springboot发布webservice
时间: 2023-05-31 16:19:47 浏览: 334
### 回答1:
Spring Boot可以轻松地发布Web服务,只需要遵循以下步骤:
1. 创建一个Spring Boot项目,并添加Web服务的依赖。
2. 创建一个WebService类,并使用@WebService注解标注该类。
3. 在WebService类中定义WebService的方法,并使用@WebMethod注解标注该方法。
4. 在Spring Boot的配置文件中添加WebService的配置信息。
5. 启动Spring Boot应用程序,并访问WebService的URL地址,即可调用WebService的方法。
以上就是使用Spring Boot发布WebService的基本步骤。
### 回答2:
Spring Boot 是一款非常流行的开源 Java 框架,它的特点是快速构建配置后端应用程序。它提供了很多功能,其中一个重要的特点是支持 WebService。下面我将介绍 Spring Boot 如何发布 WebService。
1. 添加依赖
Spring Boot 通过添加spring-boot-starter-web-services依赖来支持 WebService。可以在pom.xml文件中添加以下代码:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
</dependency>
```
2. 创建 Endpoint
Spring Boot 需要通过 Endpoint 来发布 WebService。创建 Endpoint 的方式有两种:
(1)使用 @Endpoint 注解标记的类。这种方式适用于需要手动管理 Endpoint 的情况。
(2)使用 @WebService 注解标记的类。这种方式适用于 Endpoint 的创建和管理都由 Spring 进行管理的情况。
例如,我们创建一个 HelloWorld 的 Endpoint,代码如下:
```java
@Endpoint
public class HelloWorldEndpoint {
@PayloadRoot(namespace = "http://example.com", localPart = "HelloRequest")
@ResponsePayload
public HelloResponse sayHello(@RequestPayload HelloRequest request) {
HelloResponse response = new HelloResponse();
response.setMessage("Hello " + request.getName() + "!");
return response;
}
}
```
3. 配置 Endpoint
要发布一个 Endpoint,需要将其向 JAX-WS 发布,可以在配置类中添加以下代码:
```java
@Configuration
@EnableWs
public class WebServiceConfig extends WsConfigurerAdapter {
@Bean
public ServletRegistrationBean<MessageDispatcherServlet> messageDispatcherServlet(ApplicationContext context) {
MessageDispatcherServlet servlet = new MessageDispatcherServlet();
servlet.setApplicationContext(context);
servlet.setTransformWsdlLocations(true);
return new ServletRegistrationBean<>(servlet, "/ws/*");
}
@Bean(name = "hello")
public DefaultWsdl11Definition defaultWsdl11Definition(XsdSchema helloSchema) {
DefaultWsdl11Definition wsdl11Definition = new DefaultWsdl11Definition();
wsdl11Definition.setPortTypeName("HelloPort");
wsdl11Definition.setLocationUri("/ws");
wsdl11Definition.setTargetNamespace("http://example.com");
wsdl11Definition.setSchema(helloSchema);
return wsdl11Definition;
}
@Bean
public XsdSchema helloSchema() {
return new SimpleXsdSchema(new ClassPathResource("xsd/hello.xsd"));
}
}
```
4. 创建 WSDL
创建一个包含 Endpoint 信息的 WSDL 文件,例如 hello.xsd,代码如下:
```xml
<?xml version="1.0"?>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema"
xmlns:tns="http://example.com" targetNamespace="http://example.com">
<xs:element name="HelloRequest" type="tns:helloRequest"/>
<xs:element name="HelloResponse" type="tns:helloResponse"/>
<xs:complexType name="helloRequest">
<xs:sequence>
<xs:element name="name" type="xs:string"/>
</xs:sequence>
</xs:complexType>
<xs:complexType name="helloResponse">
<xs:sequence>
<xs:element name="message" type="xs:string"/>
</xs:sequence>
</xs:complexType>
</xs:schema>
```
5. 启动应用
将所有代码打包成 WAR 包,并部署到 Tomcat 等 Web 服务器上。然后就可以通过 http://localhost:8080/ws/hello.wsdl 访问 WSDL 文件,例如:
```xml
<wsdl:definitions xmlns:wsdl="http://schemas.xmlsoap.org/wsdl/"
xmlns:tns="http://example.com"
