香农编码后怎么译码matlab
时间: 2023-10-31 17:14:11 浏览: 175
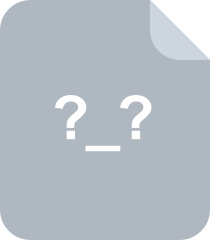
香农编码的matlab代码
香农编码(Shannon-Fano编码)是一种可变长度编码技术,可以将不同的符号映射到不同长度的比特串上,从而实现数据压缩。在解码时,需要根据编码表将比特串转化为原始符号。MATLAB可以很方便地实现香农编码和解码,以下是一个简单的实现过程:
1. 首先,定义要编码的符号及其出现概率。假设有n个符号,第i个符号的出现概率为pi。
2. 根据符号出现概率,生成Shannon-Fano编码表。对符号进行分组,使得每个组中的符号出现概率之和尽量相等,然后对每个组进行递归编码,将0和1分别添加到编码中,直到每个符号都有唯一的编码。
3. 对要编码的数据进行逐个符号编码,将每个符号的编码拼接起来即可得到编码后的比特串。
4. 在解码时,根据编码表,将比特串逐个符号解码,即可得到原始数据。
下面是一个简单的MATLAB代码实现:
```matlab
% 定义符号及其出现概率
symbols = ['A', 'B', 'C', 'D', 'E', 'F'];
probabilities = [0.2, 0.15, 0.1, 0.1, 0.25, 0.2];
% 生成Shannon-Fano编码表
code_table = shannon_fano(symbols, probabilities);
% 要编码的数据
data = 'ABBCADEF';
% 编码
code = shannon_fano_encode(data, code_table)
% 解码
decoded_data = shannon_fano_decode(code, code_table)
% Shannon-Fano编码函数
function code_table = shannon_fano(symbols, probabilities)
% 递归函数,将符号分组并编码
function code_table = shannon_fano_helper(symbols, probabilities, prefix)
n = length(symbols);
if n == 1
% 如果只有一个符号,则直接返回其编码
code_table = struct('symbol', symbols, 'code', prefix);
else
% 将符号按照出现概率从大到小排序
[~, idx] = sort(probabilities, 'descend');
symbols = symbols(idx);
probabilities = probabilities(idx);
% 找到分组位置
cum_prob = cumsum(probabilities);
[~, group_pos] = min(abs(cum_prob - sum(probabilities) / 2));
% 对两个组递归编码,将0和1分别加入编码
code_table1 = shannon_fano_helper(symbols(1:group_pos), probabilities(1:group_pos), strcat(prefix, '0'));
code_table2 = shannon_fano_helper(symbols(group_pos+1:end), probabilities(group_pos+1:end), strcat(prefix, '1'));
% 合并编码表
code_table = [code_table1, code_table2];
end
end
% 调用递归函数生成编码表
code_table = shannon_fano_helper(symbols, probabilities, '');
end
% Shannon-Fano编码函数
function code = shannon_fano_encode(data, code_table)
n = length(data);
code = '';
for i = 1:n
idx = find([code_table.symbol] == data(i));
code = strcat(code, code_table(idx).code);
end
end
% Shannon-Fano解码函数
function decoded_data = shannon_fano_decode(code, code_table)
n = length(code);
decoded_data = '';
i = 1;
while i <= n
j = i;
while j <= n
idx = find(strcmp({code_table.code}, code(i:j)));
if ~isempty(idx)
decoded_data = strcat(decoded_data, code_table(idx).symbol);
i = j + 1;
break;
end
j = j + 1;
end
if j > n
error('无法解码:无匹配的编码')
end
end
end
```
以上是一个简单的MATLAB实现,可以根据具体情况进行修改和调整。
阅读全文
相关推荐
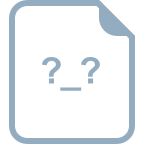






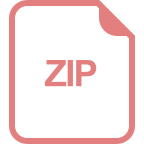
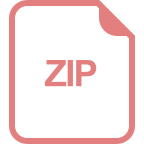
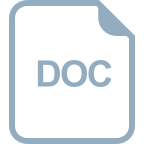


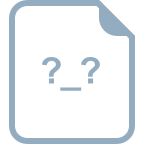
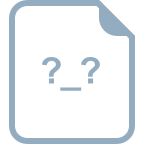
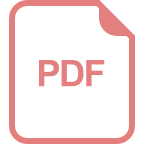
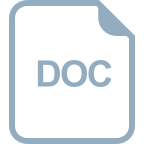
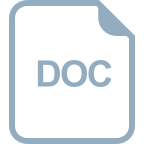