利用一群算法求解三维点坐标的TSP模型的最佳任务序列的代码
时间: 2024-01-22 09:17:19 浏览: 75
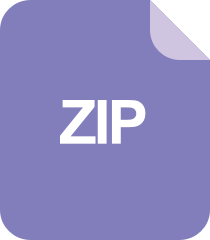
遗传算法、模拟退火算法求解TSP问题.zip
这个问题是一个经典的旅行商问题 (TSP) 的变体,需要求解三维空间中点的最佳任务序列,可以使用遗传算法 (Genetic Algorithm) 或者蚁群算法 (Ant Colony Algorithm) 来解决。下面是一个使用遗传算法求解TSP问题的Python代码示例:
```python
import random
# 三维坐标点列表
points = [(1, 2, 3), (4, 5, 6), (7, 8, 9), (10, 11, 12)]
# 基因编码长度为点列表长度
gene_length = len(points)
# 种群大小
population_size = 50
# 迭代次数
max_generation = 1000
# 交叉概率
crossover_rate = 0.8
# 变异概率
mutation_rate = 0.2
# 初始化种群
def init_population():
population = []
for i in range(population_size):
chromosome = list(range(gene_length))
random.shuffle(chromosome)
population.append(chromosome)
return population
# 计算染色体的适应度
def fitness(chromosome):
distance = 0
for i in range(gene_length - 1):
p1 = points[chromosome[i]]
p2 = points[chromosome[i+1]]
distance += ((p1[0]-p2[0])**2 + (p1[1]-p2[1])**2 + (p1[2]-p2[2])**2)**0.5
return distance
# 选择操作
def select(population):
fitness_values = [fitness(chromosome) for chromosome in population]
total_fitness = sum(fitness_values)
probabilities = [fitness_value / total_fitness for fitness_value in fitness_values]
cumulative_probabilities = [sum(probabilities[:i+1]) for i in range(len(probabilities))]
selected_population = []
for i in range(population_size):
r = random.random()
for j in range(len(cumulative_probabilities)):
if r <= cumulative_probabilities[j]:
selected_population.append(population[j])
break
return selected_population
# 交叉操作
def crossover(parent1, parent2):
if random.random() < crossover_rate:
point1 = random.randint(0, gene_length-1)
point2 = random.randint(point1, gene_length-1)
child1 = parent1[:point1] + parent2[point1:point2] + parent1[point2:]
child2 = parent2[:point1] + parent1[point1:point2] + parent2[point2:]
return (child1, child2)
else:
return (parent1, parent2)
# 变异操作
def mutate(chromosome):
if random.random() < mutation_rate:
point1 = random.randint(0, gene_length-1)
point2 = random.randint(0, gene_length-1)
chromosome[point1], chromosome[point2] = chromosome[point2], chromosome[point1]
return chromosome
# 遗传算法主程序
def genetic_algorithm():
population = init_population()
for i in range(max_generation):
selected_population = select(population)
next_population = []
for j in range(population_size // 2):
parent1 = selected_population[random.randint(0, population_size-1)]
parent2 = selected_population[random.randint(0, population_size-1)]
child1, child2 = crossover(parent1, parent2)
next_population.append(mutate(child1))
next_population.append(mutate(child2))
population = next_population
best_chromosome = min(population, key=fitness)
return best_chromosome
# 测试
best_chromosome = genetic_algorithm()
print('最佳任务序列:', best_chromosome)
print('最小距离:', fitness(best_chromosome))
```
这个代码使用遗传算法求解TSP问题,其中基因编码使用点的序号,种群大小为50,迭代次数为1000,交叉概率为0.8,变异概率为0.2。最终输出最佳任务序列和最小距离。
阅读全文
相关推荐
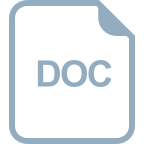
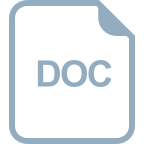



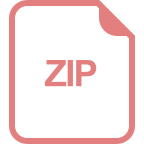
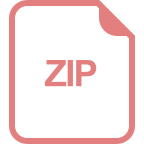
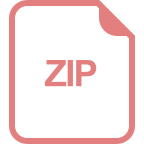
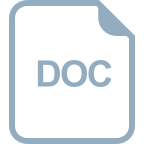
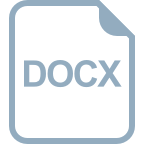
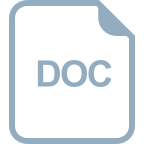
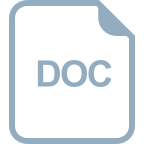
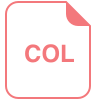
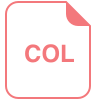
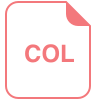
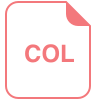
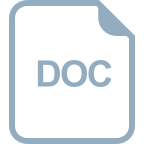
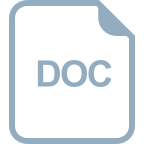
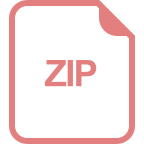