xmlns:xsd="http://www.w3.org/2001/XMLSchema"
targetNamespace="http://example.com">
<wsdl:types>
<xsd:schema targetNamespace="http://example.com">
<xsd:import namespace="http://example.com" schemaLocation="hello.xsd"/>
</xsd:schema>
</wsdl:types>
<wsdl:message name="HelloRequest">
<wsdl:part name="parameters" element="tns:HelloRequest"/>
</wsdl:message>
<wsdl:message name="HelloResponse">
<wsdl:part name="parameters" element="tns:HelloResponse"/>
</wsdl:message>
<wsdl:portType name="HelloPort">
<wsdl:operation name="sayHello">
<wsdl:input message="tns:HelloRequest"/>
<wsdl:output message="tns:HelloResponse"/>
</wsdl:operation>
</wsdl:portType>
<wsdl:binding name="HelloPortSoap11" type="tns:HelloPort">
<soap11:binding xmlns:soap11="http://schemas.xmlsoap.org/wsdl/soap/"
style="document" transport="http://schemas.xmlsoap.org/soap/http"/>
<wsdl:operation name="sayHello">
<soap11:operation soapAction=""/>
<wsdl:input>
<soap11:body use="literal"/>
</wsdl:input>
<wsdl:output>
<soap11:body use="literal"/>
</wsdl:output>
</wsdl:operation>
</wsdl:binding>
<wsdl:service name="HelloService">
<wsdl:port name="HelloPort" binding="tns:HelloPortSoap11">
<soap11:address location="http://localhost:8080/ws"/>
</wsdl:port>
</wsdl:service>
</wsdl:definitions>
```
6. 测试 WebService
现在我们可以使用任何支持 WebService 的客户端来测试我们在 Spring Boot 中发布的 WebService。打开 SoapUI 客户端,输入 WSDL 地址,点击导入即可自动创建服务代码,然后就可以测试我们的 WebService 服务了。
综上所述,借助 Spring Boot,我们可以构建 WebService 并快速发布它们。
### 回答3:
Spring Boot 是一种快速构建 Spring 应用程序的框架,可以帮助程序员更快地构建 web 服务。Spring Boot 同时支持 REST 和 SOAP 两种形式的 web 服务。本文将讨论如何使用 Spring Boot 发布 web 服务,特别是 SOAP 服务。
在 Spring Boot 中发布 SOAP 服务需要使用 Spring Web Services 模块。该模块提供了一个许多美元的框架去构建和开发SOAP Web服务。在创建 SOAP Web 服务的过程中,你将要使用以下几个 Spring 模块:Spring Boot starter web、Spring Boot starter test 和 Spring Boot starter web services。
以下是创建 SOAP 服务步骤:
1. 创建一个 Spring Boot 项目,添加 Web Services 的依赖,如下所示:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
</dependency>
2. 创建一个 WSDL 文件。WSDL 文件描述了 SOAP 服务。你可以使用 WSDL 编辑器(例如 Eclipse)来创建该文件。
3. 创建一个 SOAP 服务端点类。
使用 @Endpoint 注释来声明该类是组件,它将处理所有的 SOAP 请求。
使用 @PayloadRoot 注释来绑定特定的承载体到一些处理方法。
3. 实现 SOAP 服务。
在步骤 3 创建的 SOAP 服务端点类中,定义一个方法用于接收请求并返回响应。
将方法的名称与操作名称相同。
将方法的返回类型与 output 元素对应的类型相同。
4. 创建 Web 服务的配置类。
启用 Spring-web 服务对 SOAP web 服务的支持。
使用 @EnableWs 注释开启 Spring Web Servicess 的功能。
通过 bean() 方法将在步骤 3 中创建的 SOAP 服务端点类添加到 Spring 上下文中。
5. 测试 SOAP 服务
在得到一个运行的 Web 服务之后,我们需要测试 SOAP 服务是否运行正常,我们可以使用 SoapUI 软件来测试。
总结:
Spring官方自带的 Spring Web Services 模块是一个支持 SOAP 服务的框架,在使用 Spring Boot 构建项目的时候,尤其是构建其它微服务架构的项目,是一个非常好的选择。在日常构建项目中,对于服务接口尽可能的使用REST,对于某些非常适合使用SOAP时,我们完全可以使用这个框架。
阅读全文
相关推荐
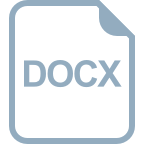

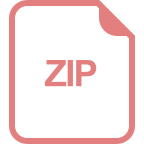
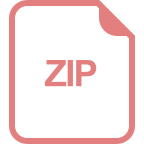
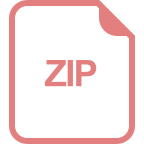
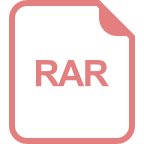
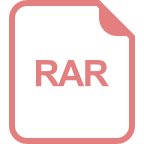
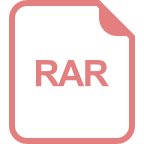
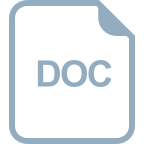



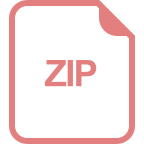
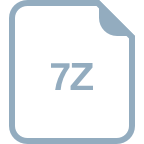
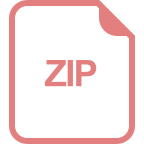

