#include "mylogin.h" mylogin::mylogin(QWidget *parent) : QDialog(parent) { this->init_ui(); connect(this->bnt_login, &QPushButton::clicked, this, &mylogin::do_login); connect(this->bnt_register, &QPushButton::clicked , this ,&mylogin::do_enroll); } mylogin::~mylogin() { } void mylogin::init_ui() { this->setFixedSize(QSize(600,350)); this->setWindowTitle(tr("岑超升")); this->setWindowIcon(QIcon(":/src/1.png")); this->lb1 = new QLabel(); this->lb2 = new QLabel(); this->lb3 = new QLabel(); this->lb1->setFixedSize(QSize(560,200)); QPixmap pic; pic.load(":/src/2.png"); //this->lb1->setPixmap(pic.scaled(this->lb1->size(), Qt::KeepAspectRatio, Qt::SmoothTransformation)); this->lb1->setPixmap(QPixmap(":/src/2.png")); this->lb2->setText(tr("用户名:")); this->lb3->setText(tr("密 码:")); this->usr_name_le = new QLineEdit(); //这两个参数后面传入信号中去,然后emit发射出去 this->usr_pass_le = new QLineEdit(); this->usr_pass_le->setEchoMode(QLineEdit::Password); this->bnt_login = new QPushButton(tr("登 陆")); this->bnt_register = new QPushButton(tr("注 册")); this->hb1 = new QHBoxLayout(); this->hb2 = new QHBoxLayout(); this->hb3 = new QHBoxLayout(); this->hb1->addWidget(this->lb2); this->hb1->addWidget(this->usr_name_le); this->hb2->addWidget(this->lb3); this->hb2->addWidget(this->usr_pass_le); this->hb3->addWidget(this->bnt_login); this->hb3->addWidget(this->bnt_register); this->vb1 = new QVBoxLayout(); this->vb1->addWidget(this->lb1); this->vb1->addLayout(this->hb1); this->vb1->addLayout(this->hb2); this->vb1->addLayout(this->hb3); this->setLayout(this->vb1); } void mylogin::do_login() { emit sig_login(usr_name_le->text(), usr_pass_le->text()); //需要把这里输入的账号密码信息发送到myapp那去,用到的函数是emit //需要自定义一个信号,sig_login //这个槽函数能够发出信号 } void mylogin::do_enroll() { emit sig_enroll(); }用C++语言解释并注释每行代码的语法
时间: 2024-03-18 16:42:29 浏览: 71
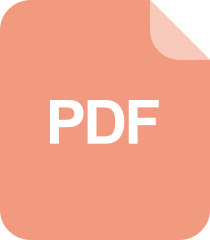
在程序中使用.ui文件
这段代码是一个 Qt 程序,用于实现一个登录界面。
```cpp
#include "mylogin.h"
```
包含一个名为 "mylogin.h" 的头文件。
```cpp
mylogin::mylogin(QWidget *parent) : QDialog(parent) {
```
定义了一个名为 mylogin 的类,并继承自 QDialog 类。在构造函数中使用了成员初始化列表,将 parent 传递给 QDialog 的构造函数。
```cpp
this->init_ui();
```
调用了 init_ui 函数,用于初始化界面。
```cpp
connect(this->bnt_login, &QPushButton::clicked, this, &mylogin::do_login);
connect(this->bnt_register, &QPushButton::clicked , this ,&mylogin::do_enroll);
```
使用 connect 函数将两个按钮的 clicked 信号连接到两个槽函数,分别是 do_login 和 do_enroll。
```cpp
mylogin::~mylogin() {
```
析构函数。没有实现具体的逻辑,因为这个类中没有需要手动释放的资源。
```cpp
void mylogin::init_ui() {
```
初始化界面的函数。
```cpp
this->setFixedSize(QSize(600,350));
```
设置窗口大小为 600x350。
```cpp
this->setWindowTitle(tr("岑超升"));
```
设置窗口标题为 “岑超升”。
```cpp
this->setWindowIcon(QIcon(":/src/1.png"));
```
设置窗口图标为 “1.png”。
```cpp
this->lb1 = new QLabel();
this->lb2 = new QLabel();
this->lb3 = new QLabel();
```
定义了三个 QLabel 对象。
```cpp
this->lb1->setFixedSize(QSize(560,200));
```
设置第一个 QLabel 的固定大小为 560x200。
```cpp
QPixmap pic;
pic.load(":/src/2.png");
//this->lb1->setPixmap(pic.scaled(this->lb1->size(), Qt::KeepAspectRatio, Qt::SmoothTransformation));
this->lb1->setPixmap(QPixmap(":/src/2.png"));
```
定义了一个 QPixmap 对象 pic,加载了 “2.png” 图片。然后将这个图片设置为第一个 QLabel 的背景图片。
```cpp
this->lb2->setText(tr("用户名:"));
this->lb3->setText(tr("密 码:"));
```
设置第二个和第三个 QLabel 的文本内容。
```cpp
this->usr_name_le = new QLineEdit();
this->usr_pass_le = new QLineEdit();
this->usr_pass_le->setEchoMode(QLineEdit::Password);
```
定义了两个 QLineEdit 对象,分别用于输入用户名和密码。将密码框的 echoMode 设置为 Password,以保证输入的内容不可见。
```cpp
this->bnt_login = new QPushButton(tr("登 陆"));
this->bnt_register = new QPushButton(tr("注 册"));
```
定义了两个 QPushButton 对象,分别用于登录和注册。
```cpp
this->hb1 = new QHBoxLayout();
this->hb2 = new QHBoxLayout();
this->hb3 = new QHBoxLayout();
this->hb1->addWidget(this->lb2);
this->hb1->addWidget(this->usr_name_le);
this->hb2->addWidget(this->lb3);
this->hb2->addWidget(this->usr_pass_le);
this->hb3->addWidget(this->bnt_login);
this->hb3->addWidget(this->bnt_register);
```
定义了三个 QHBoxLayout 对象,分别用于放置用户名、密码和登录、注册两个部分。将 QLabel 和 QLineEdit、QPushButton 对象添加到对应的 QHBoxLayout 中。
```cpp
this->vb1 = new QVBoxLayout();
this->vb1->addWidget(this->lb1);
this->vb1->addLayout(this->hb1);
this->vb1->addLayout(this->hb2);
this->vb1->addLayout(this->hb3);
this->setLayout(this->vb1);
```
定义了一个 QVBoxLayout 对象,用于放置整个界面的内容。将三个 QHBoxLayout 添加到 QVBoxLayout 中,然后将 QVBoxLayout 设置为当前窗口的布局。
```cpp
void mylogin::do_login() {
emit sig_login(usr_name_le->text(), usr_pass_le->text());
}
```
定义了一个槽函数 do_login,当用户点击登录按钮时,会发射一个 sig_login 信号,并将输入的用户名和密码作为参数传递给信号。
```cpp
void mylogin::do_enroll() {
emit sig_enroll();
}
```
定义了一个槽函数 do_enroll,当用户点击注册按钮时,会发射一个 sig_enroll 信号。
阅读全文
相关推荐
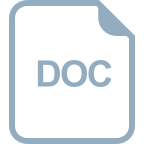
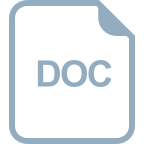













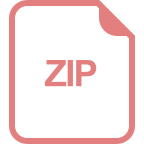
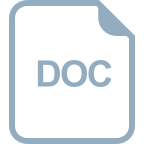
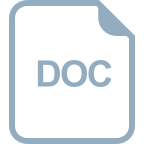