package work; import java.applet.Applet; import java.awt.Color; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.geom.Line2D; import java.awt.geom.Point2D; public class CyrusBeckAlgorithmApplet extends Applet { private static final long serialVersionUID = 1L; private Point2D.Double[] clipWindow; private Point2D.Double[][] lines; private double[][] vectors; private double[] p1, p2, D; @Override public void init() { clipWindow = new Point2D.Double[3]; clipWindow[0] = new Point2D.Double(200, 275); clipWindow[1] = new Point2D.Double(250.0 / 3, 100); clipWindow[2] = new Point2D.Double(950.0 / 3, 100); lines = new Point2D.Double[2][2]; lines[0][0] = new Point2D.Double(0, 120); lines[0][1] = new Point2D.Double(400, 120); lines[1][0] = new Point2D.Double(0, 180); lines[1][1] = new Point2D.Double(400, 180); vectors = new double[2][2]; D = new double[2]; } @Override public void paint(Graphics g) { super.paint(g); Graphics2D g2d = (Graphics2D) g; // draw clip window g2d.setColor(Color.BLACK); g2d.draw(new Line2D.Double(clipWindow[0], clipWindow[1])); g2d.draw(new Line2D.Double(clipWindow[1], clipWindow[2])); g2d.draw(new Line2D.Double(clipWindow[2], clipWindow[0])); // draw lines for (int i = 0; i < lines.length; i++) { Point2D.Double p1 = lines[i][0]; Point2D.Double p2 = lines[i][1]; cyrusBeckClip(g2d, p1, p2); } } private void cyrusBeckClip(Graphics2D g2d, Point2D.Double p1, Point2D.Double p2) { double tE = 0, tL = 1; double dx = p2.x - p1.x; double dy = p2.y - p1.y; for (int i = 0; i < clipWindow.length; i++) { Point2D.Double P1 = clipWindow[i]; Point2D.Double P2 = clipWindow[(i + 1) % clipWindow.length]; double nx = -(P2.y - P1.y); double ny = P2.x - P1.x; double D = -nx * P1.x - ny * P1.y; double numerator = nx * p1.x + ny * p1.y + D; double denominator = -(nx * dx + ny * dy); if (denominator == 0) { if (numerator < 0) { return; } } else { double t = numerator / denominator; if (denominator < 0) { tE = Math.max(tE, t); } else { tL = Math.min(tL, t); } } } if (tE <= tL) { double x1 = p1.x + tE * dx; double y1 = p1.y + tE * dy; double x2 = p1.x + tL * dx; double y2 = p1.y + tL * dy; g2d.setColor(Color.BLUE); g2d.draw(new Line2D.Double(p1, new Point2D.Double(x1, y1))); g2d.setColor(Color.RED); g2d.draw(new Line2D.Double(new Point2D.Double(x1, y1), new Point2D.Double(x2, y2))); g2d.setColor(Color.BLUE); g2d.draw(new Line2D.Double(new Point2D.Double(x2, y2), p2)); } } } 将此代码改为 Java 应用程序运行
时间: 2023-06-24 10:03:56 浏览: 131
将代码中的 `extends Applet` 和 `public void init()` 方法删除,并将 `public void paint(Graphics g)` 方法改为 `public static void main(String[] args)` 方法,代码如下:
```java
package work;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.geom.Line2D;
import java.awt.geom.Point2D;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class CyrusBeckAlgorithmApp {
private static final Point2D.Double[] clipWindow = { new Point2D.Double(200, 275),
new Point2D.Double(250.0 / 3, 100), new Point2D.Double(950.0 / 3, 100) };
private static final Point2D.Double[][] lines = { { new Point2D.Double(0, 120), new Point2D.Double(400, 120) },
{ new Point2D.Double(0, 180), new Point2D.Double(400, 180) } };
public static void main(String[] args) {
JFrame frame = new JFrame("Cyrus Beck Algorithm");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel() {
private static final long serialVersionUID = 1L;
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
// draw clip window
g2d.setColor(Color.BLACK);
g2d.draw(new Line2D.Double(clipWindow[0], clipWindow[1]));
g2d.draw(new Line2D.Double(clipWindow[1], clipWindow[2]));
g2d.draw(new Line2D.Double(clipWindow[2], clipWindow[0]));
// draw lines
for (int i = 0; i < lines.length; i++) {
Point2D.Double p1 = lines[i][0];
Point2D.Double p2 = lines[i][1];
cyrusBeckClip(g2d, p1, p2);
}
}
};
frame.add(panel);
frame.setSize(400, 300);
frame.setVisible(true);
}
private static void cyrusBeckClip(Graphics2D g2d, Point2D.Double p1, Point2D.Double p2) {
double tE = 0, tL = 1;
double dx = p2.x - p1.x;
double dy = p2.y - p1.y;
for (int i = 0; i < clipWindow.length; i++) {
Point2D.Double P1 = clipWindow[i];
Point2D.Double P2 = clipWindow[(i + 1) % clipWindow.length];
double nx = -(P2.y - P1.y);
double ny = P2.x - P1.x;
double D = -nx * P1.x - ny * P1.y;
double numerator = nx * p1.x + ny * p1.y + D;
double denominator = -(nx * dx + ny * dy);
if (denominator == 0) {
if (numerator < 0) {
return;
}
} else {
double t = numerator / denominator;
if (denominator < 0) {
tE = Math.max(tE, t);
} else {
tL = Math.min(tL, t);
}
}
}
if (tE <= tL) {
double x1 = p1.x + tE * dx;
double y1 = p1.y + tE * dy;
double x2 = p1.x + tL * dx;
double y2 = p1.y + tL * dy;
g2d.setColor(Color.BLUE);
g2d.draw(new Line2D.Double(p1, new Point2D.Double(x1, y1)));
g2d.setColor(Color.RED);
g2d.draw(new Line2D.Double(new Point2D.Double(x1, y1), new Point2D.Double(x2, y2)));
g2d.setColor(Color.BLUE);
g2d.draw(new Line2D.Double(new Point2D.Double(x2, y2), p2));
}
}
}
```
阅读全文
相关推荐
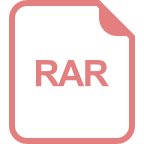
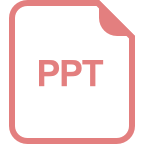
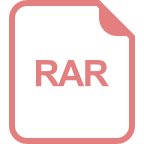









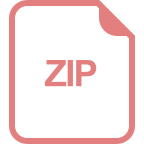
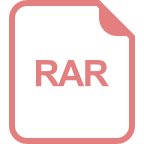
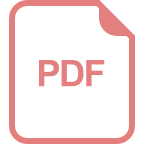
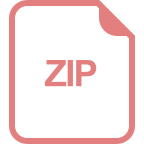
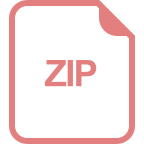
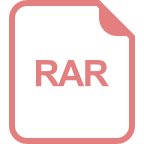
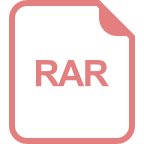