raise TypeError(f"cannot convert the series to {converter}") TypeError: cannot convert the series to <class 'float'>
时间: 2024-05-29 19:11:31 浏览: 53
This error message indicates that the code is attempting to convert a pandas series (a collection of data) to a float data type, but this is not possible. The specific line of code that is causing the error would be helpful in understanding the context of the issue.
One possible solution could be to check the data type of the series and convert it to a compatible data type before attempting to convert it to a float. Alternatively, the code may need to be restructured to handle the data differently or to use a different data type altogether.
相关问题
TypeError: cannot convert the series to <class 'float'>
This error occurs when you try to convert a pandas series object to a float data type using a function or method that expects a single value.
For example, if you have a pandas dataframe with a column 'salary' and you try to convert it to a float using the float() function like this:
```
import pandas as pd
df = pd.read_csv('employees.csv')
salary = df['salary']
float(salary)
```
You'll get the TypeError because the float() function expects a single value but the 'salary' column is a series object with multiple values.
To fix this error, you can either convert each value in the series to a float using a loop or apply() method or use a pandas method like astype() to convert the entire series to a float data type.
Here's an example of using the astype() method:
```
salary = df['salary'].astype(float)
```
This will convert the entire 'salary' column to a float data type.
TypeError: cannot convert the series to <class 'int'>
这个错误通常出现在 Pandas 中,是因为你尝试将一个 Pandas Series 对象转换为整数类型。Pandas Series 对象是一维标记数组,其中包含数据值。如果你想对 Pandas Series 进行数学运算,需要使用 Pandas 提供的函数,比如 apply()。
例如,假设你有一个名为 data 的 Pandas Series,你想对它进行数学运算。你可以这样做:
```
import pandas as pd
data = pd.Series([1, 2, 3, 4, 5])
result = data.apply(lambda x: x - 1)
print(result)
```
这将输出一个新的 Pandas Series,其中每个值都减去了 1。
如果你确信 Series 中只包含一个整数值,并且你想将其转换为整数类型,你可以使用 iloc 属性来获取该值,并使用 int() 函数将其转换为整数。例如:
```
import pandas as pd
data = pd.Series([42])
result = int(data.iloc[0])
print(result)
```
这将输出整数值 42。
相关推荐
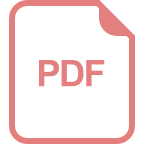
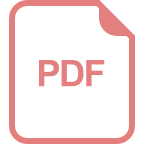
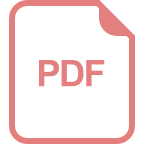











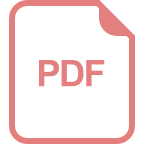
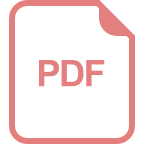