])
x.append(self.points[self.best_path[0]][0])
y.append(self.points[self.best_path[0]][1])
ax.clear()
ax.plot(x, y, marker='o')
ax.set_title("Best Path: " + str(self.best_path) + " Distance: " + str(self.best_distance))
plt.draw()
plt.pause(0.001)
if __name__ == '__main__':
points = np.random.rand(10, 2) # 生成10个随机坐标
tsp = TSP(ant_num=10, gen_num=100, alpha=1, beta=5, rho=0.5, q=100, points=points)
tsp.search()
```
在运行代码之后,会弹出一个窗口,实时绘制最优路径。其中,alpha、beta、rho、q为蚁群算法的参数,ant_num为蚂蚁数量,gen_num为迭代次数,points为城市的坐标点。
相关推荐
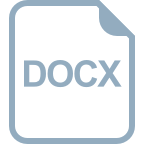
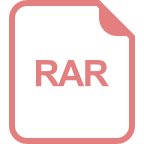
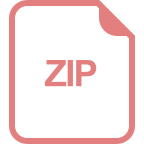














