GL.FrameteTexture
时间: 2024-02-04 07:04:28 浏览: 124
我认为你可能有一个拼写错误,正确的函数应该是 `GL.FramebufferTexture`,而不是 `GL.FrameteTexture`。
`GL.FramebufferTexture` 是一个用于将纹理对象附加到帧缓冲对象的 OpenGL 函数。它允许你将一个已经创建的纹理绑定到帧缓冲对象的指定附件上,以供渲染到纹理的操作。
以下是一个示例,展示了如何使用 `FramebufferTexture` 函数将纹理附加到帧缓冲对象:
```csharp
using OpenTK;
using OpenTK.Graphics;
using OpenTK.Graphics.OpenGL4;
// 创建帧缓冲对象
int framebufferId = GL.GenFramebuffer();
GL.BindFramebuffer(FramebufferTarget.Framebuffer, framebufferId);
// 创建纹理对象
int textureId = GL.GenTexture();
GL.BindTexture(TextureTarget.Texture2D, textureId);
// 设置纹理参数
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureWrapS, (int)TextureWrapMode.ClampToEdge);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureWrapT, (int)TextureWrapMode.ClampToEdge);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureMinFilter, (int)TextureMinFilter.Linear);
GL.TexParameter(TextureTarget.Texture2D, TextureParameterName.TextureMagFilter, (int)TextureMagFilter.Linear);
// 分配空间并初始化纹理图像数据
int width = 512;
int height = 512;
GL.TexImage2D(TextureTarget.Texture2D, 0, PixelInternalFormat.Rgba, width, height, 0,
PixelFormat.Rgba, PixelType.UnsignedByte, IntPtr.Zero);
// 将纹理对象附加到帧缓冲对象的颜色附件上
GL.FramebufferTexture(FramebufferTarget.Framebuffer, FramebufferAttachment.ColorAttachment0, textureId, 0);
// 检查帧缓冲对象的完整性
FramebufferErrorCode status = GL.CheckFramebufferStatus(FramebufferTarget.Framebuffer);
if (status != FramebufferErrorCode.FramebufferComplete)
{
// 处理帧缓冲对象不完整的情况
// ...
}
```
在这个示例中,我们首先创建了一个帧缓冲对象,并绑定到当前的帧缓冲目标上。然后,我们创建了一个纹理对象,并绑定到当前的纹理单元上。
接下来,我们设置了纹理的参数,例如纹理坐标的边缘处理方式、缩小/放大过滤模式。
然后,我们使用 `glTexImage2D` 函数为纹理对象分配空间并初始化数据。这一步会将指定大小的纹理图像数据传递给 OpenGL。
最后,我们使用 `FramebufferTexture` 函数将纹理对象附加到帧缓冲对象的颜色附件上。对于颜色附件,我们使用 `FramebufferAttachment.ColorAttachment0`。
在附加完纹理之后,你可以进行后续的渲染操作,将渲染结果输出到纹理对象中。
请注意,这只是一个简单的示例,实际的应用中可能还需要考虑其他因素,例如深度缓冲区、模板缓冲区的附加,以及多个纹理对象的附加等。你可以根据自己的需求进行相应的修改和扩展。
阅读全文
相关推荐
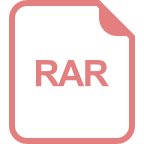
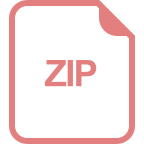
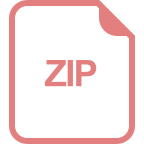
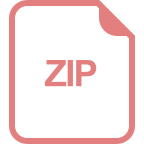
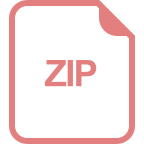
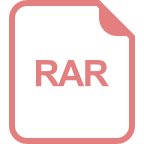
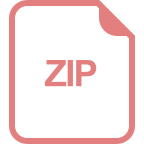
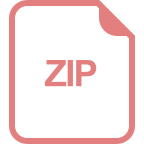
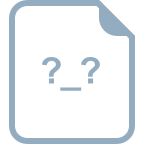
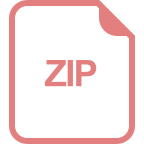
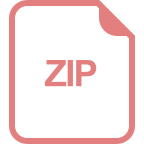
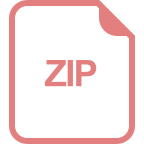
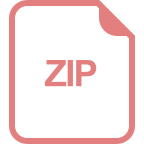