python 霍夫变换来检测图像上的直线,然后确定该直线的起始点
时间: 2023-06-10 14:03:00 浏览: 102
和终止点的位置,你能给出这个算法的大致流程吗?
当一幅图像中包含了较多的直线,并且这些直线都可以被表示为 y = mx + b 的形式,那么霍夫变换就可以被用来检测出这些直线。其基本思想是由直线上的每个点,计算出所有可能的直线参数(即斜率 m 和截距 b),并在参数空间中进行投票。每个直线参数都对应参数空间上的一条直线,直线上的所有投票对应于在图像上找到了一条直线,其斜率和截距与参数空间中的直线参数相对应。最终,投票最多的直线参数被认为是图像中的一条直线。
具体流程如下:
1. 对图像进行 Canny 边缘检测,得到图像中的所有边缘。
2. 初始化一个空的参数空间 accumulator,并规定步长。可以根据图像大小和图像中直线的密集程度来设置 accumulator 的大小和步长。
3. 对于每个边缘像素,计算所有可能的斜率和截距,并在相应的 accumulator 单元格中进行投票。
4. 在 accumulator 中找到投票最多的直线参数,对应于图像中的一条直线。
5. 通过起始点和终止点估计直线的长度和位置。
希望这个简单的算法流程能对您有所帮助。
相关问题
基于python,写一个指针式仪表自动读数的代码。首先对输入的图像进行霍夫直线检测出指针,再求得指针与起始刻度的夹角。最后根据仪表配置文件中的起始终止刻度角度计算出指针式仪表的读数,实现指针式仪表的自动读数功能。
好的,我明白你的要求了。以下是基于Python的代码实现指针式仪表自动读数功能:
首先需要导入相关的库,包括cv2(OpenCV),numpy和math:
```python
import cv2
import numpy as np
import math
```
然后定义一个函数来进行霍夫直线检测,从而找到指针:
```python
def get_pointer(img):
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
lines = cv2.HoughLines(edges, 1, np.pi / 180, 200)
pointer = None
if lines is not None:
for line in lines:
rho, theta = line[0]
if abs(theta - np.pi / 2) < np.pi / 3:
a = math.cos(theta)
b = math.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
pointer = ((x1, y1), (x2, y2))
break
return pointer
```
上述代码中,首先将输入图像转换为灰度图像,然后使用Canny算子进行边缘检测。接下来使用霍夫直线变换检测所有直线,并选择与y轴夹角在60度以内的直线作为指针的候选线。最后返回指针的起始和结束点坐标。
接下来,定义一个函数来计算指针相对于起始刻度的夹角:
```python
def get_angle(pointer, start_angle):
dx = pointer[1][0] - pointer[0][0]
dy = pointer[1][1] - pointer[0][1]
angle = math.atan2(dy, dx) * 180 / np.pi
if angle < 0:
angle += 360
angle -= start_angle
if angle < 0:
angle += 360
return angle
```
上述代码中,首先计算指针起始点与结束点的坐标差,然后使用arctan函数计算角度,并将弧度转换为角度。最后将起始角度减去当前角度,得到相对于起始刻度的夹角。
最后,定义一个函数来根据仪表配置文件中的起始终止刻度角度计算出指针式仪表的读数:
```python
def read_scale(angle, start_scale, end_scale, n):
scale_angle = (end_scale - start_scale) / n
scale = int(angle / scale_angle)
if scale < 0:
scale = 0
elif scale > n:
scale = n
return scale
```
上述代码中,首先计算出每个刻度之间的夹角,然后根据当前夹角计算出指针所在的刻度位置,最后返回刻度位置。
综合以上三个函数,可以实现指针式仪表自动读数的功能:
```python
def auto_read_scale(img, start_angle, start_scale, end_scale, n):
pointer = get_pointer(img)
if pointer is None:
return None
angle = get_angle(pointer, start_angle)
scale = read_scale(angle, start_scale, end_scale, n)
return scale
```
上述代码中,auto_read_scale函数接收一个图像、起始角度、起始终止刻度角度以及刻度数,先调用get_pointer函数找到指针的位置,然后使用get_angle函数计算出指针的相对夹角,最后使用read_scale函数计算出指针所在的刻度位置,并返回刻度位置。
希望以上代码可以满足您的需求。
霍夫直线检测源代码,不使用opencv
霍夫直线检测是一种经典的图像处理算法,它可以在图像中检测出直线的位置和方向。以下是使用Python实现的简单霍夫直线检测算法,不使用OpenCV库。
```python
import numpy as np
import math
def hough_transform(img):
width, height = img.shape
theta_max = 180
rho_max = int(math.sqrt(width**2 + height**2))
accumulator = np.zeros((rho_max, theta_max), dtype=np.uint8)
for x in range(width):
for y in range(height):
if img[x][y] == 255:
for theta in range(theta_max):
rho = int(x * math.cos(theta * math.pi / 180) + y * math.sin(theta * math.pi / 180))
accumulator[rho][theta] += 1
return accumulator
def hough_lines(img, threshold):
accumulator = hough_transform(img)
width, height = accumulator.shape
lines = []
for rho in range(width):
for theta in range(height):
if accumulator[rho][theta] >= threshold:
a = math.cos(theta * math.pi / 180)
b = math.sin(theta * math.pi / 180)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * a)
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * a)
lines.append((x1, y1, x2, y2))
return lines
```
该代码包含两个函数:
1. `hough_transform`函数实现了霍夫变换,输入为二值化的图像,输出为霍夫空间中的累加器数组。
2. `hough_lines`函数使用霍夫变换结果,输入为二值化的图像和阈值,输出为所有检测到的直线的起始和结束点坐标。
需要注意的是,由于该实现没有使用OpenCV库,因此需要手动将图像转换为二值化格式。
阅读全文
相关推荐
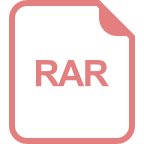
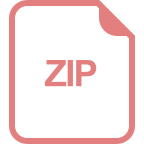
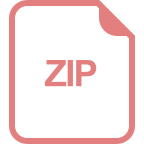
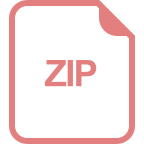
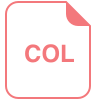
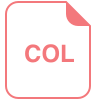
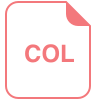
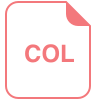

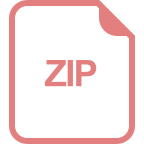