js写一个贪吃蛇
时间: 2023-08-10 21:05:15 浏览: 40
好的,以下是基于HTML5 canvas和JavaScript的简单贪吃蛇游戏代码:
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>贪吃蛇游戏</title>
<style>
#canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="canvas" width="600" height="400"></canvas>
<script src="snake.js"></script>
</body>
</html>
```
JavaScript代码:
```javascript
var canvas = document.getElementById('canvas');
var ctx = canvas.getContext('2d');
var snake = [];
snake[0] = {x: 9*20, y: 10*20};
snake[1] = {x: 8*20, y: 10*20};
snake[2] = {x: 7*20, y: 10*20};
var food = {x: Math.floor(Math.random()*30)*20, y: Math.floor(Math.random()*20)*20};
var direction = 'RIGHT';
function drawSnake() {
for (var i = 0; i < snake.length; i++) {
ctx.fillStyle = (i == 0) ? 'green' : 'white';
ctx.fillRect(snake[i].x, snake[i].y, 20, 20);
ctx.strokeStyle = 'red';
ctx.strokeRect(snake[i].x, snake[i].y, 20, 20);
}
}
function drawFood() {
ctx.fillStyle = 'blue';
ctx.fillRect(food.x, food.y, 20, 20);
ctx.strokeStyle = 'red';
ctx.strokeRect(food.x, food.y, 20, 20);
}
document.addEventListener('keydown', function(event) {
var key = event.keyCode;
if (key == 37 && direction != 'RIGHT') {
direction = 'LEFT';
} else if (key == 38 && direction != 'DOWN') {
direction = 'UP';
} else if (key == 39 && direction != 'LEFT') {
direction = 'RIGHT';
} else if (key == 40 && direction != 'UP') {
direction = 'DOWN';
}
});
function moveSnake() {
var head = {x: snake[0].x, y: snake[0].y};
if (direction == 'LEFT') {
head.x -= 20;
} else if (direction == 'UP') {
head.y -= 20;
} else if (direction == 'RIGHT') {
head.x += 20;
} else if (direction == 'DOWN') {
head.y += 20;
}
if (head.x == food.x && head.y == food.y) {
food = {x: Math.floor(Math.random()*30)*20, y: Math.floor(Math.random()*20)*20};
} else {
snake.pop();
}
snake.unshift(head);
if (head.x < 0 || head.x > canvas.width-20 || head.y < 0 || head.y > canvas.height-20) {
clearInterval(interval);
alert('游戏结束!');
}
for (var i = 1; i < snake.length; i++) {
if (head.x == snake[i].x && head.y == snake[i].y) {
clearInterval(interval);
alert('游戏结束!');
break;
}
}
}
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawSnake();
drawFood();
moveSnake();
}
var interval = setInterval(draw, 100);
```
该代码创建了一个canvas元素,并在其中绘制了贪吃蛇和食物。用户可以通过键盘控制贪吃蛇的移动方向。当贪吃蛇吃到食物时,它会变长,新的食物会随机出现在画布上。如果贪吃蛇撞到边界或自己的身体,则游戏结束。
相关推荐
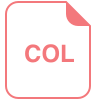
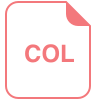
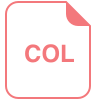
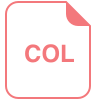
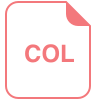









