如何用neo4j和python语言构建知识图谱
时间: 2024-10-18 19:16:25 浏览: 37
在Python中使用Neo4j构建知识图谱通常涉及以下几个步骤:
1. 安装依赖:首先,你需要安装`neo4j-driver`库来连接到Neo4j数据库。你可以通过pip来安装:
```
pip install neo4j
```
2. 连接到 Neo4j:使用`neo4j`库创建连接,需要提供 Neo4j 的地址、用户名和密码:
```python
from neo4j import GraphDatabase
uri = "bolt://localhost:7687" # 更改为你实际的地址
driver = GraphDatabase.driver(uri, auth=("username", "password"))
with driver.session() as session:
# 在这里执行查询或操作
```
3. 插入数据:使用`session.run()`方法插入节点(实体)和边(关联):
```python
node_data = {"name": "Alice", "age": 30}
session.run("CREATE (:Person {properties})", properties=node_data)
relationship_data = {"from_node_id": node_data["id"], "to_node_id": another_node_data["id"], "type": "KNOWS"}
session.run("MATCH (a), (b) WHERE id(a)={from_node_id} AND id(b)={to_node_id} CREATE (a)-[:{relationship_type}]->(b)", relationship_type=relationship_data["type"])
```
4. 查询和遍历:使用Cypher查询语言来查找和分析图谱中的信息,例如找到所有Alice的朋友:
```python
query = """
MATCH (p:Person {name: 'Alice'})-[:KNOWS]->(friend)
RETURN friend.name AS friend_name
"""
results = session.run(query)
for record in results:
print(f"Alice knows {record['friend_name']}")
```
5. 构建复杂图谱:可以利用Python的循环和条件语句构建更复杂的结构,并将它们批量插入。
阅读全文
相关推荐
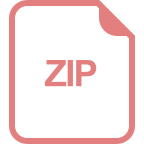
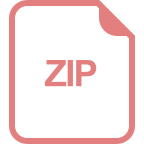
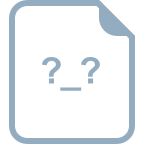
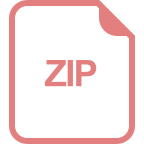

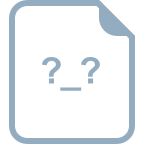
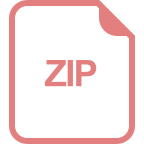
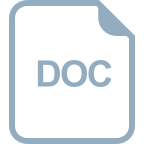
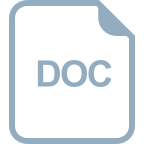
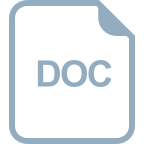
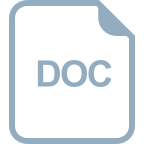
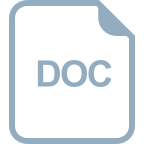
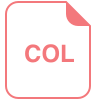




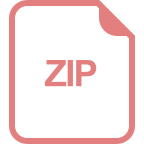
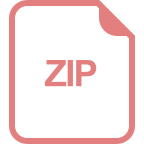