package JavaFillBlank13_2; import java.applet.*; import java.awt.*; public class JavaFillBlank13_2 extends Applet { String audioFileName, imageFileName; AudioClip clip; Image img; int x, y; public void init() { try {//******Found**** x = Integer.parseInt(getParameter("x")); //******Found**** y = Integer.parseInt(getParameter("y")); } catch (NumberFormatException ex) { } audioFileName = getParameter("audioFileName"); imageFileName = getParameter("imageFileName"); //******Found**** clip = getAudioClip(getDocumentBase(), audioFileName); //******Found**** img = getImage(getDocumentBase(), imageFileName); } public void paint(Graphics g) { //******Found**** g.drawImage(img, x, y, this); //在指定位置(x,y)处显示图片 //******Found**** clip.loop();//循环播放 } }<!DOCTYPE HTML> <HTML><HEAD></HEAD> <BODY> <APPLET CODE="JavaFillBlank13_2.class" WIDTH=800 HEIGHT=500> <param name="x" value="10"/> <param name="y" value="10"/> <param name="audioFileName" value="C:\Users\lenovo\Music\自然冥想 - 瀑布流水声 自然水声&轻音乐 大自然的声音 放松 助眠 冥想 (纯音乐).ogg"/> <param name="imageFileName" value="C:\Users\lenovo\Desktop\Java程序设计\6-1\src\JavaFillBlank13_2\zzu.jfif"/> </APPLET> </BODY> </HTML>显示不了图片为什么
时间: 2023-07-01 12:23:25 浏览: 58
这可能是因为你指定的图片文件路径有误,导致无法加载图片。请检查路径是否正确,并确保图片文件存在于指定的路径中。同时,你需要注意以下几点:
1. 在HTML代码中,参数值需要使用双引号括起来,并且路径中的反斜杠需要使用双反斜杠或正斜杠来表示。
2. 确认图片的文件格式是否被浏览器支持。常见的图片格式包括JPEG、PNG、GIF等。
3. 确认图片的大小是否超出了浏览器的显示范围。如果图片太大,可能会被截断或缩小,导致无法正常显示。
4. 在Java代码中,使用getImage()方法加载图片时,需要使用相对路径或绝对URL来指定图片位置。如果使用绝对路径,需要使用getCodeBase()或getDocumentBase()方法来获取Applet的代码库或文档库的URL。
你可以根据以上几点进行排查和调试,找出图片无法正常显示的原因。
相关问题
制作一个Applet小应用程序,设置其布局为BorderLayout,定义一个按钮和多行文本框并分别放到“North”区域和“Center”区域。 import java.awt.*; import java.applet.*; import javax.swing.*; import java.awt.event.*; //声明AppletDemo类,其父类为JApplet,并实现接口ActionListener (1 )
好的,以下是代码实现:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class AppletDemo extends JApplet implements ActionListener {
private JButton button;
private JTextArea textArea;
public void init() {
setLayout(new BorderLayout()); // 设置布局为BorderLayout
button = new JButton("Click Me!"); // 定义一个按钮
button.addActionListener(this); // 添加按钮的监听器
add(button, BorderLayout.NORTH); // 把按钮放到“North”区域
textArea = new JTextArea(); // 定义一个多行文本框
add(textArea, BorderLayout.CENTER); // 把文本框放到“Center”区域
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == button) {
textArea.append("Button clicked!\n"); // 按钮被点击时,在文本框中输出信息
}
}
}
```
在上面的代码中,我们使用了JApplet类来创建AppletDemo类,并实现了ActionListener接口来处理按钮的事件。在init()方法中,我们设置了布局为BorderLayout,并定义了一个按钮和一个多行文本框,并把它们放到了“North”和“Center”区域。在actionPerformed()方法中,我们判断事件的来源是否为按钮,并在文本框中输出一条信息。
编程题。在Applet中画一轮黄色新月,并且窗口上方显示红字“The Moon"。当在窗口另一位置单击鼠标后,在鼠标位置重新绘制月亮,并写出相应html文件。请根据注释行提示完成程序代码。 import java. awt.*; import java. awt. event.*; import java. applet. Applet; public class MoonApplet extends Applet implements MouseListener { int x,y; ∥圆外切矩形左上角坐标 /**完成小程序的初始化方法init()为x、y赋初值,并为本类注册鼠标事件监听器*/ (2分) /**完成小程序的绘图方法paint(Graphics g)在Applet上显示文字和新月图形*/ (4分) /**实现点击鼠标方法mouseClicked(MouseEvente)获取鼠标点击位置的坐标,重绘*/ (2分) public void mousePressed(MouseEvent e){} public void mouseEntered(MouseEvent e){} public void mouseExited(MouseEvent e){} public void mouseReleased(MouseEvent e){} } /**HTML文件:*/ (2分)
下面是完整的程序代码和对应的HTML文件:
MoonApplet.java
```java
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class MoonApplet extends Applet implements MouseListener {
int x, y; // 圆外切矩形左上角坐标
// 完成小程序的初始化方法init()为x、y赋初值,并为本类注册鼠标事件监听器
public void init() {
x = 50;
y = 50;
addMouseListener(this);
}
// 完成小程序的绘图方法paint(Graphics g)在Applet上显示文字和新月图形
public void paint(Graphics g) {
// 显示红字“The Moon"
g.setColor(Color.red);
g.drawString("The Moon", 10, 30);
// 画黄色新月
g.setColor(Color.yellow);
g.fillArc(x, y, 100, 100, 30, 300);
g.fillArc(x + 50, y, 100, 100, 210, 300);
}
// 实现点击鼠标方法mouseClicked(MouseEvent e)获取鼠标点击位置的坐标,重绘
public void mouseClicked(MouseEvent e) {
x = e.getX();
y = e.getY();
repaint();
}
public void mousePressed(MouseEvent e) {}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
}
```
MoonApplet.html
```html
<html>
<head>
<title>Moon Applet</title>
</head>
<body>
<applet code="MoonApplet.class" width="300" height="200"></applet>
</body>
</html>
```
说明:
1. 程序中用到了java.awt和java.awt.event两个包,需要在程序开头进行导入。
2. init()方法中设置初始的坐标值x和y,并通过addMouseListener()方法注册鼠标事件监听器。
3. paint()方法中先用g.drawString()方法显示红字“The Moon",然后用g.fillArc()方法画出黄色的新月图形。
4. mouseClicked()方法中获取鼠标点击位置的坐标,并调用repaint()方法重绘界面。
5. mousePressed()、mouseEntered()、mouseExited()、mouseReleased()这四个方法可以不进行实现,留空即可。
6. HTML文件中通过<applet>标签引用了该Applet程序,并设置了宽度和高度。
相关推荐
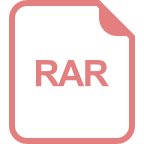
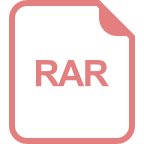
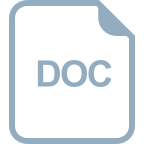












